Open xiaozhongliu opened 4 years ago
Can you try using @grpc/grpc-js
instead of the grpc
package in the client, to see if that has the same problem?
@murgatroid99 Thanks for your reply. I noticed that @grpc/grpc-js has formal version now, I've tried with it and it resulted in code 13. I've also tried with node 12 and node 14, the same results for grpc and @grpc/grpc-js packages.
I think the situations I share indicate implementation on establishing a gRPC request may be different, do you need any other clue to further investigate? Plz tell me if you need.
@xiaozhongliu Which version of gRPC-Go are you using?
And can you also try gRPC-Java? This should at least help us figure out who (Go or Core/node) is having the wrong behavior.
@menghanl Sorry for the late reply. I tested with the latest grpc-go and grpc-java. The latest summary is as below, that indicates the node client may have the incorrect behavior.
go client => gRPC service ✅
java client => gRPC service ✅
node client => gRPC service ✅
go client => gateway => gRPC service ✅
java client => gateway => gRPC service ✅
node client => gateway => gRPC service ❌
Do you have an istio enabled k8s cluster to check on this issue?
Problem description
Error occurs when calling a gRPC service which is behind the istio gateway: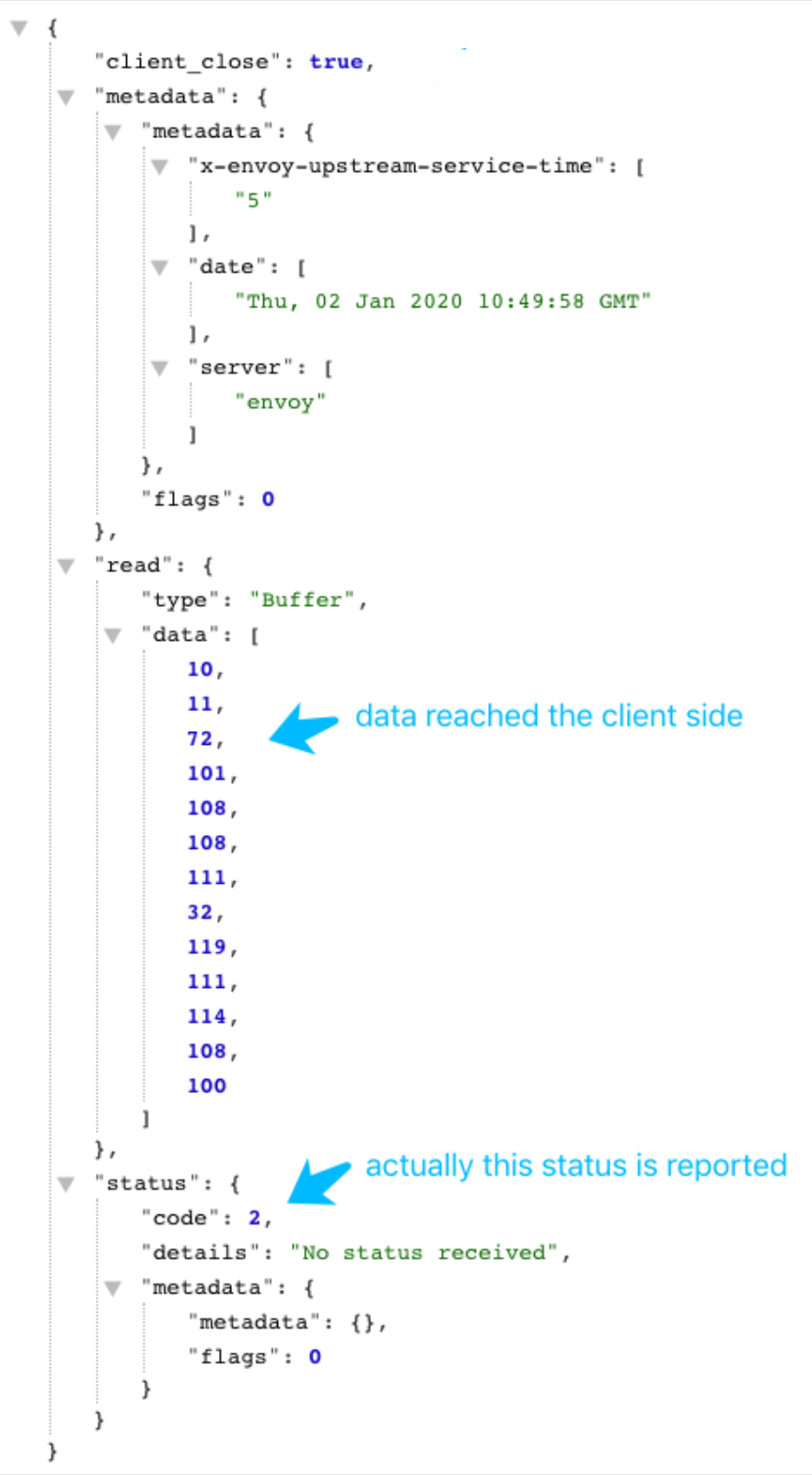
But it's ok if I use go client to request exactly the same gRPC service. Is there any difference on implementing go/node clients? Below are the results for all possible situations: go client => gRPC service ✅ node client => gRPC service ✅ go client => gateway => gRPC service ✅ node client => gateway => gRPC service ❌
Reproduction steps
server: any valid server gateway:
node client:
Environment