Closed BelvedereHenrique closed 3 years ago
One more thing, the same happens for the Update as well. But on the update, since it utilizes the Key column, both Status and PK are wrong.
I think I've found the problem source. Column resolver has a cache using a dictionary. The problem is, the key for the dictionary is the type of the entity. Since all of my entities inherit from a base entity, the cache always stores this base type as the key, not the correct one. So, whenever a second entity comes to be inserted, the cache retrieves the column name value it stored for EntityBase on the first request.
On this screenshot, I'm trying to insert a second entity of different type, but inherited from the same base class. Since the cache stores the base class as the key, as I mentioned above, it wont work.
Is there a way to disable the cache or something like that?
Fixed.
Turned my EntityBase into a Interface, IEntityBase with properties.
(https://docs.microsoft.com/pt-br/dotnet/csharp/programming-guide/classes-and-structs/interface-properties)
Now the cache doesn't look to the interface to get the type and store it, it looks into the class instance type
Followed this documentation to understand how getType works https://docs.microsoft.com/en-us/dotnet/api/system.type.makegenerictype?view=net-5.0
Still, a option to disable cache would be nice. What you think?
Using an interface is a good way to solve this. Since .NET Core there is no reliable way to determine the 'reflected' type of a property.
Disabled caching would hurt performance a lot, you don't want that.
Hi,
I have this base entity class:
and my entities inherit from it:
Here are the mappings:
Here's the problem I'm facing.
If I create one of those, using dommel Insert, the second one won't work, because dommel (i think so) gets lost on columns mapping of the base entity. For exemple, If I insert
Avaliacao
, the columns are : PK_AVL, DESIG_AVL, STATUS_AVL. Then, right after, if I try to insertCondicaoAcesso
, which the columns are: PK_CAC, DESIG_CAC, STATUS_CAC ; Dommel fails.On the case mentioned above, dommel will try tu insert on
CondicaoAcesso
using these columns: PK_AVL, DESIG_CAC, STATUS_CACSee that PK and Status are wrong? Something is binding the first entity columns we inserted (avaliacao) on the second insert (CondicaoAcesso);
Debugging the code, I think I've found where the issue is, but I don't know how to fix it yet. It seens like Insert.cs, line 86 (
var columnNames = typeProperties.Select(p => Resolvers.Column(p, sqlBuilder)).ToArray();
) is getting the wrong columns.Here are 2 screenshots of: 1 - Inserting First Entity (avaliacao) and 2 - Trying to insert second entity (CondicaoAcesso) 1-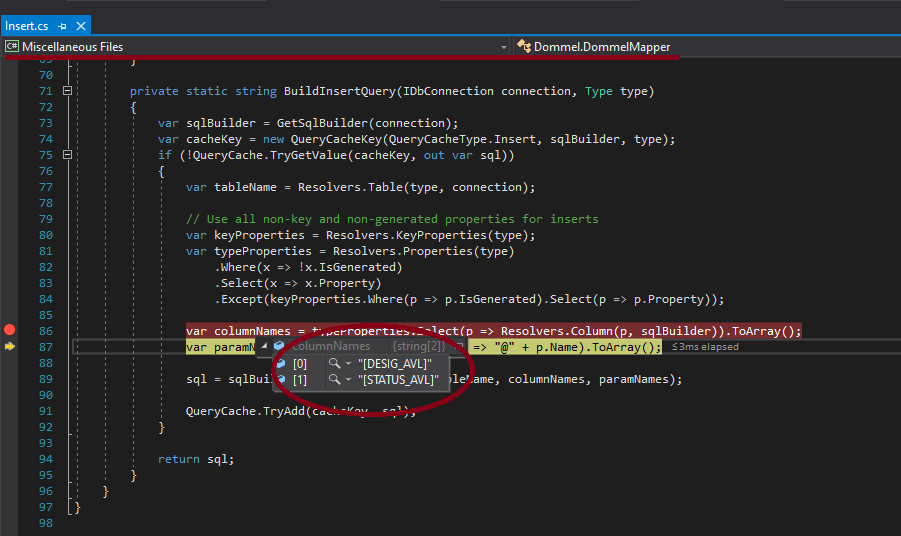
2-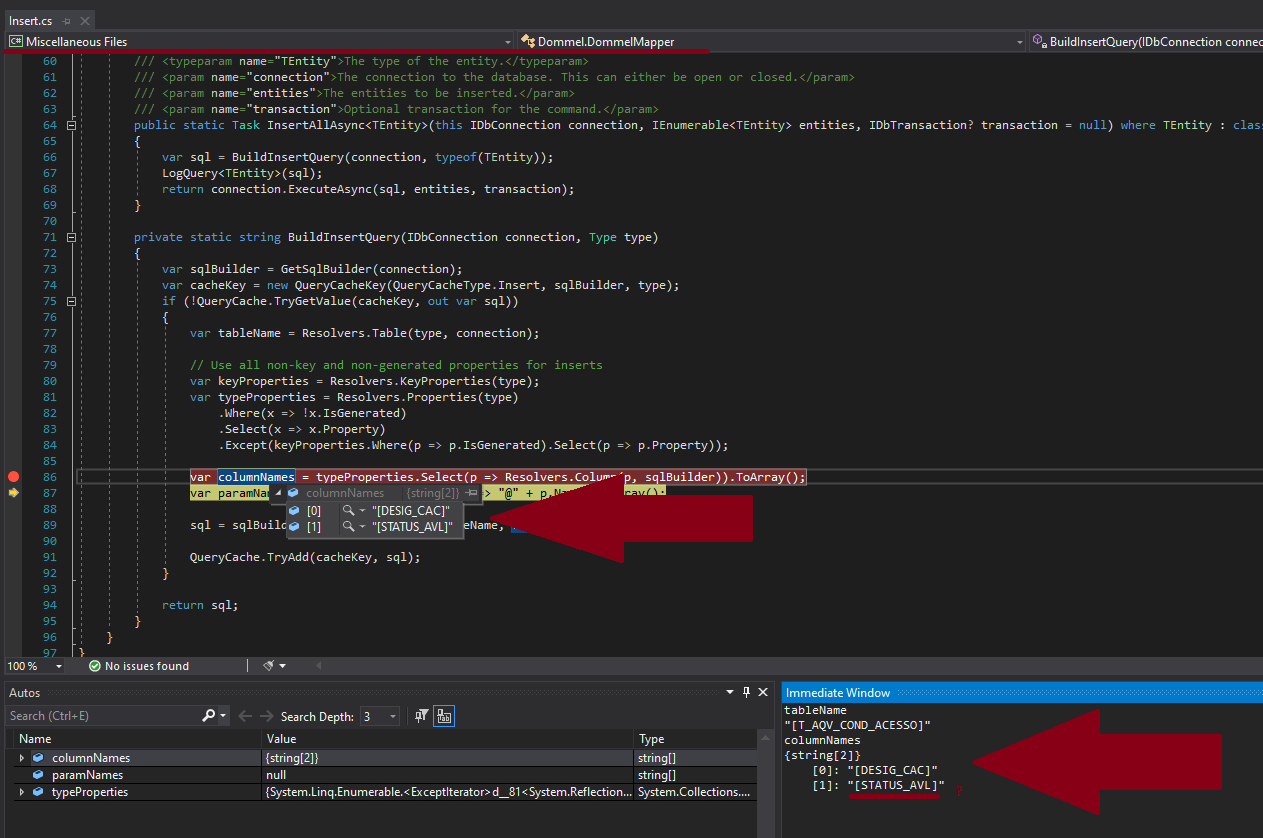
Any idea on how to fix this, or if I'm doing something wrong?
Versions: