Description
I'm following the Hovering Toolbar example and when comes to this checking formatting function.
The hovering toolbar is still able to get the right matches to show the correct color of the active format.
Maybe I'm wrong somewhere or does this actually happen to all people?
Element implicitly has an 'any' type because expression of type 'string' can't be used to index type 'Node'.
No index signature with a parameter of type 'string' was found on type 'Node'.ts(7053)
Description I'm following the Hovering Toolbar example and when comes to this checking formatting function. The hovering toolbar is still able to get the right matches to show the correct color of the active format.
Maybe I'm wrong somewhere or does this actually happen to all people?
I got the following error saying
Recording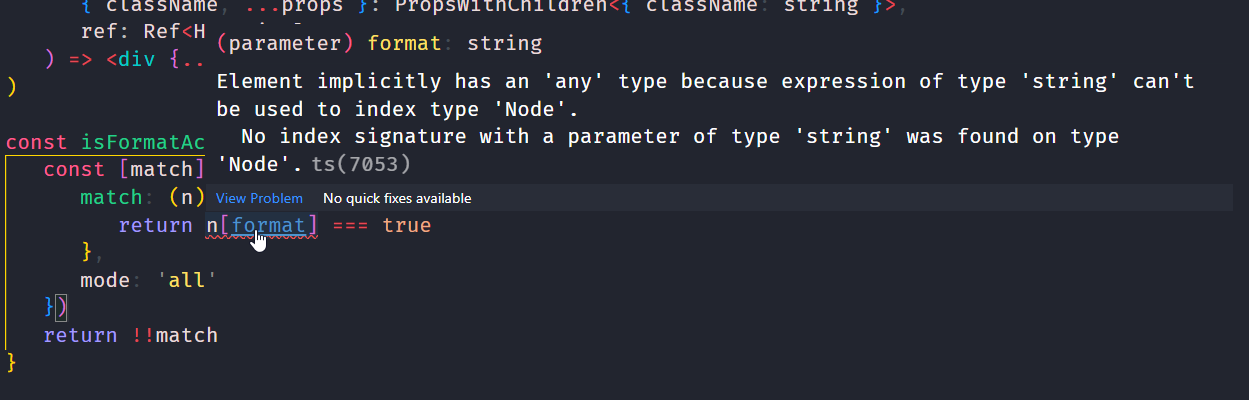
Environment
Slate Version:
Operating System: Window
TypeScript Version:
Context