I have two questions:
1) am I using resolver correctly to build VIPER modules
2) ~~why is it working when using UIKit (UIviewController) and not when using AsyncDisplayKit/Texture
(ASViewController<ASDisplayNode>)~~
1) Am I using resolver correctly
I have multiple module (here Root and Signin).
Each module are in there own *.xcodeproj.
Here are my files, with UIKit (working ✅):
(Public) Module Root
```swift
import UIKit
import Resolver
public class RootModule {
@LazyInjected var view: RootView
public init() {}
public func getViewController() -> UIViewController {
view
}
}
```
(Internal) RootView
```swift
import Resolver
import UIKit
final class RootView: UIViewController {
@Injected var presenter: RootPresenter
override func viewDidLoad() {
super.viewDidLoad()
}
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
presenter.viewDidAppear()
}
}
```
(Internal) RootInteractor
```swift
final class RootInteractor {
weak var presenter: RootPresenter!
}
```
(Internal) RootPresenter
```swift
import Resolver
final class RootPresenter {
@Injected var router: RootRouter
@Injected var interactor: RootInteractor
weak var view: RootView!
func viewDidAppear() {
router.showSignIn()
}
}
```
(Internal) RootRouter
```swift
import Resolver
import SignIn
final class RootRouter {
@Injected var signInModule: SignInModule
weak var presenter: RootPresenter!
func showSignIn() {
let siginInView = signInModule.getPresentation()
siginInView.modalPresentationStyle = .fullScreen
let rootView = presenter.view
rootView?.present(siginInView, animated: true, completion: nil)
}
}
```
Few questions:
1) Is the registration correct? There shouldn't be any dependency cycle nor retain cycle?
2) Who should hold the view? Is it correct if the RootModule is holding a lazy view?
3) Does the order in the registerRoot() change something?
# 2) why is it working when using UIKit and not AsyncDisplayKit
Before EDIT
Doing the following changes (and only this changes):
```diff
import Resolver
-import UIKit
+import AsyncDisplayKit
-final class RootView: UIViewController {
+final class RootView: ASViewController {
@Injected var presenter: RootPresenter
override func viewDidLoad() {
super.viewDidLoad()
}
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
presenter.viewDidAppear()
}
}
```
makes:
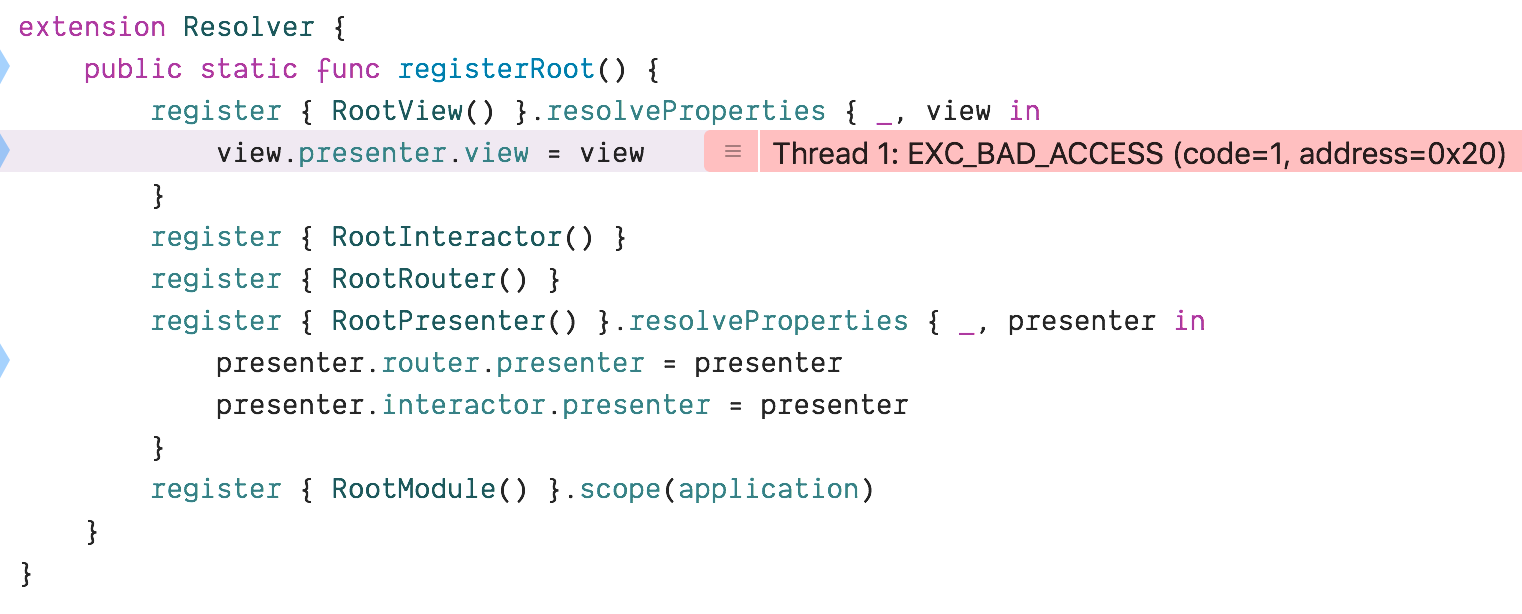
when looking at the callstack I can see:
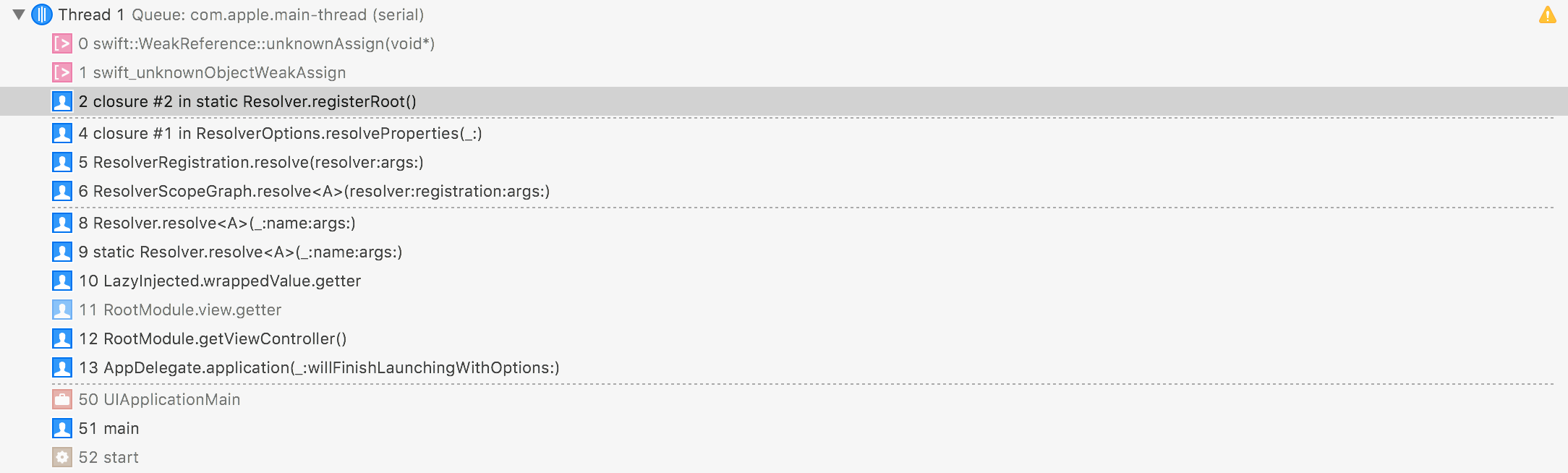
What does that means ? any idea why there is a difference between `ASViewController` and `UIViewController`?
EDIT: This solves it
import Resolver
import AsyncDisplayKit
final class RootView: ASViewController<ASDisplayNode> {
@Injected var presenter: RootPresenter
+ init() {
+ super.init(node: ASDisplayNode())
+ }
+ required init?(coder _: NSCoder) {
+ fatalError("init(coder:) has not been implemented")
+ }
override func viewDidLoad() {
super.viewDidLoad()
}
override func viewDidAppear(_ animated: Bool) {
super.viewDidAppear(animated)
presenter.viewDidAppear()
}
}
I have two questions: 1) am I using resolver correctly to build VIPER modules 2) ~~why is it working when using
UIKit
(UIviewController
) and not when usingAsyncDisplayKit
/Texture
(ASViewController<ASDisplayNode>
)~~1) Am I using resolver correctly
I have multiple module (here
Root
andSignin
). Each module are in there own*.xcodeproj
.Here are my files, with UIKit (working ✅):
(Public) Module Root
```swift import UIKit import Resolver public class RootModule { @LazyInjected var view: RootView public init() {} public func getViewController() -> UIViewController { view } } ```(Internal) RootView
```swift import Resolver import UIKit final class RootView: UIViewController { @Injected var presenter: RootPresenter override func viewDidLoad() { super.viewDidLoad() } override func viewDidAppear(_ animated: Bool) { super.viewDidAppear(animated) presenter.viewDidAppear() } } ```(Internal) RootInteractor
```swift final class RootInteractor { weak var presenter: RootPresenter! } ```(Internal) RootPresenter
```swift import Resolver final class RootPresenter { @Injected var router: RootRouter @Injected var interactor: RootInteractor weak var view: RootView! func viewDidAppear() { router.showSignIn() } } ```(Internal) RootRouter
```swift import Resolver import SignIn final class RootRouter { @Injected var signInModule: SignInModule weak var presenter: RootPresenter! func showSignIn() { let siginInView = signInModule.getPresentation() siginInView.modalPresentationStyle = .fullScreen let rootView = presenter.view rootView?.present(siginInView, animated: true, completion: nil) } } ```I'm setuping DI like this:
Few questions: 1) Is the registration correct? There shouldn't be any dependency cycle nor retain cycle? 2) Who should hold the view? Is it correct if the
RootModule
is holding a lazy view? 3) Does the order in theregisterRoot()
change something?# 2) why is it working when usingUIKit
and notAsyncDisplayKit
Before EDIT
Doing the following changes (and only this changes): ```diff import Resolver -import UIKit +import AsyncDisplayKit -final class RootView: UIViewController { +final class RootView: ASViewControllerEDIT: This solves it