Closed Nazushvel closed 2 years ago
Changing this issue as I have found this is quite larger of an issue that the original post.
The issue looks to be that two different completion menus/entries are showing up depending on how they are brought up.
This is using Nvim:7.2 with C++ using the clang completion
This issue looks to be that when first beginning to type the completion menu will show one set of options:
However if I then add a space to the end and delete it to retrigger completion I get the following:
local fn = vim.fn local install_path = fn.stdpath('data') .. '/site/pack/packer/start/packer.nvim' if fn.empty(fn.glob(install_path)) > 0 then packer_bootstrap = fn.system({ 'git', 'clone', '--depth', '1', 'https://github.com/wbthomason/packer.nvim', install_path }) vim.cmd [[packadd packer.nvim]] end return require('packer').startup(function(use) use { "wbthomason/packer.nvim" } use { "sainnhe/gruvbox-material" } use { "nvim-treesitter/nvim-treesitter" } use { "p00f/nvim-ts-rainbow" } use { "JoosepAlviste/nvim-ts-context-commentstring" } use { "tpope/vim-commentary" } use { "neovim/nvim-lspconfig" } use { "williamboman/mason-lspconfig.nvim" } use { "williamboman/mason.nvim" } use { "WhoIsSethDaniel/mason-tool-installer.nvim" } use { "michaelb/sniprun", run = "bash install.sh" } use { "kyazdani42/nvim-tree.lua" } use { "nvim-lualine/lualine.nvim" } use { "sakhnik/nvim-gdb" } use { "hrsh7th/cmp-nvim-lsp" } use { "hrsh7th/cmp-nvim-lsp-signature-help" } use { "hrsh7th/cmp-buffer" } use { "hrsh7th/cmp-path" } use { "hrsh7th/nvim-cmp" } if packer_bootstrap then require('packer').sync() end -- ColorScheme TODO add if check for tty vim.opt.termguicolors = true vim.opt.background = 'dark' vim.g.gruvbox_material_background = 'hard' vim.g.gruvbox_material_foreground = 'original' vim.cmd [[colorscheme gruvbox-material]] --vim.cmd [[set completeopt=menu,menuone,noselect]] -- Settings vim.opt.clipboard = "unnamedplus" vim.opt.number = true vim.opt.hidden = true vim.opt.cursorline = true vim.opt.relativenumber = true vim.g.mapleader = "," vim.opt.wildmenu = true vim.opt.smartindent = true vim.opt.autoindent = true vim.opt.shiftwidth = 2 -- TreeSitter require("nvim-treesitter.configs").setup { ensure_installed = "all", highlight = { enable = true, }, rainbow = { enable = true, extended_mode = true, max_file_lines = nil, }, context_commentstring = { enable = true }, } vim.cmd [[set foldmethod=expr]] vim.cmd [[set foldexpr=nvim_treesitter#foldexpr()]] -- LSP Config & Mason require('mason').setup {} require('mason-tool-installer').setup { ensure_installed = { 'clangd', 'clang-format', 'json-lsp', 'cmake-language-server', 'cmakelang' }, auto_update = true, } local on_attach = function(client, bufnr) --vim.api.nvim_buf_set_option(bufnr, 'omnifunc', 'v:lua.vim.lsp.omnifunc') local bufopts = { noremap = true, silent = true, buffer = bufnr } vim.keymap.set('n', 'K', vim.lsp.buf.hover, bufopts) vim.keymap.set('n', '<C-k>', vim.lsp.buf.signature_help, bufopts) vim.keymap.set('n', 'gr', vim.lsp.buf.references, bufopts) vim.keymap.set('n', '<space>f', vim.lsp.buf.formatting, bufopts) end local capabilities = vim.lsp.protocol.make_client_capabilities() capabilities = require('cmp_nvim_lsp').update_capabilities(capabilities) local servers = { 'clangd', 'sumneko_lua' } for _, lsp in ipairs(servers) do require('lspconfig')[lsp].setup { on_attach = on_attach, capabilities = capabilities, } end -- Nvim CMP local cmp = require('cmp') cmp.setup { mapping = cmp.mapping.preset.insert { ["<C-n>"] = cmp.mapping(function(fallback) if cmp.visible() then cmp.select_next_item() elseif has_words_before() then cmp.complete() else fallback() -- The fallback function sends a already mapped key. In this case, it's probably `<Tab>`. end end, { "i", "s" }), ["<C-p>"] = cmp.mapping(function() if cmp.visible() then cmp.select_prev_item() end end, { "i", "s" }), }, sources = cmp.config.sources { { name = 'nvim_lsp' }, { name = 'nvim_lua' }, { name = 'nvim_lsp_signature_help' }, { name = "buffer" }, { name = "path" }, }, } -- SnipRun require('sniprun').setup { display = { "Classic", "VirtualTextErr", }, } vim.api.nvim_set_keymap("v", "ff", "<Plug>SnipRun", {}) vim.api.nvim_set_keymap("n", "fc", ":SnipClose<CR>", {}) -- NvimTree vim.api.nvim_set_keymap("n", "<leader>n", ":NvimTreeToggle<CR>", {}) require("nvim-tree").setup { disable_netrw = false, view = { number = true, relativenumber = true }, renderer = { icons = { symlink_arrow = " >> ", show = { file = true, folder = true, folder_arrow = true, git = false, }, glyphs = { default = "", symlink = "", bookmark = "M", folder = { arrow_closed = ">", arrow_open = "v", default = "", open = "", empty = "", empty_open = "", symlink = "", symlink_open = "", }, }, }, } } -- Lua Line require('lualine').setup { options = { icons_enabled = false, theme = "gruvbox-material", component_separators = { left = '', right = '' }, section_separators = { left = '', right = '' }, }, sections = { lualine_a = { 'mode' }, lualine_b = { 'filename' }, lualine_c = { 'branch', 'diff' }, lualine_x = { 'location', 'progress' }, lualine_y = {}, lualine_z = {} }, tabline = { lualine_a = { { 'buffers', mode = 4, max_length = vim.o.columns } }, lualine_b = {}, lualine_c = {}, lualine_x = {}, lualine_y = {}, } } end)
This is depending on to the clangd's behavior. I can't fix it.
Changing this issue as I have found this is quite larger of an issue that the original post.
The issue looks to be that two different completion menus/entries are showing up depending on how they are brought up.
This is using Nvim:7.2 with C++ using the clang completion
This issue looks to be that when first beginning to type the completion menu will show one set of options:
However if I then add a space to the end and delete it to retrigger completion I get the following: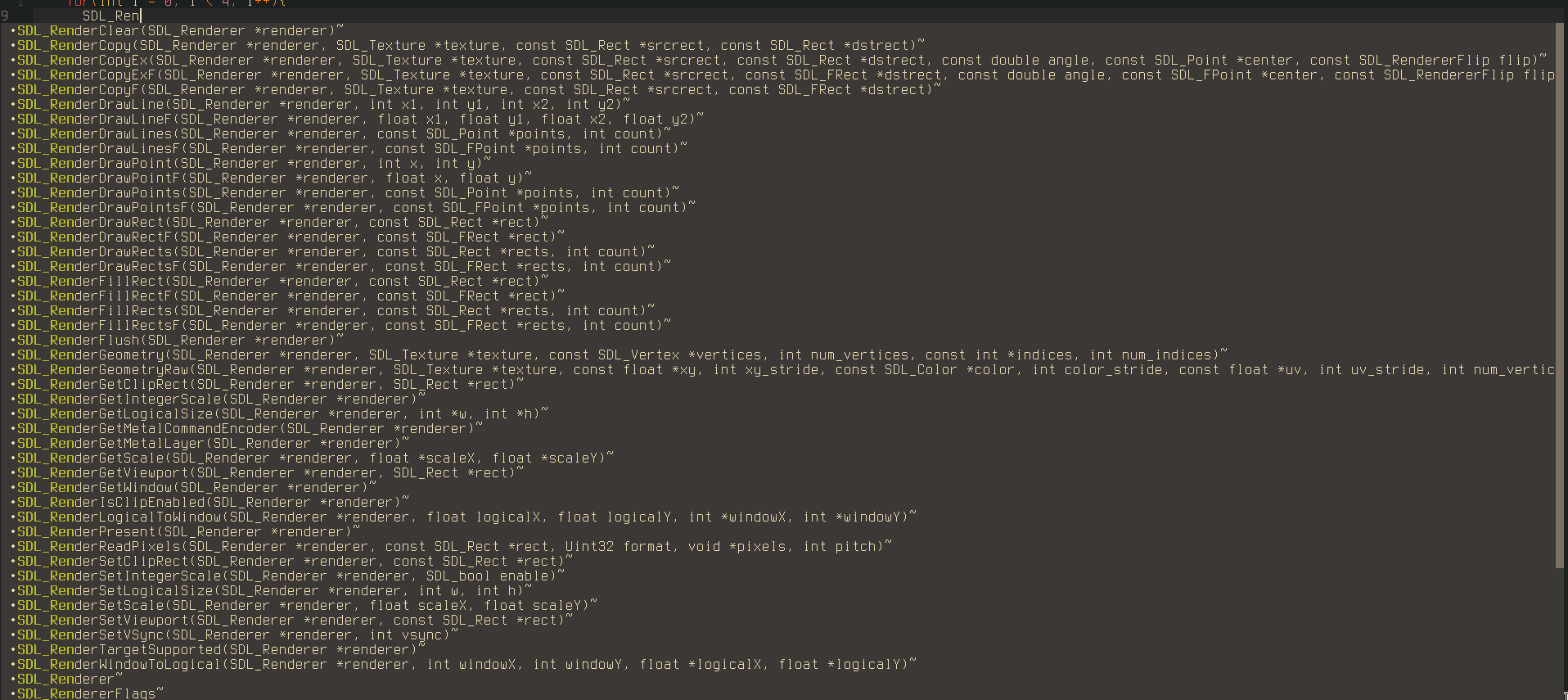