Closed JulesPruvost closed 1 year ago
Hello So I managed to make it stop disconnecting by removing:
// NOTE: some RF69 modules use high power output,
// those are usually marked RF69H(C/CW).
// To configure RadioLib for these modules,
// you must call setOutputPower() with
// second argument set to true.
Serial.print(F("[RF69] Setting high power module ... "));
int state = radio.setOutputPower(20, true);
if (state == RADIOLIB_ERR_NONE) {
Serial.println(F("success!"));
} else {
Serial.print(F("failed, code "));
Serial.println(state);
while (true);
}
And just making it default:
// initialize RF69 with default settings
Serial.print(F("[RF69] Initializing ... "));
int state = radio.begin();
if (state == RADIOLIB_ERR_NONE) {
Serial.println(F("success!"));
} else {
Serial.print(F("failed, code "));
Serial.println(state);
while (true);
}
But now I just receive nothing in my Serial Monitor, I'll try to debug in a second
Oh nevermind, I'm not able to debug on the raspberry pi Pico
Added an delay of 3 seconds and now I receive some output in the serial monitor: 06:36:56.911 -> [RF69] Initializing ... failed, code -2
In your first example, you cannot just call setOutputPower
- first you have to initialize the module with begin
, only after that you can call other methods. See the wiki and all of the examples.
Next, from the photo, it seems you are powering the RFM69 directly from some on-board power source for the RP2040. Make sure that source is sufficient to power the RFM69 during transmissions. If it is not, I would expect resets/brownouts of the RP2040.
Finally, the code -2 means that the module was not found, which usually signals some problem in the wiring. You did not specify any SPI bus, which means RadioLib will use the default SPI
. Googling Raspberry Pi Pico pinout, it seems you have connected the module to SPI1
- I don't know if that is correct, you should double-check.
Hello, Thanks for the quick reply. Is there any way I could set the SPI pins in the library?
See the Basics wiki page - you can, but your platform has to support it.
Not sure If I can ask this here but so I am using this Arduiuno core : https://github.com/arduino/ArduinoCore- But whenever I run my code with this:
#include <RadioLib.h>
#include <SPI.h>
MBbdSPI spi(MOSI_PIN, MISO_PIN, SCK_PIN);
SPISettings spiSettings(2000000, MSBFIRST, SPI_MODE0);
RF69 radio = new Module(CS_PIN, INT_PIN, RST_PIN, spi, spiSettings); // Module(cs, int, rst, gpio, &spi, spiSettings)
I Receive this error:
C:\Users\User\Documents\Arduino\pico-file.ino\pico-file.ino.ino:17:67: error: no matching function for call to 'Module::Module(int, int, int, arduino::MbedSPI&, arduino::SPISettings&)'
RF69 radio = new Module(CS_PIN, INT_PIN, RST_PIN, spi, spiSettings); // Module(cs, int, rst, gpio, &spi, spiSettings)
^
In file included from c:\Users\User\Documents\Arduino\libraries\RadioLib\src/RadioLib.h:39:0,
from C:\Users\Jules\Documents\Arduino\pico-file.ino\pico-file.ino.ino:4:
c:\Users\Jules\Documents\Arduino\libraries\RadioLib\src/Module.h:73:5: note: candidate: Module::Module(const Module&)
Module(const Module& mod);
^~~~~~
c:\Users\User\Documents\Arduino\libraries\RadioLib\src/Module.h:73:5: note: candidate expects 1 argument, 5 provided
In file included from c:\Users\Jules\Documents\Arduino\libraries\RadioLib\src/RadioLib.h:39:0,
from C:\Users\Jules\Documents\Arduino\pico-file.ino\pico-file.ino.ino:4:
c:\Users\User\Documents\Arduino\libraries\RadioLib\src/Module.h:49:5: note: candidate: Module::Module(pin_size_t, pin_size_t, pin_size_t, pin_size_t, arduino::SPIClass&, arduino::SPISettings)
Module(RADIOLIB_PIN_TYPE cs, RADIOLIB_PIN_TYPE irq, RADIOLIB_PIN_TYPE rst, RADIOLIB_PIN_TYPE gpio, SPIClass& spi, SPISettings spiSettings = RADIOLIB_DEFAULT_SPI_SETTINGS);
^~~~~~
c:\Users\User\Documents\Arduino\libraries\RadioLib\src/Module.h:49:5: note: no known conversion for argument 4 from 'arduino::MbedSPI' to 'pin_size_t {aka unsigned char}'
c:\Users\Jules\Documents\Arduino\libraries\RadioLib\src/Module.h:32:5: note: candidate: Module::Module(pin_size_t, pin_size_t, pin_size_t, pin_size_t)
Module(RADIOLIB_PIN_TYPE cs, RADIOLIB_PIN_TYPE irq, RADIOLIB_PIN_TYPE rst, RADIOLIB_PIN_TYPE gpio = RADIOLIB_NC);
^~~~~~
c:\Users\User\Documents\Arduino\libraries\RadioLib\src/Module.h:32:5: note: candidate expects 4 arguments, 5 provided
C:\Users\User\Documents\Arduino\pico-file.ino\pico-file.ino.ino: In function 'void setup()':
C:\Users\User\Documents\Arduino\pico-file.ino\pico-file.ino.ino:54:5: error: expected primary-expression before '/' token
* /
^
C:\Users\User\Documents\Arduino\pico-file.ino\pico-file.ino.ino:55:1: error: expected primary-expression before '}' token
}
^
You have to use SPIClass
, not MbedSPI
- that's what RadioLib expects. If the Arduino-mbed core doesn't work, RadioLib also supports this one: https://github.com/earlephilhower/arduino-pico
Apparently it's supposed to be better than the official.
I've tried that other library before and it seemed to be not working with my pico. Whenever I uploaded code with that core my arduino just disconnected like I said before and I couldn't see anything on my serial monitor because I had no serial monitor so I'd like to keep that for last case scenario.
Now when I reverenced referenced SPIClass with the nmbed core. It pointed me to a class HardwareSPI with a constructor with 0 params. https://github.com/arduino/ArduinoCore-API/blob/master/api/HardwareSPI.h). Don't think it's possible to change the SPI pins here.
Also I am using the default SPI pins which makes it weird that the normal SPI settings don't work: https://github.com/raspberrypi/pico-sdk/blob/master/src/boards/include/boards/pico.h
From what I could tell, HardwareSPI is just an API abstraction, you cannot create an instance of it (notice how all of its methods are pure virtual).
You can try to run the sketch in debug verbose mode (https://github.com/jgromes/RadioLib/wiki/Debug-mode), though I doubt much useful info will be shown.
So I've tried like 5 different SPI pinouts that I could find on google but just everything gives this, I'll give the other core a try. Lets hope it works :/
20:01:00.336 -> [RF69] Initializing ... R 10 0
20:01:00.336 -> RF69 not found! (1 of 10 tries) RADIOLIB_RF69_REG_VERSION == 0x0000, expected 0x0024
20:01:00.378 -> R 10 0
20:01:00.378 -> RF69 not found! (2 of 10 tries) RADIOLIB_RF69_REG_VERSION == 0x0000, expected 0x0024
20:01:00.378 -> R 10 0
20:01:00.378 -> RF69 not found! (3 of 10 tries) RADIOLIB_RF69_REG_VERSION == 0x0000, expected 0x0024
20:01:00.413 -> R 10 0
20:01:00.413 -> RF69 not found! (4 of 10 tries) RADIOLIB_RF69_REG_VERSION == 0x0000, expected 0x0024
20:01:00.413 -> R 10 0
20:01:00.413 -> RF69 not found! (5 of 10 tries) RADIOLIB_RF69_REG_VERSION == 0x0000, expected 0x0024
20:01:00.459 -> R 10 0
20:01:00.459 -> RF69 not found! (6 of 10 tries) RADIOLIB_RF69_REG_VERSION == 0x0000, expected 0x0024
20:01:00.459 -> R 10 0
20:01:00.459 -> RF69 not found! (7 of 10 tries) RADIOLIB_RF69_REG_VERSION == 0x0000, expected 0x0024
20:01:00.504 -> R 10 0
20:01:00.504 -> RF69 not found! (8 of 10 tries) RADIOLIB_RF69_REG_VERSION == 0x0000, expected 0x0024
20:01:00.504 -> R 10 0
20:01:00.504 -> RF69 not found! (9 of 10 tries) RADIOLIB_RF69_REG_VERSION == 0x0000, expected 0x0024
20:01:00.552 -> R 10 0
20:01:00.552 -> RF69 not found! (10 of 10 tries) RADIOLIB_RF69_REG_VERSION == 0x0000, expected 0x0024
20:01:00.552 -> No RF69 found!
20:01:00.552 -> failed, code -2
Hey So I managed to get it working with the SPI bus: but I am getting the error -16 now
Hey so I managed to get it working for some reason. I had to use GPx number for pins and not the actual pins on the board. Yeah idk so thanks anyway for the help.
Glad you were able to get it working, thanks for letting me know!
Sketch that is causing the module fail
Hardware setup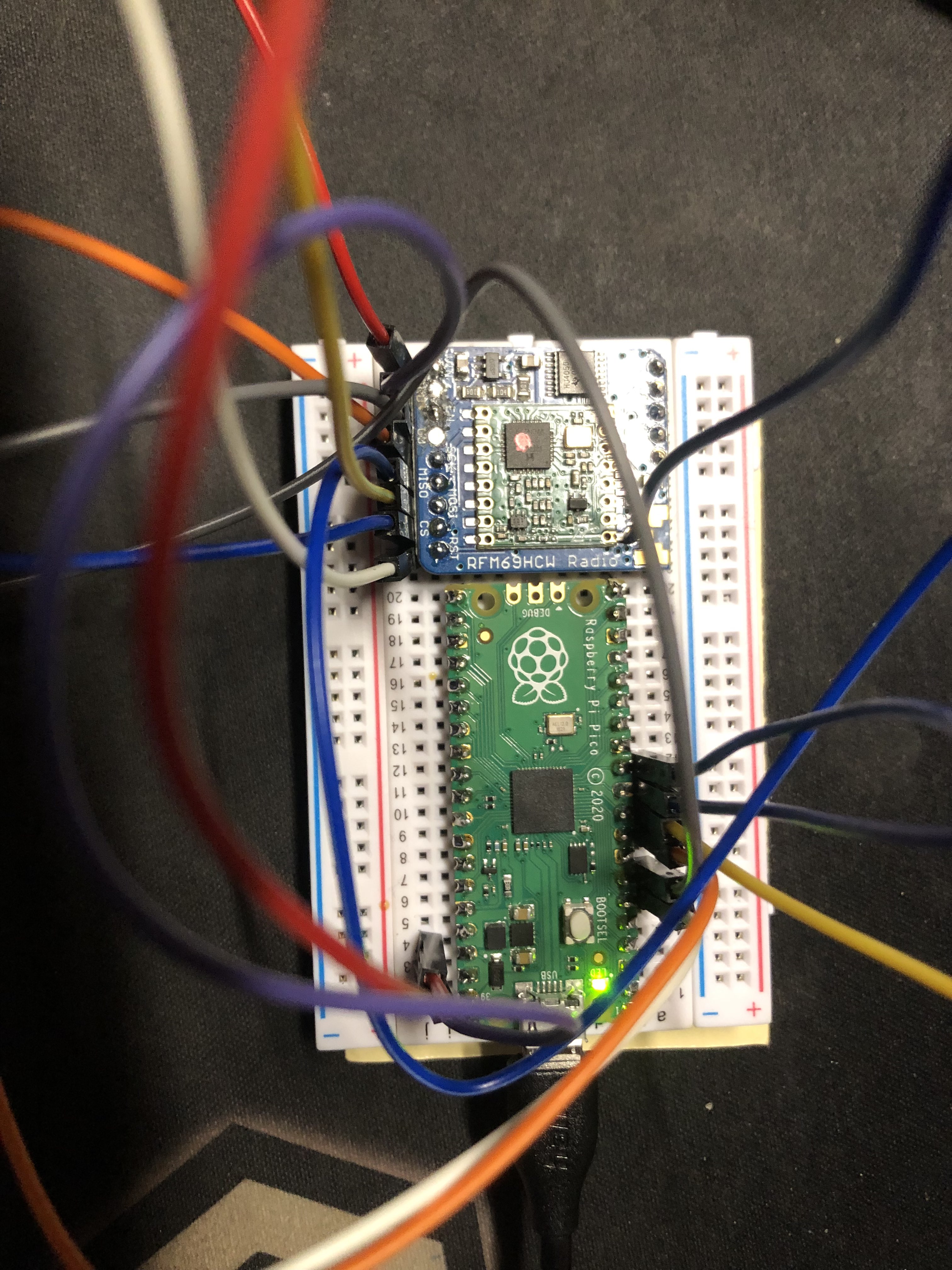
Debug mode output It just disconnects so I can't really give any debugging error here. I am receiving this windows error "A request for the USB device descriptor failed.". When uploading other programs, I just get a COM7 port where I can look at Serial Monitor. But here after uploading it just disconnects my Raspberry Pi Pico and the light on the pi keeps blinking
Additional info (please complete):