Closed WolfgangFahl closed 2 years ago
I am using
docker run --publish=8080:8080 --name openjscad --volume $HOME/openjscad/workspace:/openjscad/packages/web/examples/workspace bitplan/openjscad:latest
as a workaround to access a V1 via http://localhost:8080 - i think this info should be more prominent for users who can't use V2 due to it's incompatibilities.
I love the
and to see that things are working with
and having the editor right there:
So all three things are missing in V2 out of the box. I'd appreciate if the V1 behavior would be made the default and V2 features are only optional.
And seeing my design:
Helps a lot ...
Hello, I think that your design can be adapted to V2 syntax. Roughly you only need to change lines like
function main() {
return (rotate([0,90,0],lock()).translate([0,0,54]));
}
to
const main = () => {
return translate([0,0,54], rotate([0,90,0],lock()));
}
and start your script with requires like
const jscad = require('@jscad/modeling')
const { curves, maths, extrusions, primitives, transforms, booleans,
colors, geometries, measurements, utils } = jscad
const { bezier } = curves
const { slice, extrudeLinear } = extrusions
const { cuboid, polygon, polyhedron } = primitives
const { intersect, subtract,union } = booleans
const { center, scale, translateX, translateY, translateZ, translate
,rotateX, rotateY, rotateZ, rotate } = transforms
const { colorize } = colors
const { geom3, poly3 } = geometries
const { vec3 } = maths
const { measureBoundingBox, measureArea } = measurements
const { degToRad } = utils
@WolfgangFahl I understand your frustration, as switching versions is sometimes scary, and often tedious task.
I have created a discussion page that explains a bit about how to migrate from V1 to V2 https://github.com/jscad/OpenJSCAD.org/discussions/883.
hope you convert more of your scripts to V2, feel free to ask more qustions if you have issues doing that
also if you have a bit of patience try using your fav text editor, save file. drag drop on openjscad and use "auto reload"
I was doing so by converting your script to V2 and writing down important steps.
here is a version of your script that works in V2
const jscad = require('@jscad/modeling')
const { connectors, geometry, geometries, maths, primitives, text, utils, booleans, expansions, extrusions, hulls, measurements, transforms, colors } = jscad
const { cuboid, sphere, circle, star,cylinderElliptic, polyhedron, roundedRectangle, rectangle } = primitives
const { translate, scale, center } = transforms
const { union, subtract, intersect } = booleans
const { path2, geom2, geom3, poly2, poly3 } = geometries
const { slice, extrudeFromSlices, extrudeLinear, extrudeRectangular, extrudeRotate, project } = extrusions
const { mat4, line2, line3, vec2, vec3, vec4 } = maths
// to make this conversion to V2 easier, some functions are not importd, but implemented as compat layer
// rotate in V2 uses radinas instad of degrees
const rotate = (angles,...parts)=>transforms.rotate(angles.map(utils.degToRad), ...parts)
// in V2 cube is ony for actual cubes with all of the sides same size
const cube = (params)=>cuboid(params)
// change in parameter names for cylinder
const cylinder = ({h,r})=>primitives.cylinder({radius:r, height:h})
// difference is subtract in V2
const difference = subtract
// title : BikeCaseLock
// author : Wolfgang Fahl
// license : Apache License
// revision : 0.0.3
// tags : Bike,Lock
// file : BikeCaseLock/main.jscad
// date : 2020-04-06
// construct a cylinder at the given position
function cylinderAt(list,h,r,dx,dy,dz,rx,ry,rz) {
list.push(translate([dx, dy, dz+h/2], rotate([rx,ry,rz],cylinder({
h: h,
r:r,
}))));
// console.trace('cylinderAt',list[list.length-1],{h,r,dx,dy,dz,rx,ry,rz})
}
// construct a cube at the given position
function cubeAt(list,w,l,h,dx,dy,dz,rx,ry,rz) {
list.push(translate([dx, dy, dz+h/2],rotate([rx,ry,rz],cube({
size: [w,l,h],
}))));
// console.trace('cubeAt',list[list.length-1],{w,l,h,dx,dy,dz,rx,ry,rz})
}
function lockholder() {
h=9.6;
hr=1.5;
hd=2*hr;
w=15.3;
l=9.6;
var parts = [];
var holes = [];
parts.push(translate([0,0,h/2],cube({
size: [w, l, h],
})));
cylinderAt(holes,l,hr*1.1,-3.5,-2,0,0,0,0);
cylinderAt(holes,l,hr,5.7,0,0,90,0,0);
cylinderAt(holes,h,3,8,0,4.4,90,0,0);
// return [parts,colors.colorize([1,0,0,0.5],holes)]
return difference(union(parts),union(holes));
}
// the joint for moving the lock
function lockjoint(height,w,wh,dx,dy) {
var parts = [];
var holes = [];
hr=1.5;
t=9
h=t+1
l=t-1
ddy=-w/2+dy-5
dzl=height/2-h/2
ddx=dx+1
cubeAt(parts,l,t,h,ddx,ddy,dzl,0,0,0);
cylinderAt(holes,h,hr*1.1,ddx,ddy,dzl,0,0,0);
// return [parts,colors.colorize([1,0,0,0.5],holes)]
return difference(union(parts),union(holes));
}
// the moving part
function lockmover(height,w,wh,dxw,dy) {
var parts = [];
var holes = [];
r=54;
rh=15;
dz=-40
dx2=5
cubeAt(holes,r*2-dx2,w,2*r+20,-dx2/2,0,-r,0,0,0)
for (zf=-1;zf<=1;zf+=2)
cubeAt(holes,15,w,height,r-5,0,zf*height,0,0,0)
cylinderAt(parts,w,r,0,0,dz/2,90,0,0)
cylinderAt(holes,wh,rh,dxw,45.5,1,0,90,0)
// return [parts,colors.colorize([1,0,0,0.5],holes)]
return difference(union(parts),union(holes));
}
// the handle for the lock
function lockhandle(height,w,wh,dx,dy) {
var parts = [];
var holes = [];
lhh=6
lhl=4
lhw=22
lhd=7
ldx=dx+0.6
ldz=height/2
ldy=dy+lhd+1.8
lockwidth=10
lockdepth=lhh+3.5
// 3 cubes for locking
lhl2=lhl+1
cubeAt(parts,lhh,lhw,lhl2,ldx,ldy,ldz-lhl2/2,0,0,0);
cubeAt(parts,lhh,lhw-lhd,lhl2,ldx+lhh-1,ldy-5,ldz-lhl2/2,0,0,0);
cubeAt(parts,lockdepth,lockwidth,lhl,ldx-lhh,ldy,ldz-lhl/2,0,0,0);
// one cube for strength
cl=10
cubeAt(parts,cl,cl,cl,ldx,ldy-lhw/2-cl/2,ldz-cl/2,0,0,0);
// cut off a bit
rs=lhh
cylinderAt(holes,wh,rs,dx-rs/2,dy+lhw,0,0,0,0)
// return [parts,colors.colorize([1,0,0,0.5],holes)]
return difference(union(parts),union(holes));
}
// the lock itself
function lock() {
var parts = [];
var holes = [];
// the height
height=26.7
w=69
wh=25
dx=44
dy=13
parts.push(lockjoint(height,w,wh,dx,dy));
slh=6.5
for (fz=-1;fz<=1;fz+=2)
cylinderAt(holes,slh,10,dx-2.5,-26,fz*(slh+2.5)+height/2-3.5,0,0,0);
parts.push(lockhandle(height,w,wh,dx,dy))
parts.push(lockmover(height,w,wh,dx,dy))
// spring
sr=5.2
sd=4.5
sdx=dx+sd
sdy=-w/2+dy+2*sr
sdz=height/2-sr/2
cylinderAt(holes,sd,sr ,sdx,sdy,sdz,0,90,0,0)
cylinderAt(parts,sd,sr+1,sdx,sdy,sdz,0,90,0,0)
// return [parts,colors.colorize([1,0,0,0.5],holes)]
return difference(union(parts),union(holes));
}
function main() {
// let ret = lock()
// console.log(ret);
// return ret;
return translate([0,0,54], rotate([0,90,0],lock()));
}
module.exports = {main}
and again no issue tracker no link to the github repository - no link to these discussions.
Both Discord Community and Open Issue links have been added to the latest version of the WEB UI at openjscad.xyz
Great. I still think github discussion should be more prominent. The legacy forum is IMHO awkward to use.
For me the forum is not that bad, but email notifications are not going through, so I have to repeatedly open the forum to see if there is something new :(
@WolfgangFahl please open this again if there are outstanding issues. It seems that all the documentation issues have been addressed, and a discussion has been added to assist those converting from V1 to V2.
See http://nicescad.bitplan.com/ and https://ochafik.com/openscad2/ for much simpler approaches that are possible these days.
@WolfgangFahl those are openscad, not openjscad, I am sure openscad users will be more interested in those.
We are aware that there are much more things that could be done to make jscad better, and our time is limited so we are making changes anytime we can.
I will disagree with your observation that those two pages show anything of: "much simpler approaches".
It is very easy to be a critic, let me show you.
your link does not even stretch with the browser :P
I am petty, and will argue, but I am also open to cooperation if in time in the future there will be overlap and integrations with openscad. you can join discord https://discord.gg/AaqGskur93 there is also an openscad channel on cadhub server
@hrgdavor thx for the feedback. I'll have to look whether https://nicegui.io/ https://quasar.dev/ or nicescad itself need to be improved here. I'll not put the current state of nicescad into the front row (yet). http://www.implicitcad.org/editor is e.g. a project that has already 1.2 k stars - all these projects IMHO focus too much on technology and not enough on e.g. interoperability. Having many options on the other hand is a benefit. So we have to balance. I am happier with not trying the OpenJscad approach (any more) and sticking to python since that's my preferred language - not because there is something bad about javascript or rust or any other language.
@WolfgangFahl That is exactly the same reason I prefer openjscad. I prefer to code in Javascript, regardless how much better is openscad, the special language used there is just too freaky to me. I also can not stand python syntax, so that one is also no-go for me.
Discussed in https://github.com/jscad/OpenJSCAD.org/discussions/814
In the meantime i think this is a bug.
I had a frustrating experience to day. I wanted to work on an existing openjsacd V1 design. When i tried to open https://www.openjscad.org/ i got: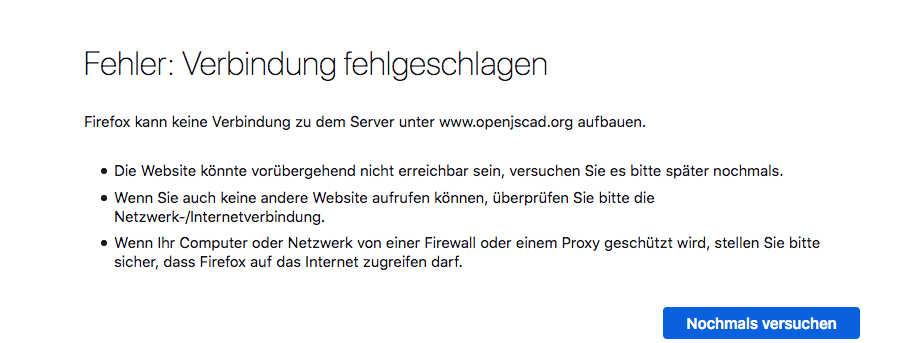
So i tried searching for openjscad github and found: https://github.com/jscad/OpenJSCAD.org
which pointed me to https://www.openjscad.xyz/ for an online version.
I dragged and dropped my design (after first not knowing where to drop since the V1 drop area seems to be gone in V2) and got the error message: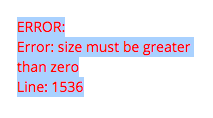
which is not userfriendly and not helpful especially since a link to the issue tracker is missing. I clicked on
and again no issue tracker no link to the github repository - no link to these dicussions.
So i can't use V1 and I cant' use V2 online any more. I'll have to start my own copy of V1 to continue which is very awkward and in my opinon not the goal of creating new Versions of software.
This issue is about the links to github / the issue tracker and discussions missing. I'll create another issue for the error message itself.
v1 Design that has a problem