Closed bdr99 closed 1 year ago
I would try removing the device from BlueZ to ensure that this is not a caching issues and also log packets with Wireshark to ensure the device enumeration is actually working correctly over the air.
@dlech Thanks for the reply!
I tried removing the device from BlueZ using bluetoothctl remove CF:E6:08:09:01:02
but unfortunately that didn't help, so I don't think it's a caching issue.
I used btmon to capture the traffic and view it in WireShark, and I do see the FFF0 service in the capture. So somehow it is getting lost in between. In this test, client.services
only contained the first two services. Do you have any other ideas on what could be happening here?
Here is the WireShark capture: smart_scale_missing_service.log
It looks like the info is getting from the device to BlueZ. So, I'm guessing there is a bug in BlueZ maybe? You could try running bluetoothd
with the --debug
option to see if it gives any useful information.
Would you mind explaining how exactly to do that? When I try to run sudo bluetoothd --debug
, I get an error saying D-Bus setup failed: Name already in use
.
Also, as a side note, I tried running bluetoothctl scan le
, followed by bluetoothctl connect CF:E6:08:09:01:02
, and finally bluetoothctl info CF:E6:08:09:01:02
. This was the output:
Device CF:E6:08:09:01:02 (public)
Name: eufy T9148
Alias: eufy T9148
Paired: no
Trusted: no
Blocked: no
Connected: yes
LegacyPairing: no
UUID: Device Information (0000180a-0000-1000-8000-00805f9b34fb)
UUID: Battery Service (0000180f-0000-1000-8000-00805f9b34fb)
ManufacturerData Key: 0xff5f
ManufacturerData Value:
cf e6 08 09 01 02 cf 00 00 3a 02 00 00 00 01 01 .........:......
00 00 f7 ...
Battery Percentage: 0x5f (95)
So, the service is not showing in bluetoothctl
either. Does this help narrow down the problem?
Would you mind explaining how exactly to do that?
sudo systemctl stop bluetooth.service
sudo bluetooth --debug --nodetach
So, the service is not showing in
bluetoothctl
either. Does this help narrow down the problem?
it indicates that it is indeed a BlueZ problem, not a Bleak problem.
Well, this isn't good. I ran bluetoothd in debug mode like you explained, and it segfaulted:
bluetoothd[5518]: src/device.c:device_connect_le() Connection attempt to: CF:E6:08:09:01:02
bluetoothd[5518]: src/adapter.c:connected_callback() hci0 device CF:E6:08:09:01:02 connected eir_len 30
bluetoothd[5518]: src/device.c:att_connect_cb() connect to CF:E6:08:09:01:02: Function not implemented (38)
bluetoothd[5518]: src/adapter.c:dev_disconnected() Device CF:E6:08:09:01:02 disconnected, reason 0
bluetoothd[5518]: src/adapter.c:adapter_remove_connection()
bluetoothd[5518]: plugins/policy.c:disconnect_cb() reason 0
bluetoothd[5518]: src/adapter.c:bonding_attempt_complete() hci0 bdaddr CF:E6:08:09:01:02 type 1 status 0xe
bluetoothd[5518]: src/device.c:device_bonding_complete() bonding (nil) status 0x0e
bluetoothd[5518]: src/device.c:btd_device_set_temporary() temporary 1
bluetoothd[5518]: src/device.c:device_bonding_failed() status 14
bluetoothd[5518]: src/adapter.c:resume_discovery()
bluetoothd[5518]: src/device.c:btd_device_set_temporary() temporary 0
bluetoothd[5518]: src/device.c:device_connect_le() Connection attempt to: CF:E6:08:09:01:02
bluetoothd[5518]: src/adapter.c:connected_callback() hci0 device CF:E6:08:09:01:02 connected eir_len 30
bluetoothd[5518]: src/device.c:att_connect_cb() connect to CF:E6:08:09:01:02: Function not implemented (38)
bluetoothd[5518]: src/adapter.c:dev_disconnected() Device CF:E6:08:09:01:02 disconnected, reason 0
bluetoothd[5518]: src/adapter.c:adapter_remove_connection()
bluetoothd[5518]: plugins/policy.c:disconnect_cb() reason 0
bluetoothd[5518]: src/adapter.c:bonding_attempt_complete() hci0 bdaddr CF:E6:08:09:01:02 type 1 status 0xe
bluetoothd[5518]: src/device.c:device_bonding_complete() bonding (nil) status 0x0e
bluetoothd[5518]: src/device.c:btd_device_set_temporary() temporary 1
bluetoothd[5518]: src/device.c:device_bonding_failed() status 14
bluetoothd[5518]: src/adapter.c:resume_discovery()
bluetoothd[5518]: src/device.c:device_remove() Removing device /org/bluez/hci0/dev_6A_A3_70_EE_49_91
bluetoothd[5518]: src/device.c:btd_device_unref() Freeing device /org/bluez/hci0/dev_6A_A3_70_EE_49_91
bluetoothd[5518]: src/device.c:device_free() 0x55d2e61346a0
bluetoothd[5518]: src/device.c:btd_device_set_temporary() temporary 0
bluetoothd[5518]: src/device.c:device_connect_le() Connection attempt to: CF:E6:08:09:01:02
bluetoothd[5518]: src/adapter.c:connected_callback() hci0 device CF:E6:08:09:01:02 connected eir_len 30
bluetoothd[5518]: attrib/gattrib.c:g_attrib_ref() 0x55d2e61256d0: g_attrib_ref=1
bluetoothd[5518]: src/device.c:load_gatt_db() Restoring CF:E6:08:09:01:02 gatt database from file
bluetoothd[5518]: src/device.c:load_gatt_db() No cache for CF:E6:08:09:01:02
bluetoothd[5518]: src/gatt-client.c:btd_gatt_client_connected() Device connected.
bluetoothd[5518]: src/device.c:gatt_debug() MTU exchange complete, with MTU: 128
bluetoothd[5518]: src/device.c:gatt_debug() Primary services found: 3
bluetoothd[5518]: src/device.c:gatt_debug() start: 0x0001, end: 0x000b, uuid: 0000180a-0000-1000-8000-00805f9b34fb
bluetoothd[5518]: src/device.c:gatt_debug() start: 0x000c, end: 0x000f, uuid: 0000180f-0000-1000-8000-00805f9b34fb
bluetoothd[5518]: src/device.c:gatt_debug() start: 0x0010, end: 0x0018, uuid: 0000fff0-0000-1000-8000-00805f9b34fb
bluetoothd[5518]: src/device.c:device_remove() Removing device /org/bluez/hci0/dev_C8_4A_3D_2E_CE_65
bluetoothd[5518]: src/device.c:btd_device_unref() Freeing device /org/bluez/hci0/dev_C8_4A_3D_2E_CE_65
bluetoothd[5518]: src/device.c:device_free() 0x55d2e6137500
bluetoothd[5518]: src/device.c:gatt_debug() Secondary services found: 3
bluetoothd[5518]: src/device.c:gatt_debug() start: 0x0001, end: 0x000b, uuid: 0000180a-0000-1000-8000-00805f9b34fb
bluetoothd[5518]: src/device.c:gatt_debug() start: 0x000c, end: 0x000f, uuid: 0000180f-0000-1000-8000-00805f9b34fb
bluetoothd[5518]: src/device.c:gatt_debug() start: 0x0010, end: 0x0018, uuid: 0000fff0-0000-1000-8000-00805f9b34fb
bluetoothd[5518]: src/device.c:gatt_debug() Characteristics found: 9
bluetoothd[5518]: src/device.c:gatt_debug() start: 0x0002, end: 0x0003, value: 0x0003, props: 0x02, uuid: 00002a27-0000-1
bluetoothd[5518]: src/device.c:gatt_debug() start: 0x0004, end: 0x0005, value: 0x0005, props: 0x02, uuid: 00002a26-0000-1
bluetoothd[5518]: src/device.c:gatt_debug() start: 0x0006, end: 0x0007, value: 0x0007, props: 0x02, uuid: 00002a29-0000-1
bluetoothd[5518]: src/device.c:gatt_debug() start: 0x0008, end: 0x0009, value: 0x0009, props: 0x02, uuid: 00002a24-0000-1
bluetoothd[5518]: src/device.c:gatt_debug() start: 0x000a, end: 0x000c, value: 0x000b, props: 0x02, uuid: 00002a25-0000-1
bluetoothd[5518]: src/device.c:gatt_debug() start: 0x000d, end: 0x0010, value: 0x000e, props: 0x12, uuid: 00002a19-0000-1
bluetoothd[5518]: src/device.c:gatt_debug() start: 0x0011, end: 0x0012, value: 0x0012, props: 0x08, uuid: 0000fff1-0000-1
bluetoothd[5518]: src/device.c:gatt_debug() start: 0x0013, end: 0x0015, value: 0x0014, props: 0x10, uuid: 0000fff2-0000-1
bluetoothd[5518]: src/device.c:gatt_debug() start: 0x0016, end: 0x0018, value: 0x0017, props: 0x10, uuid: 0000fff4-0000-1
bluetoothd[5518]: src/device.c:gatt_debug() service disappeared: start 0x0001 end 0x000b
bluetoothd[5518]: src/device.c:gatt_service_removed() start: 0x0001, end: 0x000b
[1] 5516 segmentation fault sudo bluetoothd --debug --nodetach
I understand that this is not a Bleak issue, but I'd still be curious to hear if you are able to tell anything from the above log. Do you think the segfault would be the reason the service is missing from the list?
Since there are not timestamps in the log, so I can't tell when service disappeared
happened. If there was a delay and it happened on disconnect, that seems right, if not, it could be a clue.
The device does seem to be misbehaving a bit in that it lists the same services as both primary and secondary services.
The service disappeared
log happened immediately after the preceding entry, with no delay. In fact, sometimes it doesn't even happen at all, like in the below example:
bluetoothd[5978]: src/device.c:device_connect_le() Connection attempt to: CF:E6:08:09:01:02
bluetoothd[5978]: src/adapter.c:connected_callback() hci0 device CF:E6:08:09:01:02 connected eir_len 30
bluetoothd[5978]: attrib/gattrib.c:g_attrib_ref() 0x560c15e89c70: g_attrib_ref=1
bluetoothd[5978]: src/device.c:load_gatt_db() Restoring CF:E6:08:09:01:02 gatt database from file
bluetoothd[5978]: src/device.c:load_gatt_db() No cache for CF:E6:08:09:01:02
bluetoothd[5978]: src/gatt-client.c:btd_gatt_client_connected() Device connected.
bluetoothd[5978]: src/device.c:gatt_debug() MTU exchange complete, with MTU: 128
bluetoothd[5978]: src/device.c:gatt_debug() Primary services found: 3
bluetoothd[5978]: src/device.c:gatt_debug() start: 0x0001, end: 0x000b, uuid: 0000180a-0000-1000-8000-00805f9b34fb
bluetoothd[5978]: src/device.c:gatt_debug() start: 0x000c, end: 0x000f, uuid: 0000180f-0000-1000-8000-00805f9b34fb
bluetoothd[5978]: src/device.c:gatt_debug() start: 0x0010, end: 0x0018, uuid: 0000fff0-0000-1000-8000-00805f9b34fb
bluetoothd[5978]: src/device.c:gatt_debug() Secondary services found: 3
bluetoothd[5978]: src/device.c:gatt_debug() start: 0x0001, end: 0x000b, uuid: 0000180a-0000-1000-8000-00805f9b34fb
bluetoothd[5978]: src/device.c:gatt_debug() start: 0x000c, end: 0x000f, uuid: 0000180f-0000-1000-8000-00805f9b34fb
bluetoothd[5978]: src/device.c:gatt_debug() start: 0x0010, end: 0x0018, uuid: 0000fff0-0000-1000-8000-00805f9b34fb
bluetoothd[5978]: src/device.c:gatt_debug() Characteristics found: 9
bluetoothd[5978]: src/device.c:gatt_debug() start: 0x0002, end: 0x0003, value: 0x0003, props: 0x02, uuid: 00002a27-0000-1
bluetoothd[5978]: src/device.c:gatt_debug() start: 0x0004, end: 0x0005, value: 0x0005, props: 0x02, uuid: 00002a26-0000-1
bluetoothd[5978]: src/device.c:gatt_debug() start: 0x0006, end: 0x0007, value: 0x0007, props: 0x02, uuid: 00002a29-0000-1
bluetoothd[5978]: src/device.c:gatt_debug() start: 0x0008, end: 0x0009, value: 0x0009, props: 0x02, uuid: 00002a24-0000-1
bluetoothd[5978]: src/device.c:gatt_debug() start: 0x000a, end: 0x000c, value: 0x000b, props: 0x02, uuid: 00002a25-0000-1
bluetoothd[5978]: src/device.c:gatt_debug() start: 0x000d, end: 0x0010, value: 0x000e, props: 0x12, uuid: 00002a19-0000-1
bluetoothd[5978]: src/device.c:gatt_debug() start: 0x0011, end: 0x0012, value: 0x0012, props: 0x08, uuid: 0000fff1-0000-1
bluetoothd[5978]: src/device.c:gatt_debug() start: 0x0013, end: 0x0015, value: 0x0014, props: 0x10, uuid: 0000fff2-0000-1
bluetoothd[5978]: src/device.c:gatt_debug() start: 0x0016, end: 0x0018, value: 0x0017, props: 0x10, uuid: 0000fff4-0000-1
[1] 5976 segmentation fault sudo bluetoothd --debug --nodetach
FYI I opened a BlueZ issue to track this segfault problem. https://github.com/bluez/bluez/issues/438
Cool. If you install the debug symbols package for BlueZ (bluez-dbgsym
), it should give more information about where the crash is.
@dlech Good idea, but I don't see that package on my system. Are you sure that is the correct package name?
➜ ~ sudo apt install bluez-dbgsym
Reading package lists... Done
Building dependency tree... Done
Reading state information... Done
E: Unable to locate package bluez-dbgsym
I think you might have to add something in the /etc/apt/sources.list.d/
nowadays (the debug symbols packages are no longer in the main repository). https://wiki.ubuntu.com/Debug%20Symbol%20Packages
@dlech Thanks, that worked 🙂 I appreciate your help.
One last question... Is there any way to force Bleak to try to connect to a particular service/characteristic, even if it thinks it doesn't exist? That would allow me to work around this issue while I wait for a fix from BlueZ.
If the characteristic doesn't show up in BlueZ, then there is no way for Bleak to use it.
Closing this issue since the problem was fixed on the BlueZ side. https://github.com/bluez/bluez/issues/438
bluetoothctl -v
) in case of Linux: 5.64Description
I have a device (smart scale) which exposes 3 services: device information (0x180A), battery service (0x180F), and a custom vendor-defined service (0xFFF0). The issue I'm having is that Bleak can only see the first two, and the vendor-defined service doesn't show up. When I check a tool like nRF Connect on my phone, I can see all 3 services. Also and the vendor mobile app for the smart scale is able to connect to the 0xFFF0 service and receive data from the device through it.
I'd greatly appreciate any assistance in finding the reason why Bleak cannot see/connect to this service. Please let me know if there is any other info I can provide.
Here is the output when running examples/service_explorer.py:
Here is a screenshot from nRF Connect showing all 3 services: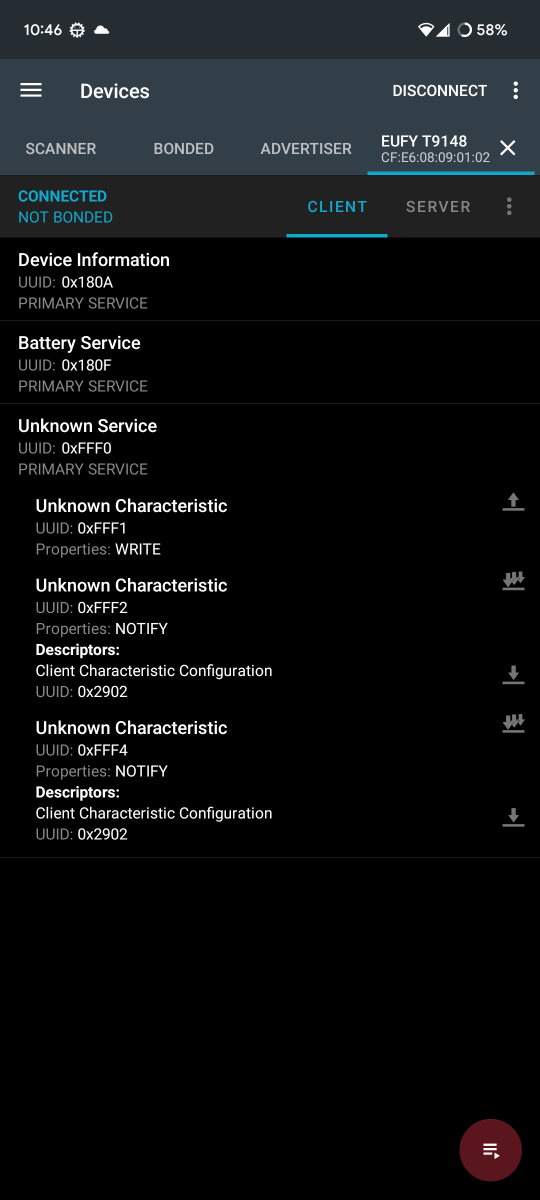
What I Did
Here is the script I wrote to try to connect to the 0xFFF0 service (which has an 0xFFF4 notify characteristic):
Here is the output I get when I run this script:
Logs
Here are the debug logs from running the above script. bleak_debug.log