Closed valtver closed 2 years ago
Hello,
Can you doublecheck that the transform is actually there? The transform object is only there if you are setting a texture from a spritesheet or the model you are loading is using texture transform.
console.log(mesh.material.baseColorTexture.transform)
// Add transform if it's not there
mesh.material.baseColorTexture.transform = new TextureTransform()
Hey,
Thanks, when I create transform during material setup it works. Just in case, I'm attaching modified "standard material" example code with working scale manipulation. So I guess that transform is there.
import { Application } from "pixi.js";
import {
Model,
LightingEnvironment,
ImageBasedLighting,
CameraOrbitControl,
Color,
StandardMaterialTexture,
TextureTransform
} from "pixi3d";
import "./styles.css";
let app = new Application({
backgroundColor: 0xdddddd,
resizeTo: window,
antialias: true
});
document.body.appendChild(app.view);
app.loader.add(
"diffuse.cubemap",
"https://raw.githubusercontent.com/jnsmalm/pixi3d-examples/master/assets/chromatic/diffuse.cubemap"
);
app.loader.add(
"specular.cubemap",
"https://raw.githubusercontent.com/jnsmalm/pixi3d-examples/master/assets/chromatic/specular.cubemap"
);
app.loader.add(
"teapot.gltf",
"https://raw.githubusercontent.com/jnsmalm/pixi3d-examples/master/assets/teapot/teapot.gltf"
);
const walltexture = StandardMaterialTexture.from(
"https://upload.wikimedia.org/wikipedia/commons/thumb/6/60/Surfaces_bumpy_walkway_between_sidewalk_and_street_closeup_view.JPG/1280px-Surfaces_bumpy_walkway_between_sidewalk_and_street_closeup_view.JPG"
);
walltexture.transform = new TextureTransform();
walltexture.transform.offset += Math.random()*100;
// const walltexture = StandardMaterialTexture.from(
// "https://static.wikia.nocookie.net/portalworldsgame/images/a/a7/Background_Block_Icon.png"
// );
var model = new Model();
app.loader.load((_, resources) => {
// Create and add the model, it uses the standard material by default.
model = Model.from(resources["teapot.gltf"].gltf);
app.stage.addChild(model);
model.y = -0.8;
model.meshes.forEach((mesh) => {
mesh.material.unlit = false; // Set unlit = true to disable all lighting.
// mesh.material.baseColor = new Color(1, 1, 1, 1); // The base color will be blended together with base color texture (if available).
mesh.material.baseColorTexture = walltexture;
console.log(mesh.material.baseColorTexture);
mesh.material.alphaMode = "opaque"; // Set alpha mode to "blend" for transparency (base color alpha less than 1).
mesh.material.exposure = 2; // Set exposure to be able to control the brightness.
mesh.material.metallic = 0; // Set to 1 for a metallic material.
// mesh.material.roughness = 0.3; // Value between 0 and 1 which describes the roughness of the material.
});
// Create the image-based lighting (IBL) object which is used to light the model. Depending on which IBL environment is used, it will greatly affect the visual appearence of the model.
let ibl = new ImageBasedLighting(
resources["diffuse.cubemap"].cubemap,
resources["specular.cubemap"].cubemap
);
LightingEnvironment.main = new LightingEnvironment(app.renderer, ibl);
});
let control = new CameraOrbitControl(app.view);
control.angles.x = 25;
var t = 0;
app.ticker.add(() => {
model.meshes.forEach((mesh) => {
t++;
mesh.material.baseColorTexture.transform.scale.set( Math.sin(t/100), Math.sin(t/100) );
})
});
If you just load a regular jpg image it won't include a transform by default. Your code must have done something different before, because if I run your code using "transform.scale.set" it's doesn't work if I don't create the texture transform. This is working as intended :-)
Okay, looks like
mesh.material.baseColorTexture.transform = new TextureTransform()
works as expected so I close that :)
Thanks again
Hi,
Faced texture offset issue. TextureTransform offset is not settable due to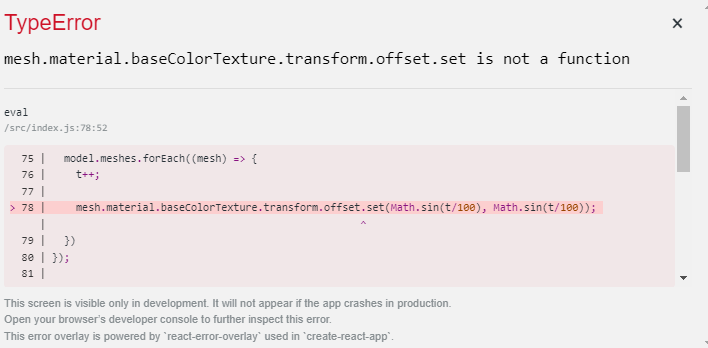
(Meanwhile scale.set() works ok)