Closed jiriks74 closed 2 years ago
Hi, unfortunately this is limitation of the debugger in the default 'RAM breakpoints' mode - no interrupts are executed except the one used by the debugger to step the program and check if a breakpoint was reached. The Wire library uses interrupts and those are blocked when you debug the program, even if you click the continue button and let the program run.
In the loop where you find the program, the code waits for variable twi_state to be changed in interrupt service routine, which will never happen. Normally the timeout would end this wait but the micros function is also not working because it depends on timer interrupt - so the program hangs there.
The only situation when other interrupts are not blocked and the program should work is if you remove all breakpoints and let the program run. But in this situation you can only stop the program by the pause (suspend) button, so it will stop at random moment. But maybe this can still be useful to some extent.
There are two solutions:
@jdolinay Is it possible to flash the bootloader using another Uno or Arduino compatible board? I wasn't able to find any guides on it
@jdolinay
I flashed my bootloader from here, but I cannot get debugging to work. Here's my platformio.ini
:
@jdolinay
After quite a lot of trouble, messing around with my older Uno and new Uno as programmer, both old and new IDEs, linking some libraries, I managed to get flashing working in the new ide and I flashed Optiboot from the main repository. Breakpoints now work flawlessly with mode set to 2
, looks like way better than with 1
. Thanks for help.
Hi, I am glad that you made it work. I have an article about replacing the bootloader here at codeproject: https://www.codeproject.com/Articles/5162481/Creating-and-Debugging-Arduino-Programs-in-Visua-3 but you already have it running :) With flash breakpoints the debugging is much better. The downside is that the flash memory is overwritten often. This is discussed in the avr_debug.pdf in chapter "Using breakpoints in flash memory ".
Hi, I try to use your debugger with my project and it's awesome! The only problem I have is when I want to use my I2C display with the debug library. My project is in PlatformIO.
When I disable the display (by commenting out
d.begin()
andd.display()
in mainmain.cpp
everything works just fine, but when I enable it, it gets stuck on some waiting loop intwi.c
. Here's mymain.cpp
andlcd.cpp
.main.cpp
:lcd.cpp
:If I pause the program, I always end up here: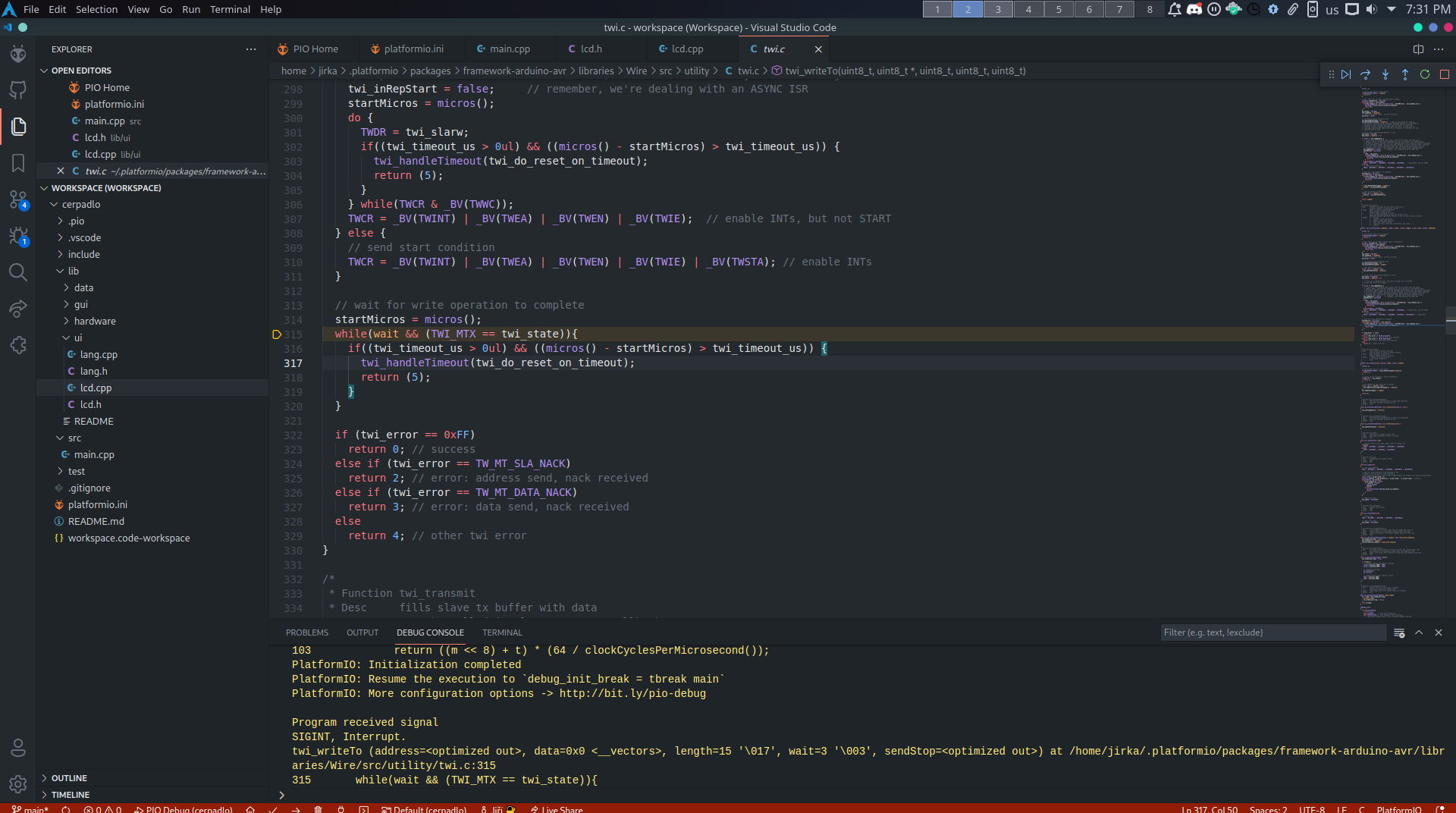
Am I doing something wrong?