Closed beegee-tokyo closed 5 years ago
Will it work with only one IRQ?
The basic functions will work only with DIO1, the only thing that needs two interrupt pins is channel activity detection.
If it should work, how do I initialize the module?
You can set the unused DIO to -1 (or just some unused pin).
Does the library setup DIO2 to control the antenna?
It doesn't, DIO2 is set as IRQ during initialization. I don't have the module you're using, so I'm not entirely sure what will happen.
PS: It's pointless to check every single possible error code, SX126x can only return some of them.
Thanks for the quick reply.
The module has two pins, TX_EN and RX_EN that can be used to control the antenna. Will change my hardware and software and use these two signals instead of DIO2. Then I can use DIO2 as the second interrupt if I need it.
The channel activity detection is only for FSK mode? I am running the module in LoRa mode. So I might not need the second IRQ.
The error check was just a quick solution to see what happens. While making the function I saw already that some errors are related to other modules.
Btw. don't get the eByte module ever. The documentation is very limited and I am in contact with eByte regarding the integration in our system. But everything is "proprietary" and not available for customers. No SW examples, no clear description of the HW and they are very slow in answering questions.
Channel activity detection is essential for LoRa operation (if you have multiple transmitters in the same channel). Since LoRa is spread-spectrum modulation, the signal might be below noise floor, so CAD is the only way to check if someone is already transmitting in your channel.
Thanks for the warning :) I have a few Dorji SX126x modules, the documentation isn't the greatest, but they do have some example codes and schematics for the modules.
Kind of stuck here with this eByte module.
I control RX and TX mode over the pins on the module, one module is at the moment only sending, the other one is only receiving. Initialization is successful, I ended up with
// SX1262 module = eByte E22-900M module
SX1262 lora = new Module(5, 25, -1, 22);
// 5 = NSS, 25 = DIO1, -1 = DIO2 (not connected), 22 is BUSY
void setup()
{
...
int state = lora.begin(915.0, 125.0, 6, 5, 0x3444, 22, 8);
...
}
Setting up the SX1262 seems to be ok, no error messages from the library.
With an oscilloscope I can see as well the SCK, MOSI, MISO and NSS (CS) signals, all fine. BUSY signal is fine as well, goes up after transmit is started and then goes back to LOW. But I never get an IRQ on DIO1. It is stuck on LOW. I tested with two different MCUs, an ESP32 and a nRF52832 to make sure its not a problem with the setup or the MCU. But I get on both setups the same error.
lora.transmit("Hello");
allways fails with TX timeout.
Debug output after RADIOLIB_DEBUG was enabled:
=====================================
SX126x receiver test
=====================================
_cs: 5
_rx: 22
_tx: -1
_int0: 25
_int1: -1
80 0 AA
9D 0 A2
96 1 A2
8F 0 A2 0 A2
8A 1 A2
93 20 E2
88 3 F2 13 FA A FF 0 FF 0 FF 0 FF 0 FF
2 3 FF FF FF
8 0 FF 0 FF 0 FF 0 FF 0 FF 0 FF 0 FF 0 FF
89 7F FF
11 0 FF 0 1
8B 6 AA 4 FF 3 FF 0 FF
11 0 FF 0 1
8B 6 A2 4 A2 3 A2 0 A2
11 0 F2 0 1
8B 6 A2 4 A2 1 A2 0 A2
11 0 E2 0 1
D 7 A2 40 A2 34 A2 44 A2
11 0 E2 0 1
8C 0 A2 8 A2 0 A2 FF A2 1 A2 0 E2
98 E1 FB E9 FF
86 39 FF 30 FF 0 FF 0 FF
95 4 FF 7 FF 0 FF 1 FF
8E 16 FF 4 FF
D 8 FF E7 FF 3 FF
success!
80 0 A2
11 0 A2 0 1
Timeout in 51200 us
8 2 A2 2 A2 0 A2 2 A2 0 A2 0 A2 0 A2 0 A2
8F 0 A2 0 A2
2 3 A2 FF A2
82 0 A2 C A2 CC A2
2 3 AA FF AA
80 0 AA
11 0 A2 0 1
Timeout in 36288 us
11 0 A2 0 1
8C 0 A2 8 A2 0 A2 C A2 1 A2 0 A2
8 2 A2 1 A2 0 A2 1 A2 0 A2 0 A2 0 A2 0 A2
8F 0 E2 0 F2
E 0 FF 48 FF 65 FF 6C FF 6C FF 6F FF 20 FF 57 FF 6F FF 72 FF 6C FF 64 FF 21 FF
2 3 FF FF FF
83 0 FF 0 FF 0 FF
2 3 AA FF AA
failed: TX timeout
The
_cs: 5
_rx: 22
_tx: -1
_int0: 25
_int1: -1
was added by me to make sure the library uses the correct pins.
Code that produces above debug log is similar to the first one I posted in this issue.
Do you have any idea what could be wrong. I don't know what the RadioLib debug output means. I don't think the problem is within your library, but maybe you can help me to figure out the problem.
I think that you're using the receive methods wrong, there are two options:
You can't mix the two together.
I suggest you try the SX126x_Receive/SX126x_ReceiveInterrupt examples.
As a side note, the debug output basically all commands and responses passed between MCU and the radio. The first number is command type (MCU -> radio), then it's in pairs (first in pair is data from MCU, second is response from the radio).
Sorry for this hectic close/open/close behaviour.
Found my problem. I needed
if (lora.setTCXO(2.4) == ERR_INVALID_TCXO_VOLTAGE)
{
Serial.println(F("Selected TCXO voltage is invalid for this module!"));
}
Because the module uses TCXO over DIO3. Just didn't find anything for the voltage in the datasheet, but with 2.4 it works. I can send and receive.
Many thanks for your library!
Hi, i have a problem with the SPI timeout , but i use MCU ESP32 and Lora module E22-900M22S with SX1268, do you have an example for this module ?
@josjimenezja I have the ESP32 working with the eByte E22-900M22S module. Will look tomorrow for my source code and send you the link to an example.
@beegee-tokyo Thanks i really appreciate it, this eByte module is very complicate to used.
@josjimenezja the problem is the bad documentation of eByte. Luckily I had a customer project and could squeeze out some info from eByte because my customer promised large orders to eByte. So I could put some pressure on eByte to get more details.
@beegee-tokyo Of course, the documentation is really bad, and there are no examples or bases to use the module. I appreciate your help.
@josjimenezja Here is a code example that works on my ESP32 and eByte module. It is based on the SX126x_Receive.ino of this library with extensions to work with the eByte E22 module. Some comments:
enableRX()
and enableTX()
. You MUST call them before you start receiving or transmitting.eByte E22 module uses DIO3 to enable/disable the chips oscillator. Use lora.setTCXO(2.4)
to tell RadioLib to use DIO3 to supply the SX126x oscillator.
/*
RadioLib SX126x Receive Example
This example listens for LoRa transmissions using SX126x Lora modules.
To successfully receive data, the following settings have to be the same
on both transmitter and receiver:
- carrier frequency
- bandwidth
- spreading factor
- coding rate
- sync word
- preamble length
Other modules from SX126x family can also be used.
For full API reference, see the GitHub Pages
https://jgromes.github.io/RadioLib/
*/
// include the library
// Forward declaration of functions void enableRX(void); void enableTX(void);
// GPIOs to control E22 RX_EN and TX_EN
// SX1262 module = eByte E22-900M module SX1262 lora = new Module(5, 21, 21, 22); // 5 = NSS, 21 = DIO1, 21 = DIO2 (not connected), 22 is BUSY
void setup() { Serial.begin(115200);
SPI.begin(18, 19, 23, 5); // SCLK GPIO 18, MISO GPIO 19, MOSI GPIO 23, CS == NSS GPIO 5
Serial.print(F("[SX1262] Initializing ... "));
// initialize SX1262 // carrier frequency: 868.0 MHz // bandwidth: 125.0 kHz // spreading factor: 7 // coding rate: 5 // sync word: 0x1424 (private network) // output power: 22 dBm // current limit: 60 mA // preamble length: 8 symbols // CRC: enabled int state = lora.begin(868.0, 125.0, 7, 5, 0x1424, 22, 8);
if (state == ERR_NONE) { Serial.println(F("success!")); } else { Serial.print(F("failed, code ")); Serial.println(state); while (true); }
// eByte E22-900M22S uses DIO3 to supply the external TCXO if (lora.setTCXO(2.4) == ERR_INVALID_TCXO_VOLTAGE) { Serial.println(F("Selected TCXO voltage is invalid for this module!")); }
// Important! To enable receive you need to switch the SX126x antenna switch to RECEIVE enableRX();
// Important! To enable transmit you need to switch the SX126x antenna switch to TRANSMIT // enableTC(); }
void loop() { Serial.print(F("[SX1262] Waiting for incoming transmission ... "));
// you can receive data as an Arduino String // NOTE: receive() is a blocking method! // See example ReceiveInterrupt for details // on non-blocking reception method. String str; int state = lora.receive(str);
// you can also receive data as byte array / byte byteArr[8]; int state = lora.receive(byteArr, 8); /
if (state == ERR_NONE) { // packet was successfully received Serial.println(F("success!"));
// print the data of the packet
Serial.print(F("[SX1262] Data:\t\t"));
Serial.println(str);
// print the RSSI (Received Signal Strength Indicator)
// of the last received packet
Serial.print(F("[SX1262] RSSI:\t\t"));
Serial.print(lora.getRSSI());
Serial.println(F(" dBm"));
// print the SNR (Signal-to-Noise Ratio)
// of the last received packet
Serial.print(F("[SX1262] SNR:\t\t"));
Serial.print(lora.getSNR());
Serial.println(F(" dBm"));
} else if (state == ERR_RX_TIMEOUT) { // timeout occurred while waiting for a packet Serial.println(F("timeout!"));
} else if (state == ERR_CRC_MISMATCH) { // packet was received, but is malformed Serial.println(F("CRC error!"));
} else { // some other error occurred Serial.print(F("failed, code ")); Serial.println(state);
} }
// Set RX pin HIGH and TX pin LOW to switch to RECEIVE void enableRX(void) { digitalWrite(RADIO_RXEN, HIGH); digitalWrite(RADIO_TXEN, LOW); delay(100); }
// Set TX pin HIGH and RX pin LOW to switch to TRANSMIT void enableTX(void) { digitalWrite(RADIO_RXEN, LOW); digitalWrite(RADIO_TXEN, HIGH); delay(100); }
Here is my HW setup between ESP32 and eByte module:
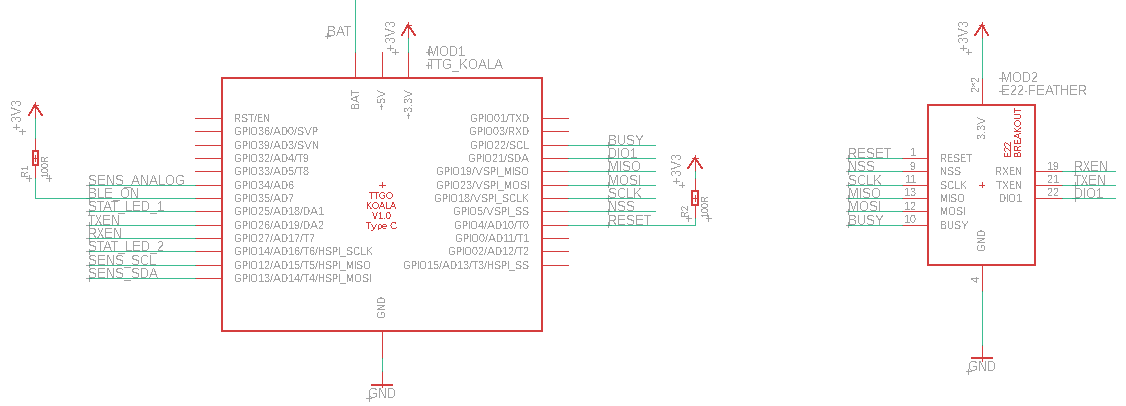
@beegee-tokyo thanks my friend.
@beegee-tokyo I have the 705 error code, in the typedef.h the description is "timed out while waiting for complete SPI command." do you now something about this error code? could be a hardware error?
Example code run perfect on my setup this morning, so it could be a hardware problem. Check all SPI lines and make sure that
SPI.begin(18, 19, 23, 5);
// SCLK GPIO 18, MISO GPIO 19, MOSI GPIO 23, CS == NSS GPIO 5
is setting the correct pins. Don't cross connect MISO and MOSI. It should be ESP -> E22 as MOSI -> MOSI and MISO -> MISO. Make sure the used GPIOs are not used otherwise. On my cheap Chinese ESP32 board they connected the on board LED on GPIO5 which is the default CS of the SPI. I had to remove the LED, to get SPI working well.
How did you connect the Reset input of the eByte module? Either connect it to the Reset of the ESP or spend another GPIO and reset the E22 by software.
ohh okay, I will check the hardware to rule out such failures.
How did you connect the Reset input of the eByte module? Either connect it to the Reset of the ESP or spend another GPIO and reset the E22 by software. If you connect RESET of E22 to RESET of ESP32 do a HW reset (push reset button of ESP32 or power off/on cycle of both modules) to make sure the E22 module starts up correct.
The reset input of the eByte module is connected to a GPIO 12 in the ESP32
So you do a
pinMode(12,OUTPUT_PULLUP);
digitalWrite(12, LOW);
delay(100);
digitalWrite(12,HIGH);
Before initializing the module?
Not, that's very important, when i write this code before initializing the module , the initialization is successful and begin to receive. Now i think that i have to do some HW changes in the receptor and the transmitter.
If you have RESET of the E22 connected to a GPIO on the ESP you must initialize the GPIO as output and perform a LOW - HIGH cycle, otherwise your E22 is in an undefined state.
Glad my example works for you now. Good luck.
@beegee-tokyo it's working , i really appreciate your help. thank you very much.
@beegee-tokyo hi, i have one more question, how far can you transmit? because i can some many meters, like 20 or 25.
Still testing antennas and Lora settings, but my best was ~400m. I can only test in an area with many buildings and obstacles (metal structures), so it's not the best condition for LoRa. In addition my base station antenna is surrounded on 3 sides by higher buildings. I am testing antennas from Airgain and eByte and some unknown Chinese suppliers. The best so far are the Airgain antennas.
i have the same problem with the place, but now i try to find the best value of the lora.beging () parametters. With this values "lora.begin(915.0, 500.0, 12, 8, 0x1424, 18, 8)" and the nodes very closer(50cm), my RSSI = -63 dBm and SNR= 4 dBm (indoor).
RSSI of - 63 is not very good. Try to lower your bandwidth (500MHz is outside of regulation in most countries anyway) I use 125MHz only.
Are you sure your E22 module has a SX1268? All modules I bought from eByte have a SX1262 chip. Try to use SX1262 lora = new Module(....
instead of SX1268.
Try the settings in my example code, only change frequency from 868.0 to 915.0 if that is the frequency for LoRa in your country
i try with sx1262 and your settings. The RSSI = -71 dBm and SNR= 12.25 dBm the distance between the nodes is like 50 cm. I don't know why i have this RSSI
What antennas are you using? Are you sure RXEN and TXEN GPIOs are configured as output and set correct before sending/receiving?
I had similar signal problem with another module (ISP4520 from Insight SIP). I thought it uses a SX1262, but instead it was a SX1261. On that specific module changing the code from SX1262 to SX1261 solved the weak signal problem. Try different SX12x versions and see if anything changes.
Unfortunately the Semtech SX126x series has no function to read out what chip type it is.
I try with all the SX126X versions and the better was SX1262, the best RSSI was -58 dBm. The RXEN and TEXN GPIOs are well configured, but I did tests with activating and deactivating them and the result is the same. I'm confused. This is the antenna model that i used. Specification Center Frequency: 868 to 915MHz Polarization: Vertical Polarization Gain: 5.0dBi V.S.W.R: <=1.5 impendance: 50 ohm Radome Material: ABS Connector Material: Copper operation Temperature: -40 to +85 Celsius Length:200mm Relative Humidity: Up to 95%
Antenna specs look good. I expected that using SX1262 is the best, because that is the chip eByte is using.
You are using the same settings on both E22 modules, sender and receiver? Is your antenna connection good? The Ipex/U.FL connector from the antenna cable is plugged in complete?
I have no other ideas right now regarding the weak signal. As I said, best result I got was ~400m with many buildings between the two LoRa nodes. When both devices are on my table I see usually a RSSI of - 20dBm or better. I am testing with the settings I wrote before on all devices and I have a mixed setup of ESP32 with eByte module (my gateway) and up to 6 sensor nodes with ISP4520 (nRF52832 + SX1261).
Now i can trasmit with RSSI = 0 when the antennas are together, when are like 20 metres indoor the RSSI = -35 - -45. I change the current limit of the trasmitter of 60mA to 100mA because the datasheet said that the necesarie current fot trasmiter is 100mA. this is the function "begin(float freq, float bw, uint8_t sf, uint8_t cr, uint16_t syncWord, int8_t power, float currentLimit, uint16_t preambleLength)" I have one more question, are you using a gateway? how i can send information of my node to my gateway? The gateway is connected to TTN and have the same frequency as the node.(915MHz)
I nether changed the transmit power while using this library but to be honest I am not using RadioLib anymore. I converted Semtech's SX126x library to Arduino because the Semtech library has LoRaWan support. But the setup is not as easy as RadioLib. If you want to try, you can find it in my repos here on GitHub. Yesterday I was able to reach 730m distance between two LoRa nodes in open field (no buildings between the nodes, only some trees). The 730m was the distance I could walk, then I was at the end of the open area. Communication was still stable at 730m,but I do not know the RSSI levels, because my gateway has no display (yet).
The ESP32 + SX1262 (eByte E22) is my gateway from LoRa to WiFi. My sensor nodes are Nordic nRF52832 + SX1261 (in one module, Insight SIP ISP4520). I could test 6 nodes connected to the gateway, but I think 10 is possible as well, just don't have enough nodes yet. I am not using LoRaWan (yet), the LoRaWan gateways are quite expensive (starting from 200US$ up}. Instead I wrote my own communication layer to handle the data flow between the nodes and the gateway.
Oh okay. I try to connect to loraWAN with radiolib but I can't, what is the name of your library, what is the link? I still do not do tests in the open field, but with the change I commented, I think I have good reach.
I feel shy to promote my library here on another fellow open source developers library. @jgromes Jan, no bad intensions from my side.
The link is SX126x-Arduino. But no promises that it works, because there is no public LoRaWan gateway available here (in the Philippines) so the only thing I could test is that my lib is trying to connect. LoRaWan gateways are quite expensive so I decided to not spend money on it, as I don't really need it.
Thanks for your help friends, i going to try to connected to my gateway and I write to you if I have any problem or if I can do it.
That would be great. You need to look into the file Commissioning.h to setup some stuff. If you run into problems, please open an issue on my repo, I don't want to hijack Jan's issues for my stuff.
@beegee-tokyo no worries
@josjimenezja I'm glad you were able to get help here, that being said, I would appreciate if you could take the discussion somewhere else. It's been off-topic for a while now. Thanks
@jgromes thanks for your understanding. I am still using your library to connect a SX127x chip to my SX126x network. Your library is supporting way more chips than I can dream off.
@josjimenezja let's continue this on my repos issue.
I have a Dorji SX1262 (868) I can't make it work in RX mode. The TX is working "SX126x_Transmit.ino" using "lora.setTCXO(1.6);"
ESP32 with Dorji SX1262 (DIO2 not connected) My RX code (not working):
#include <Arduino.h>
#include <WiFi.h>
#include <RadioLib.h>
time_t updateTime;
// Flag for sending finished
bool sendDone = false;
SPIClass *loraSPI = new SPIClass(HSPI);
// NSS DIO1 DIO2 BUSY
SX1262 lora = new Module(15, 22, -1, 21, *loraSPI);
int PIN_LORA_RESET = 19;
#define ssid = "MyWiFi"
#define pass = "qwerty123"
WiFiClient client;
struct tm timeinfo;
uint8_t dataMsg[6];
char ackMsg[] = "ACK";
long timeSinceLastPacket = 0;
uint16_t nodeId = 0;
char timeStamp[32];
void setup()
{
pinMode(PIN_LORA_RESET, OUTPUT);
digitalWrite(PIN_LORA_RESET, LOW);
delay(100);
digitalWrite(PIN_LORA_RESET, HIGH);
Serial.begin(115200);
// initialize SX1262
// carrier frequency: 868.0 MHz
// bandwidth: 125.0 kHz
// spreading factor: 7
// coding rate: 5
// sync word: 0x1424 (private network)
// output power: 22 dBm
// current limit: 60 mA
// preamble length: 8 symbols
// CRC: enabled
// Initialize LoRa
Serial.println(F("[SX1262] Initializing ... "));
int state = lora.begin(868.0, 125.0, 7); //SF7
if (state == ERR_NONE) {
Serial.println(F("LORA init success!"));
} else {
Serial.print(F("init failed, code "));Serial.println(state);
}
if (lora.setTCXO(1.6) == ERR_INVALID_TCXO_VOLTAGE)
{
Serial.println(F("Selected TCXO voltage is invalid for this module!"));
}
}
void loop() {
Serial.print(F("[SX1262] Waiting for incoming transmission ... "));
String str;
int state = lora.receive(str);
if (state == ERR_NONE) {
// packet was successfully received
Serial.println(F("success!"));
// print the data of the packet
Serial.print(F("[SX1262] Data:\t\t")); Serial.println(str);
// print the RSSI (Received Signal Strength Indicator)
// of the last received packet
Serial.print(F("[SX1262] RSSI:\t\t")); Serial.print(lora.getRSSI()); Serial.println(F(" dBm"));
// print the SNR (Signal-to-Noise Ratio)
// of the last received packet
Serial.print(F("[SX1262] SNR:\t\t")); Serial.print(lora.getSNR()); Serial.println(F(" dB"));
} else if (state == ERR_RX_TIMEOUT) {
// timeout occurred while waiting for a packet
Serial.println(F("timeout!"));
} else if (state == ERR_CRC_MISMATCH) {
// packet was received, but is malformed
Serial.println(F("CRC error!"));
} else {
// some other error occurred
Serial.print(F("failed, code ")); Serial.println(state);
}
}
With this code I get:
[SX1262] Waiting for incoming transmission ... timeout!
[SX1262] Waiting for incoming transmission ... timeout!
[SX1262] Waiting for incoming transmission ... timeout!
[SX1262] Waiting for incoming transmission ... timeout!
...
By the way I have another device transmitting over 868Mhz SF7 "helloworld" (tested and working)
Any Idea how to fix it?
This question is off-topic in this issue - please open a new issue, and provide minimum sketch required to reproduce the issue, as well as links to any hardware you are using. Bonus points if you use the bug report issue template ;)
@johnnytolengo The Dorji SX1262 module is using DIO2 to control the antenna switch. http://www.dorji.com/docs/data/DRF1262T.pdf -> table 1
You need to add in setup()
lora.setDio2AsRfSwitch(true);
and it should work. Don't connect DIO2 of the Dorji module to anything external.
I know this is old, and SX126x no longer uses DIO2, but I read this issue when I was trying to understand how to use RadioLib and used the advice in it.
This statement:
You can set the unused DIO to -1 (or just some unused pin).
is very, very, wrong. Module::init
will pass -1 to pinMode
, which interprets it as 255. It then goes and reads a 30 byte array with offset 255 (no bounds checking), find a random address, and changes it.
Much of time time, the random address will contain zero, and nothing will actually change (because of a very basic check in pinMode). Then you can change a completely different part of your program, suddenly that memory doesn't contain zero, and your program crashes in a third, unrelated part because the stack got corrupted. That's alot of fun to track down.
(This is for the Arduino core. Not sure how others behave).
That's a very good point, thanks! I've seen -1 being used as "do not use this pin" value in other libraries (Adafruit comes to mind), so I assumed it's a value that's checked in Arduino core. But you're right, the signature is pinMode(uint8_t, uint8_t)
- obviously -1 will mess things up.
I added a quick check to make sure -1 isn't passed to pinMode in 67c6544d28d8252f1944c84434f22c7c3e5e5209.
I know it's a zombie thread, but I've set the SX1268 to 1.8V for the TCXO, and it's worked fine for me. I'm using an E22 400M30S.
I also know it's old thread.The eByte documentation for the module (E22-900M30S) is actually not so bad. The only thing I was missing is the TCXO voltage. But there is another E22 Series User Manual where the TCXO voltage is mentioned - 1.8V.
@faydr Hello, I know it's been a while, but im currently trying to use an E22 400M30S. You said that you make it worked. If you could send me your code and your HW set up, it would help me a lot <3.
I use an ESP8266/E22400M30S That's my transmit code : /* RadioLib SX126x Receive Example
This example listens for LoRa transmissions using SX126x Lora modules. To successfully receive data, the following settings have to be the same on both transmitter and receiver:
preamble length
Other modules from SX126x family can also be used.
For full API reference, see the GitHub Pages https://jgromes.github.io/RadioLib/ */
// include the library
// Forward declaration of functions
void enableTX(void);
// GPIOs to control E22 RX_EN and TX_EN
// SX1262 module = eByte E22-900M module SX1268 radio = new Module(15, 4, 4, 5); // 5 = NSS, 4 = DIO1 4 = DIO2 (not connected), 5 is BUSY
void setup() { //pinMode(1,FUNCTION_3); //pinMode(3,FUNCTION_3); Serial.begin(115200);
SPI.begin(); // SCLK GPIO 18, MISO GPIO 19, MOSI GPIO 23, CS == NSS GPIO 5
Serial.print(F("[SX1262] Initializing ... "));
// initialize SX1262 // carrier frequency: 868.0 MHz // bandwidth: 125.0 kHz // spreading factor: 7 // coding rate: 5 // sync word: 0x1424 (private network) // output power: 22 dBm // current limit: 60 mA // preamble length: 8 symbols // CRC: enabled int state = radio.begin(434.0, 125.0, 9, 7, 0x34, 10, 8, 1.6, false);
if (state == RADIOLIB_ERR_NONE) { Serial.println(F("success!")); } else { Serial.print(F("failed, code ")); Serial.println(state); while (true); }
// eByte E22-900M22S uses DIO3 to supply the external TCXO if (radio.setTCXO(1.8) == RADIOLIB_ERR_INVALID_TCXO_VOLTAGE) { Serial.println(F("Selected TCXO voltage is invalid for this module!")); }
//Important! To enable transmit you need to switch the SX126x antenna switch to TRANSMIT enableTX(); }
void loop() { //TRANSMIT Serial.print(F("[SX1262] Transmitting packet ... "));
// you can transmit C-string or Arduino string up to // 256 characters long // NOTE: transmit() is a blocking method! // See example SX126x_Transmit_Interrupt for details // on non-blocking transmission method. String str = "Hello World!"; int state = radio.transmit(str);
// you can also transmit byte array up to 256 bytes long / byte byteArr[] = {0x01, 0x23, 0x45, 0x56, 0x78, 0xAB, 0xCD, 0xEF}; int state = radio.transmit(byteArr, 8); / if (state == RADIOLIB_ERR_NONE) { // the packet was successfully transmitted Serial.println(F("success!/t/t")); Serial.println(str); // print measured data rate Serial.print(F("[SX1262] Datarate:\t")); Serial.print(radio.getDataRate()); Serial.println(F(" bps"));
} else if (state == RADIOLIB_ERR_PACKET_TOO_LONG) { // the supplied packet was longer than 256 bytes Serial.println(F("too long!"));
} else if (state == RADIOLIB_ERR_TX_TIMEOUT) { // timeout occured while transmitting packet Serial.println(F("timeout!"));
} else { // some other error occurred Serial.print(F("failed, code ")); Serial.println(state);
}
// wait for a second before transmitting again delay(1000); }
// Set TX pin HIGH and RX pin LOW to switch to TRANSMIT void enableTX(void) { digitalWrite(RADIO_RXEN, LOW); digitalWrite(RADIO_TXEN, HIGH); delay(100); }
And that's my reception code, if you see anything weird dont hesitate :)
/* RadioLib SX126x Receive Example
This example listens for LoRa transmissions using SX126x Lora modules. To successfully receive data, the following settings have to be the same on both transmitter and receiver:
preamble length
Other modules from SX126x family can also be used.
For full API reference, see the GitHub Pages https://jgromes.github.io/RadioLib/ */
// include the library
// Forward declaration of functions void enableRX(void);
// GPIOs to control E22 RX_EN and TX_EN
// SX1262 module = eByte E22-900M module SX1268 radio = new Module(15, 4, 4, 5); // 5 = NSS, 4 = DIO1 4 = DIO2 (not connected), 5 is BUSY
void setup() { //pinMode(1,FUNCTION_3); //pinMode(3,FUNCTION_3); Serial.begin(115200);
SPI.begin(); // SCLK GPIO 18, MISO GPIO 19, MOSI GPIO 23, CS == NSS GPIO 5
Serial.print(F("[SX1262] Initializing ... "));
// initialize SX1262 // carrier frequency: 868.0 MHz // bandwidth: 125.0 kHz // spreading factor: 7 // coding rate: 5 // sync word: 0x1424 (private network) // output power: 22 dBm // current limit: 60 mA // preamble length: 8 symbols // CRC: enabled int state = radio.begin(434.0, 125.0, 9, 7, 0x34, 10, 8, 1.6, false);
if (state == RADIOLIB_ERR_NONE) { Serial.println(F("success!")); } else { Serial.print(F("failed, code ")); Serial.println(state); while (true); }
// eByte E22-900M22S uses DIO3 to supply the external TCXO if (radio.setTCXO(1.8) == RADIOLIB_ERR_INVALID_TCXO_VOLTAGE) { Serial.println(F("Selected TCXO voltage is invalid for this module!")); }
// Important! To enable receive you need to switch the SX126x antenna switch to RECEIVE enableRX();
}
void loop() {
Serial.print(F("[SX1262] Waiting for incoming transmission ... "));
// you can receive data as an Arduino String // NOTE: receive() is a blocking method! // See example ReceiveInterrupt for details // on non-blocking reception method. String str; int state = radio.receive(str,0);
// you can also receive data as byte array /* byte byteArr[8]; int state = lora.receive(byteArr, 8);
*/ if (state == RADIOLIB_ERR_NONE) { // packet was successfully received Serial.println(F("success!"));
// print the data of the packet
Serial.print(F("[SX1262] Data:\t\t"));
Serial.println(str);
// print the RSSI (Received Signal Strength Indicator)
// of the last received packet
Serial.print(F("[SX1262] RSSI:\t\t"));
Serial.print(radio.getRSSI());
Serial.println(F(" dBm"));
// print the SNR (Signal-to-Noise Ratio)
// of the last received packet
Serial.print(F("[SX1262] SNR:\t\t"));
Serial.print(radio.getSNR());
Serial.println(F(" dBm"));
} else if (state == RADIOLIB_ERR_RX_TIMEOUT) { // timeout occurred while waiting for a packet Serial.println(F("timeout!"));
} else if (state == RADIOLIB_ERR_CRC_MISMATCH) { // packet was received, but is malformed Serial.println(F("CRC error!"));
} else { // some other error occurred Serial.print(F("failed, code ")); Serial.println(state);
} // wait for a second before transmitting again delay(1000); }
// Set RX pin HIGH and TX pin LOW to switch to RECEIVE void enableRX(void) { digitalWrite(RADIO_RXEN, HIGH); digitalWrite(RADIO_TXEN, LOW); delay(100); }
@Upergy -- I'll try to look at your code in the next week or two to see if I notice anything with it.
Here's my code for QMesh:
-Dan Fay
Hello, Thank you for your quick reply, i will try to clarify the code and sens you my Hardware set UP tomorow :)
Téléchargez Outlook pour Androidhttps://aka.ms/ghei36
From: faydr @.> Sent: Wednesday, June 8, 2022 3:26:32 PM To: jgromes/RadioLib @.> Cc: Upergy @.>; Mention @.> Subject: Re: [jgromes/RadioLib] Question for SX126x module: IRQs and DIO2 (#12)
@Upergyhttps://github.com/Upergy -- I'll try to look at your code in the next week or two to see if I notice anything with it.
Here's my code for QMesh:
-Dan Fay
— Reply to this email directly, view it on GitHubhttps://github.com/jgromes/RadioLib/issues/12#issuecomment-1149914476, or unsubscribehttps://github.com/notifications/unsubscribe-auth/AZQTHDIW2LCW4JWVCLYNZL3VOCNQRANCNFSM4HQ6GP3Q. You are receiving this because you were mentioned.Message ID: @.***>
I am connecting a eByte E22-900M22 module with the SX1262 chip. This module has DIO1 and DIO2 exposed, but DIO2 (in my setup) is used to control the RX/TX direction of the antenna. DIO3 is not available.
The initialization routine for the SX1262 module asks for 2 interrupt inputs. But I can only assign DIO1.
Questions:
The code is based on the SX126x_Receive.ino and SX126x_Transmit.ino examples: