Open muntazir143 opened 2 years ago
Try comment this line:
builder: DevicePreview.appBuilder,
@jonataslaw yaa it throwing bug if we are using device preview.
@jonataslaw The issue still persists even after removing the device preview dependency. Unexpected null value. If I hot reload with having that error then it also throws this.
@jonataslaw Have you found any solution? I'm in the middle of a project. Please look into it.
I'm building my app for flutter web and using getx as state management. This is the error I'm getting consistently and I can't get any solution looking up the internet.
This is my main page code
This is the error I'm getting:
Screenshots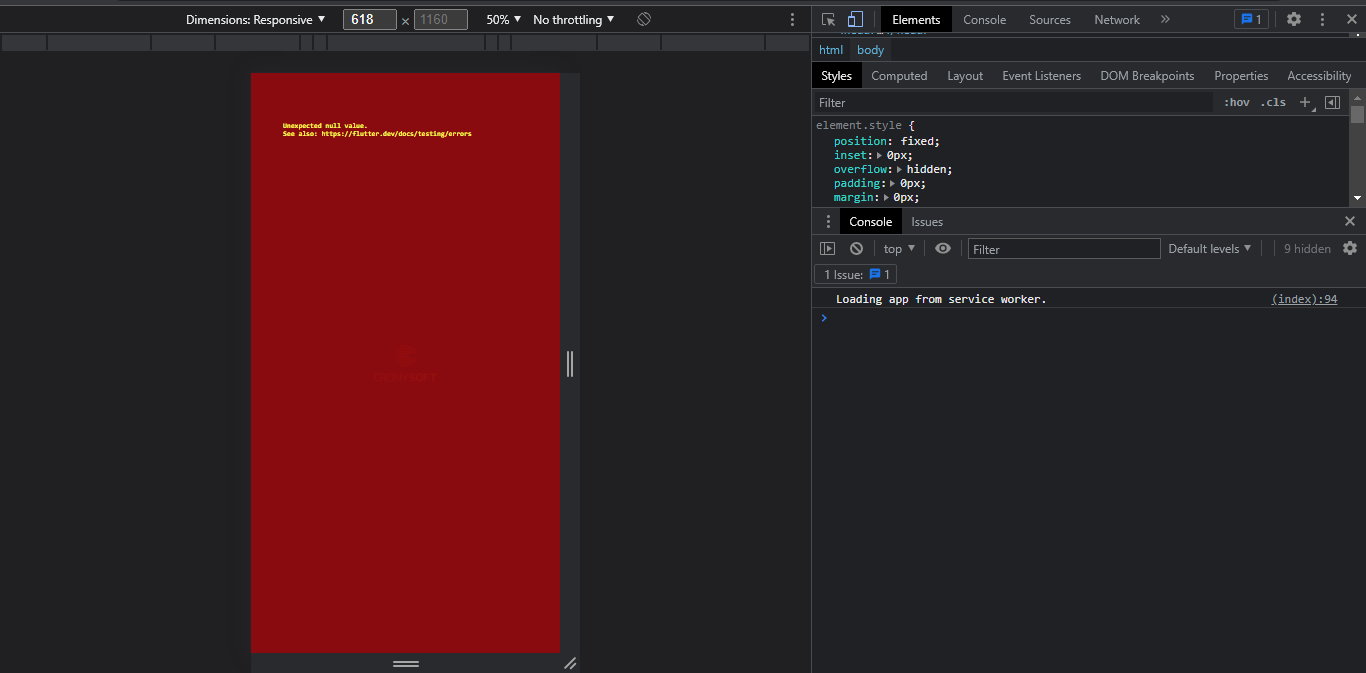
Flutter Version: Flutter 2.10.0
Getx Version: get: ^4.6.1
Describe on which device you found the bug: Chrome