Closed stidiat closed 3 years ago
Hi @stidiat did you manage to resolve the issue?
I can see that for NAD you are using an EdiElement attribute that should only contain one element - for example the NAD/1 but instead you are using it as if it was decorated as a Segment. In your case you should be using one segment class for NAD and an other to define the segment group. The group must inherit from the same class that defines the starting segment NAD in your case. Also it does not seem right to be using the SegmentGroupAttribute in your case . In order to see more examples of SegmentGroup use cases checkout other questions regarding loops and segment groups
1. One way todo this
public class ORDERS
{
public MES MES { get; set; }
}
[EdiMessage]
public class MES
{
[EdiCondition("ADE", Path = "RFF/0")]
public RFF AccountNumber { get; set; }
[EdiCondition("PD", Path = "RFF/0")]
public RFF PromotionDealNumber { get; set; }
[EdiCondition("BY", Path = "NAD/0")]
public NAD Buyer { get; set; }
[EdiCondition("SU", Path = "NAD/0")]
public NAD Supplier { get; set; }
[EdiCondition("DP", Path = "NAD/0")]
public NAD DeliveryParty { get; set; }
[EdiCondition("VA", Path = "RFF/0")]
public RFF VATRegistrationNumber { get; set; }
}
[EdiSegment, EdiPath("RFF")]
public class RFF_Element
{
[EdiValue("X(3)", Path = "RFF/0")]
public string ReferenceQualifier { get; set; }
[EdiValue("X(35)", Path = "RFF/0/1")]
public string ReferenceNumber { get; set; }
}
[EdiSegment, EdiPath("NAD")]
public class NAD
{
[EdiValue("X(3)", Path = "NAD/0")]
public string PartyQualifier { get; set; }
[EdiValue("X(35)", Path = "NAD/1")]
public string PartyIdentification { get; set; }
[EdiValue("X(3)", Path = "NAD/1/2")]
public string ResponsibleAgency { get; set; }
[EdiValue("X(3)", Path = "NAD/8")]
public string Country { get; set; }
}
2. And another way
public class ORDERS
{
public MES MES { get; set; }
}
[EdiMessage]
public class MES
{
public List<RFF> ReferenceNumbers { get; set; }
public List<NAD> Parties { get; set; }
}
[EdiSegment, EdiPath("RFF")]
public class RFF_Element
{
[EdiValue("X(3)", Path = "RFF/0")]
public string ReferenceQualifier { get; set; }
[EdiValue("X(35)", Path = "RFF/0/1")]
public string ReferenceNumber { get; set; }
}
[EdiSegment, EdiPath("NAD")]
public class NAD
{
[EdiValue("X(3)", Path = "NAD/0")]
public string PartyQualifier { get; set; }
[EdiValue("X(35)", Path = "NAD/1")]
public string PartyIdentification { get; set; }
[EdiValue("X(3)", Path = "NAD/1/2")]
public string ResponsibleAgency { get; set; }
[EdiValue("X(3)", Path = "NAD/8")]
public string Country { get; set; }
}
Hi @cleftheris , I'm very appreciate for your reply.
Yes, I managed to do it in another way.
The point is that I need to output it in the original order, this is the document:
And this is my code:
public class ORDERS
{
public MES MES { get; set; }
}
[EdiMessage]
public class MES
{
[EdiCondition("ADE", Path = "RFF/0")]
public RFF AccountNumber { get; set; }
[EdiCondition("PD", Path = "RFF/0")]
public RFF PromotionDealNumber { get; set; }
[EdiCondition("BY", Path = "NAD/0")]
public NAD Buyer { get; set; }
[EdiCondition("SU", Path = "NAD/0")]
public NAD Supplier { get; set; }
[EdiCondition("DP", Path = "NAD/0")]
public NAD DeliveryParty { get; set; }
[EdiCondition("VA", Path = "RFF/0")]
public RFF VATRegistrationNumber { get; set; }
}
[EdiSegment, EdiPath("RFF")]
public class RFF
{
[EdiValue("X(3)", Path = "RFF/0")]
public string ReferenceQualifier { get; set; }
[EdiValue("X(35)", Path = "RFF/0/1")]
public string ReferenceNumber { get; set; }
}
[EdiSegment, EdiPath("NAD")]
public class NAD
{
[EdiValue("X(3)", Path = "NAD/0")]
public string PartyQualifier { get; set; }
[EdiValue("X(35)", Path = "NAD/1")]
public string PartyIdentification { get; set; }
[EdiValue("X(3)", Path = "NAD/1/2")]
public string ResponsibleAgency { get; set; }
[EdiValue("X(3)", Path = "NAD/8")]
public string Country { get; set; }
}
I know the code is not up to standard or robust. But it can output the text in original order like below:
RFF+ADE:1234' RFF+PD:1704' NAD+BY+1234567890::9' NAD+SU+1234567890::9' NAD+DP+1234567890::9+++++++GB' RFF+VA:GB727255821'
I think I will rewrite the file in your standard way later. Because there are very complicated parts in the document(EDIFACT D96A INVOIC) like this:
Thank you again for your reply.
Best regards, Nick
Hi @cleftheris ,
I'm trying to deserialize the EDIFACT file, but the value of NAD is NULL.
This is my code of model:
And this is the result: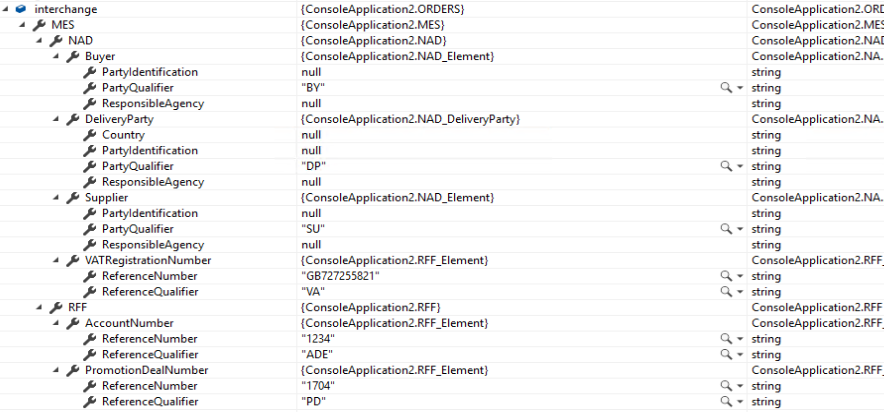
There is the EDIFACT file:
The value of RFF is correct, but the value of NAD is NULL.
And another question is, I want to serialize POCO to EDIFACT without outputting UNA segment. How can I do this?
Do you have any suggestion for me?
Thank you very much! Nick