Closed Bambofy closed 4 years ago
Hi, thanks for trying out vuda.
Unfortunately, I don't have a working mac OS environment at the moment - so no real repro possibilities right now. Would you mind trying a couple of things out for me.
I am currently suspecting that the Intel drivers does not satisfy the vulkan specs with respect to memory property flags.
Hi, thanks for trying out vuda.
Unfortunately, I don't have a working mac OS environment at the moment - so no real repro possibilities right now. Would you mind trying a couple of things out for me.
- run the vulkaninfoSDK.exe and report back the output.
- try to run the same code above, but where you replace the function "logical_device::malloc(void devPtr, size_t size)" with `inline void logical_device::malloc(void devPtr, size_t size) { device_buffer_node node = new device_buffer_node(size, m_allocator); (devPtr) = node->key(); push_mem_node(node); }`
I am currently suspecting that the Intel drivers does not satisfy the vulkan specs with respect to memory property flags.
Yes of course no problem, I will try your tips tomorrow. thanks for making this library it's very interesting :)
Compiling and executing with g++ gives this error:
richardbamford@MacBook-Air build % ./Program libc++abi.dylib: terminating with uncaught exception of type vk::IncompatibleDriverError: vk::createInstanceUnique: ErrorIncompatibleDriver zsh: abort ./Program
Changing malloc to the code you posted still results in the "IncompatibleDriverError"
Here is the information for a MacBook Air (Retina, 13-inch, 2019) running Intel UHD Graphics 617 1536 MB.
Hi,
I got a repro case running on mac OS. The error is related to this issue #14.
I have changed the way memory types and fallbacks are handled in the memory allocator (see the change log). On macOS, memory with HOST_CACHED is not HOST_COHERENT, while this is the case on windows for all three major GPU vendors (so the former implementation had a bug in the fallback procedure). Now the correct invalidate and flushes are in place for this memory type and you should be able to compile the samples.
Thank you.
Hi, I'm using vuda on a macbook air, but when i run cudaMalloc() i get a EXC_BAD_ACCESS error. See the image below of the error.
Code:
Error image: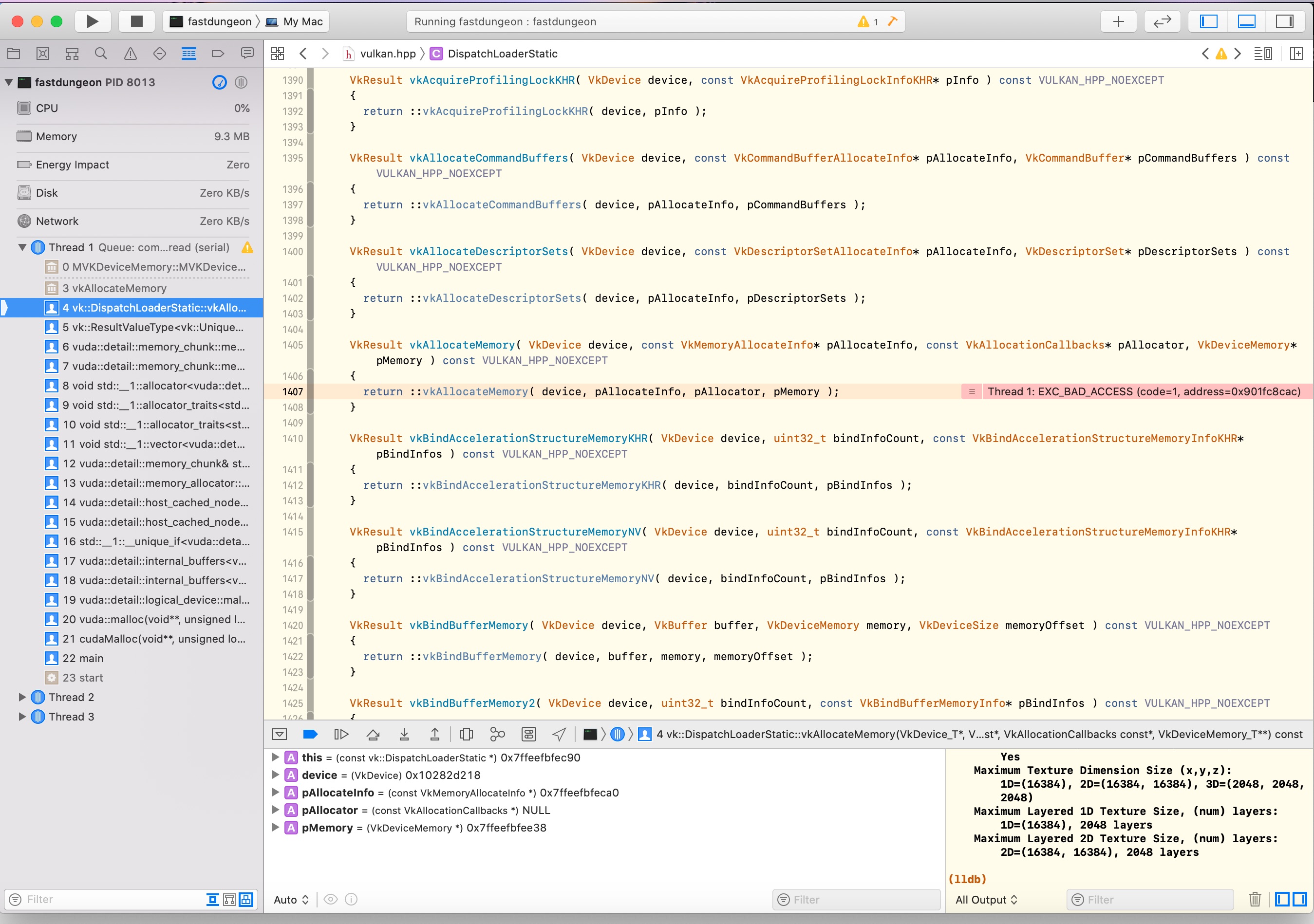
The query_device function provided in the examples reports this to console: