I am using Lepton 3.5 with Pure-thermal 2. Compiled C + V4l2 code. Initially got this error message Libv4l didn't accept RGB24 format. Can't proceed. Then changed image resolution from 80x60 to 160x120 and pixel format YUYV to UYVY then the code works but not generating good images. The image contains multiple strips having different colors as shown below:
*Changing pixel format to GREY and resolution 640480, generated the image shown below:**
Please anyone suggest how to get the perfect images in GREY, UYVY and other supported formats.
I am using Lepton 3.5 with Pure-thermal 2. Compiled C + V4l2 code. Initially got this error message Libv4l didn't accept RGB24 format. Can't proceed. Then changed image resolution from 80x60 to 160x120 and pixel format YUYV to UYVY then the code works but not generating good images. The image contains multiple strips having different colors as shown below:
*Changing pixel format to GREY and resolution 640480, generated the image shown below:**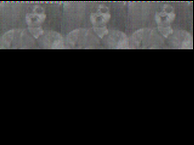
Please anyone suggest how to get the perfect images in GREY, UYVY and other supported formats.