Closed HamzaHajeir closed 3 years ago
When interrupt is activated, the module (ESP8266) just shuts off (I don't really know what happens there).
Sounds like not an issue in the library then - on ESP8266, if the software hangs, the internal watchdog resets it. If no reset occurs, then it looks like more of a hardware issue.
The Builtin LEDs just turns off
Maybe there's a pin collision then? I haven't used NodeMCU before.
Note : I can verify it's not a problem in interrupt by trying to touch D0 with 3.3V.
So you tied D0 to 3V3 and what happened then? Did the interrupt not occur? Did it occur but didn't hang? Keep in mind that the interrupt is edge-sensitive, if you literally just "touched" D0 with a wire connected to 3.3V, there will be at least several dozen edges and it will likely trigger the interrupt more than once.
Sounds like not an issue in the library then - on ESP8266, if the software hangs, the internal watchdog resets it. If no reset occurs, then it looks like more of a hardware issue.
I can't agree nor disagree.
So you tied D0 to 3V3 and what happened then? Did the interrupt not occur? Did it occur but didn't hang? Keep in mind that the interrupt is edge-sensitive, if you literally just "touched" D0 with a wire connected to 3.3V, there will be at least several dozen edges and it will likely trigger the interrupt more than once.
That's right, The interrupt just gets served without MCU gets 'hanged'.
I'll investigate more to the issue. and update with results/news.
It 'could' be ESP8266 pin interrupt not available on D0.
If DIO0 is to inform for 'packet sent'/'packet received', What does DIO1 do ? Is it necessary to provide the library with ?
Surely if D0 didn't support external interrupt, you wouldn't have been able to trigger it manually.
DIO1 is used in blocking receive and in channel activity detection to get the timeout event, it doesn't have to be used for interrupt receive (but it also shouldn't affect anything).
I don't know whether this is a new issue or we can continue here.
I've the other end node runs on another library, However When configurations are identical and equals to RadioLib default configurations, It received perfectly. Configurations include :
When I Activate CRC at both sides, Receiver (ESP8266 running RadioLib) doesn't receive.
But it received successfully when I put this line radio.setDio0Action(setFlag);
in the setup().
The code becomes :
#include <RadioLib.h>
#define D1 5
#define D0 16
#define D2 4
const int csPin = D1; // LoRa radio chip select
const int resetPin = D2; // LoRa radio reset
const int irqPin = D0; // change for your board; must be a hardware interrupt pin
const int DIO1 = 10; // change for your board; must be a hardware interrupt pin
const int DIO0 = irqPin; // change for your board; must be a hardware interrupt pin
// SX1278 has the following connections:
// NSS pin: 5 D1
// DIO0 pin: 16 D0
// RESET pin: 4 :D2
// DIO1 pin: 10 SD3
SX1278 radio = new Module(csPin, DIO0, resetPin, DIO1);
// or using RadioShield
// https://github.com/jgromes/RadioShield
//SX1278 radio = RadioShield.ModuleA;
void setup() {
Serial.begin(115200);
pinMode(LED_BUILTIN, OUTPUT);
// initialize SX1278 with default settings
Serial.print(F("[SX1278] Initializing ... "));
radio.setCRC(true);
int state = radio.begin();
if (state == ERR_NONE) {
Serial.println(F("success!"));
} else {
Serial.print(F("failed, code "));
Serial.println(state);
while (true);
}
radio.setDio0Action(setFlag);
}
void loop() {
Serial.print(F("[SX1278] Waiting for incoming transmission ... "));
// you can receive data as an Arduino String
// NOTE: receive() is a blocking method!
// See example ReceiveInterrupt for details
// on non-blocking reception method.
String str;
int state = radio.receive(str);
// you can also receive data as byte array
/*
byte byteArr[8];
int state = radio.receive(byteArr, 8);
*/
if (state == ERR_NONE) {
// packet was successfully received
Serial.println(F("success!"));
// print the data of the packet
Serial.print(F("[SX1278] Data:\t\t\t"));
Serial.println(str);
// print the RSSI (Received Signal Strength Indicator)
// of the last received packet
Serial.print(F("[SX1278] RSSI:\t\t\t"));
Serial.print(radio.getRSSI());
Serial.println(F(" dBm"));
// print the SNR (Signal-to-Noise Ratio)
// of the last received packet
Serial.print(F("[SX1278] SNR:\t\t\t"));
Serial.print(radio.getSNR());
Serial.println(F(" dB"));
// print frequency error
// of the last received packet
Serial.print(F("[SX1278] Frequency error:\t"));
Serial.print(radio.getFrequencyError());
Serial.println(F(" Hz"));
} else if (state == ERR_RX_TIMEOUT) {
// timeout occurred while waiting for a packet
Serial.println(F("timeout!"));
} else if (state == ERR_CRC_MISMATCH) {
// packet was received, but is malformed
Serial.println(F("CRC error!"));
} else {
// some other error occurred
Serial.print(F("failed, code "));
Serial.println(state);
}
}
// flag to indicate that a packet was received
volatile bool receivedFlag = false;
// disable interrupt when it's not needed
volatile bool enableInterrupt = true;
// this function is called when a complete packet
// is received by the module
// IMPORTANT: this function MUST be 'void' type
// and MUST NOT have any arguments!
ICACHE_RAM_ATTR void setFlag(void) {
// check if the interrupt is enabled
if (!enableInterrupt) {
return;
}
// we got a packet, set the flag
receivedFlag = true;
}
That could bring 2 things :
What do you think ?
I'm now enabling crc in receive with interrupt example, I'll update very soon
UPDATE
Please dismiss any information given that interrupt could be attached to D0. It's not.
Reordered pins, And works well now. If there's an issue it was the mentioned in my last comment (Enabling CRC requires setDIO0Flag()), That one can replicate easily, If not replicated, It's my incorrect setup again :) .
Regarding DIO1, Would it needs to be attached to an interruptible-pin ?
For Now I don't think there's a valid reason to let this issue opened. Closing.. Thanks for your kindly help @jgromes :)
Enabling CRC requires setDIO0Flag()
That seems very strange - by calling setDio0Flag() in blocking mode you attach interrupt to the DIO0 pin and only break out of the blocking wait loop to execute the ISR (which does nothing), so there should be no reason why that would be required to get CRC working.
What's the other library you have used? Does this happen when RadioLib is transmitting too? And what does "doesn't receive" mean in the previous sketch? Never receives any packets, hangs, or prints CRC errors?
DIO1 doesn't need an interrupt pin, it's only used in blocking loops.
That seems very strange - by calling setDio0Flag() in blocking mode you attach interrupt to the DIO0 pin and only break out of the blocking wait loop to execute the ISR (which does nothing), so there should be no reason why that would be required to get CRC working.
You're right, That's strange.
What's the other library you have used? Does this happen when RadioLib is transmitting too? And what does "doesn't receive" mean in the previous sketch? Never receives any packets, hangs, or prints CRC errors?
I mean by receiving : never receives any packet.
I'm exhausted trying to receive in the other end from this one, Could this library be ported to STM32CubeIDE using HAL ? and How ?
The other library is this one : https://github.com/wdomski/SX1278
My last issue (not receiving in the other end) could be an incorrect transmitter code. Trying to use interrupt while sending and receiving.
Describe the bug I'm using
SX127x_Receive_Interrupt
example to react in non-blocking mode, I'm using ESP8266 NodeMCU. The module works well in Receive mode (without interrupt).When interrupt is activated, the module (ESP8266) just shuts off (I don't really know what happens there). The Builtin LEDs just turns off.
The hang/shut occurs when the message is sent from the other side.
NodeMCU can be rebooted through reset pin. Note : I can verify it's not a problem in interrupt by trying to touch D0 with 3.3V.
Wiring
Ra-01 (SX1278) | NodeMCU NSS to D1 DIO0 to D0 RESET to D2 DIO1 to SD3 SCK to D5 MISO to D6 MOSI to D7
ESP8266 Arduino Core : 2.7.1 Configurations :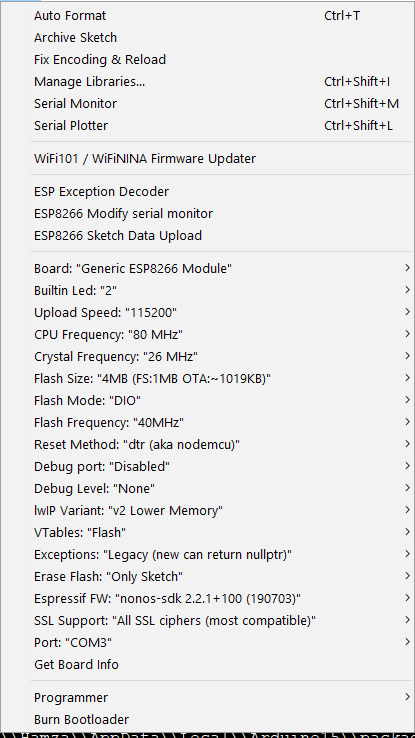
To Reproduce
Serial output
Additional info (please complete):