Closed ThisOldDog closed 2 years ago
Hello @ThisOldDog - thank you for raising an issue and for the excellent description!
That is interesting! So the solution would be if these two if statements were reversed:
} else if (interop.hasMembers(value)) {
return asJavaObject(value, Map.class, null, false, languageContext);
} else if (interop.hasArrayElements(value)) {
return asJavaObject(value, List.class, null, false, languageContext);
}
Maybe wortwhile to try to patch the Graaljs version for this? https://github.com/javadelight/delight-graaljs-sandbox/blob/master/pom.xml#L58
Thank you for your reply. I temporarily covered the code to solve the problem.😂
Hi there, We tried to call custom Java functions in JS, but when the parameters were converted, we found that the array was converted to a Map. I found the relevant conversion process from the source code:
The
hasMembers
method is called before thehasArrayElements
method:As you can see,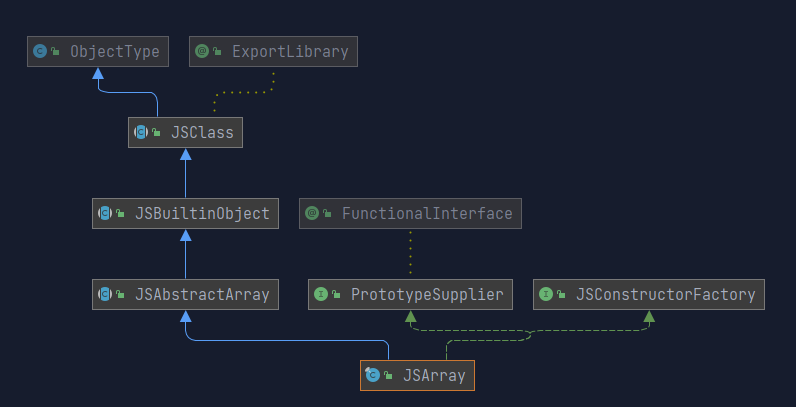
JSArray
inherits fromJSClass
:Therefore,
JSArray
will be converted into an empty Map(Although I can get the array elements byPolyglotMap.get("arrayIndex")
, but this is very strange). Do you have any ideas about why this might happen?Any help would be appreciated 😃