Closed CodeXtinction closed 5 years ago
I'm running into this too. Is there a workaround?
here's my code if that's helpful:
import React, { Component, Fragment } from 'react';
import BigCalendar from 'react-big-calendar'
import moment from 'moment'
import events from './events';
import availability from './availability';
import 'react-big-calendar/lib/css/react-big-calendar.css';
import './calendar-overrides.css';
const localizer = BigCalendar.momentLocalizer(moment) // or globalizeLocalizer
// identify busy slots with custom class
const slotPropGetter = (date) => {
const isAvailable = availability.some(({ start, end }) =>
moment(date).isBetween(start, end)
);
return isAvailable ? { style: { background: 'rgba(0, 203, 196, 0.12)' } } : {};
}
const eventPropGetter = (event) => {
switch(event.status) {
case 'canceled':
return {
style: { background: '#a09eaf', borderColor: '#a09eaf'}
}
case 'busy':
return {
style: { background: '#413e60', borderColor: '#413e60'}
}
default:
return {
style: { background: 'rgba(0, 132, 137, 0.8)', borderColor: 'rgba(0, 132, 137, 0.8)'}
}
}
};
const Event = ({ event }) =>
<div>
<div><strong>{event.title}</strong></div>
{ event.status !== 'busy' &&
(
<Fragment>
<div>{`${moment(event.start).format('h:mm')}-${moment(event.end).format('h:mm')}`}</div>
<div>{event.location}</div>
<div>{event.status === 'canceled' && '(Canceled)'}</div>
</Fragment>
)
}
</div>
const genHeaderCell = (handleNavClick, selectedDate) => ({ date }) => {
return (
<div>
{moment(date).day() === 0 && <button onClick={() => handleNavClick(new Date(moment(selectedDate).subtract(1, 'week').utc().format()))}>{'<'}</button>}
{moment(date).day() === 6 && <button onClick={() => handleNavClick(new Date(moment(selectedDate).add(1, 'week').utc().format()))}>{'>'}</button>}
<div>
{moment(date).format('ddd')}
</div>
<div>
{moment(date).format('MMM D')}
</div>
</div>
);
};
const genGutterHeader = (handleTodayClick) => () => {
return (
<div style={{ width: '100%', height: '100%', display: 'flex', alignItems: 'center', justifyContent: 'center' }}>
<button onClick={() => handleTodayClick(new Date())}>Today</button>
</div>
)
};
class Schedule extends Component {
state={
selectedDate: new Date()
}
setSelectedDate = (date) => {
this.setState({ selectedDate: date })
}
render() {
console.log(this.state.selectedDate);
return (
<BigCalendar
selectable
date={this.state.selectedDate}
localizer={localizer}
events={events}
startAccessor="start"
endAccessor="end"
defaultView="week"
views={['week']}
step={15}
timeslots={4}
getNow={() => new Date()}
onSelectEvent={event => console.log('clicked event', event)}
onSelectSlot={slot => console.log('create event here', slot)}
components={{
event: Event,
timeGutterHeader: genGutterHeader(this.setSelectedDate),
toolbar: () => null,
week: {
header: genHeaderCell(this.setSelectedDate, this.state.selectedDate)
},
}}
slotPropGetter={slotPropGetter}
eventPropGetter={eventPropGetter}
/>)
}
}
const App = () => (
<Schedule />
)
export default App;
add this line to DayColumn.js componentWillReceiveProps function: this.props.isNow && this.positionTimeIndicator()
This issue has been automatically marked as stale because it has not had recent activity. It will be closed if no further activity occurs. Thank you for your contributions.
Hi Community,
On clicking the Month, Week, Day, Agenda buttons the rbc-active class is applied to the clicked buttons and the button remains hightlighted. But on clicking Today, Back, Next the button gets hightlighted but when we click outside the button the style gets lost. How can we achieve the same style behaviour for TODAY,NEXT,BACK as it is going for MONTH,WEEK,DAY,AGENDA.
https://jquense.github.io/react-big-calendar/examples/index.html?path=/docs/props--drilldown-view
Do you want to request a feature or report a bug?
Bug
What's the current behavior?
current time highlighted is reset on navigation
got to the app demo
Correct highlighted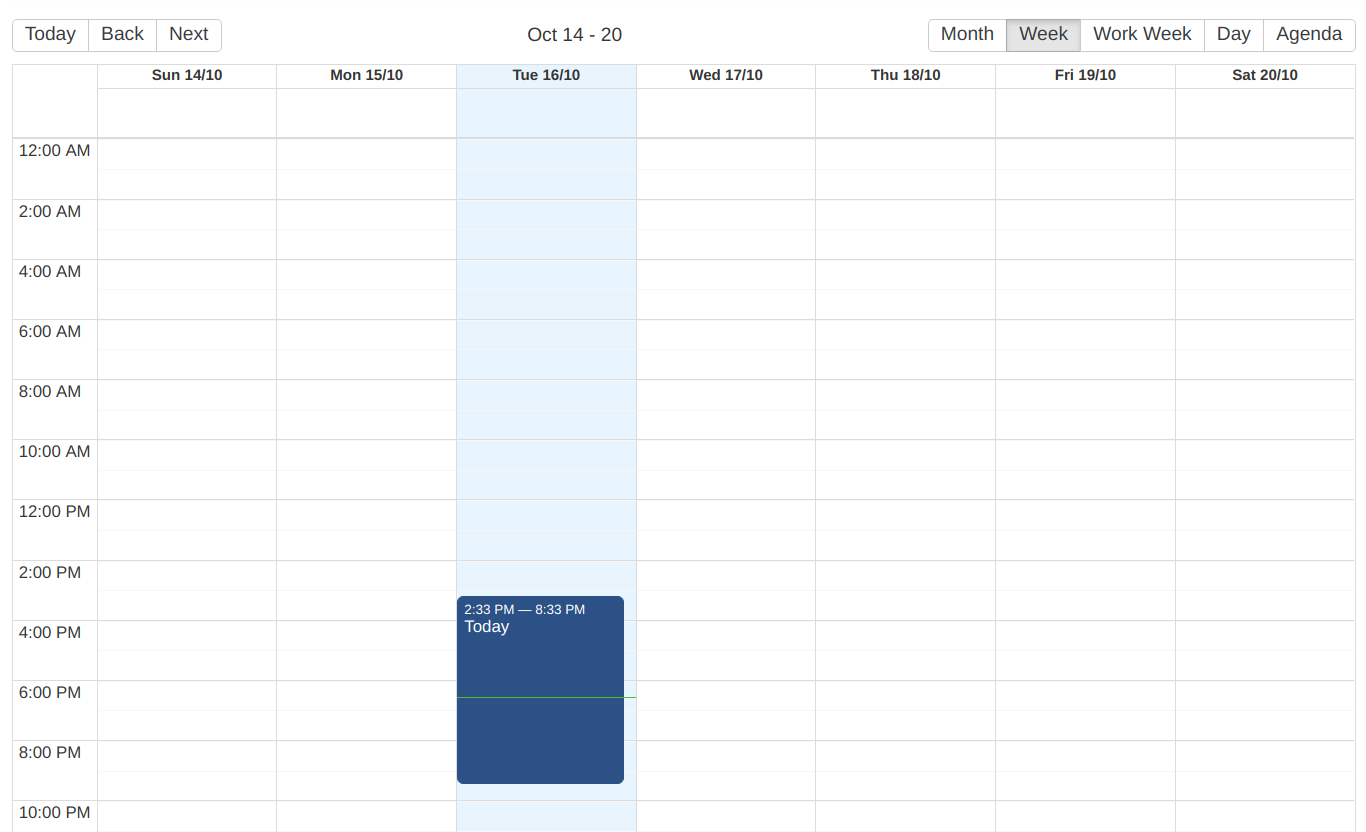
wrong highlighted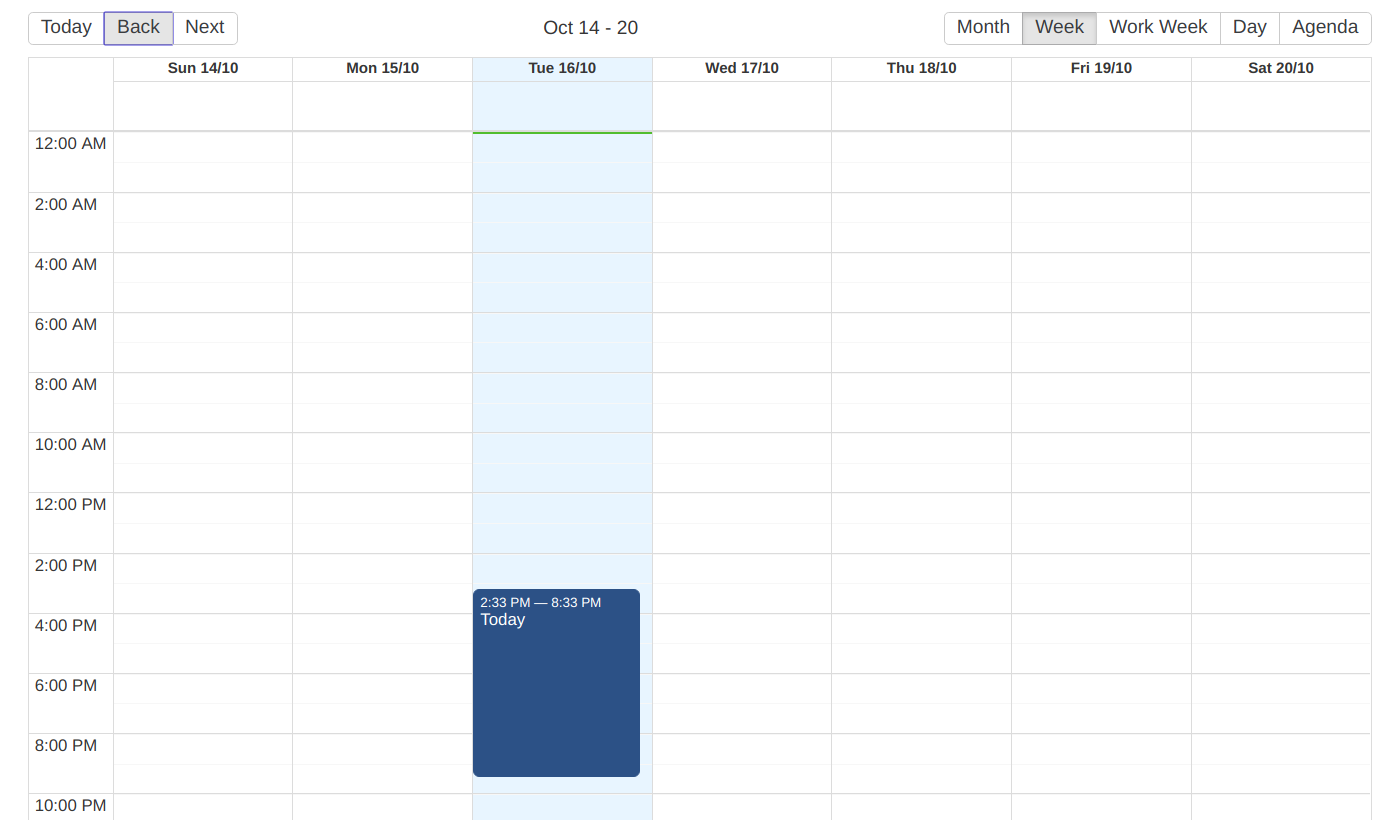
What's the expected behavior?
time highlighted should not change