Closed AshishS-1123 closed 3 years ago
@jostbr It's been a long time since I opened this PR. Yet you haven't responded. If this is a dead project, tell me right away so that I can stop wasting my time.
@AshishS-1123 My apologies for being AWOL! Thank you for your contributions. I merged #35, this means #36 have conlfict in src/maze.py. Are you able to see what that is and then I can proceed to merge #36?
@jostbr I have fixed the merge conflict. I modified the generate_maze function in src/maze.py to include the conditional statements that call the different generation algorithms. Branch can now be merged.
Changes Made
Why?
There are a lot of different algorithms for creating mazes and solving them and each of these algorithms have their pros and cons. To make this repository complete, it is necessary to include as many of these algorithms as possible.
How?
The Binary Tree Algorithm
The binary tree algorithm is very simple and involves looping through all cells in the maze only once.
The Generation Path Algorithm
In this algorithm, we are visiting the cells serially, row by row. But for showing the path generation animation, we cannot use this path, because it generates the wrong results.
Instead, we use a hack that generates the correct maze and sheds some light on why the algorithm is called binary tree algorithm.
The Binary Tree Algorithm is called so because, the maze generated can be represented as a binary tree. To understand this better, consider the following maze generated using the binary tree method.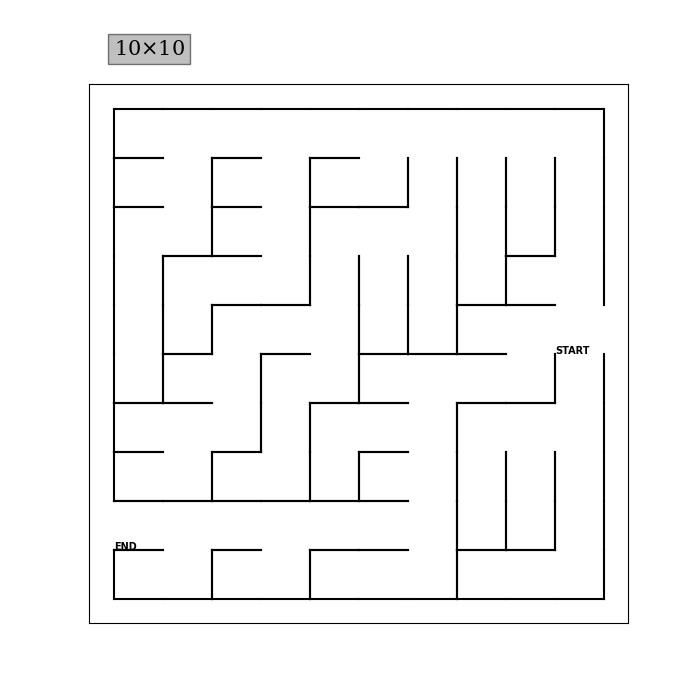
This maze can be represented as a binary tree as follows,
So, in the generation algorithm, I have used this concept and traversed the maze using dfs as can be seen using the show_generation_algorithm.
Unittests
Unittests have been added for algorithm.py in tests/algorithm_tests.py. To make testing of new algorithms easier, I have created a list of all maze generation algorithms in algorithm.py. In algorithm_tests.py, each test is run for all the algorithms in this list. This way, when a new maze generation algorithm is added, there is no need to write unittests for it separately.
The following tests have been added,