Open oleksii-tatarintsev opened 1 year ago
@oleksii-tatarintsev have you found any workaround? I'm in the same exact situation
Have you tried with what mentioned in this other issue? https://github.com/juliansteenbakker/mobile_scanner/issues/539#issuecomment-1483740222 It works for me calling controller.stop() in the initState method
@override
void initState() {
controller.stop(); ---> call stop here
super.initState();
}
I was just running into a very similar issue and have found a workaround that works for me.
instead of defining the MobileScannerController in the Scanner class definition, pass it through as a parameter upon instantiation.
this example code may be easier to understand.
class MyHomePage extends StatefulWidget {
const MyHomePage({super.key});
@override
State<MyHomePage> createState() => _MyHomePageState();
}
class _MyHomePageState extends State<MyHomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(),
body: Center(
child: Column(
children: [
TextButton(
child: Text("BARCODE SCANNER"),
onPressed: () {
Navigator.push(
context,
MaterialPageRoute(
builder: (context) => ScannerScreen(
controller: MobileScannerController(
autoStart: true,
),
),
),
);
},
),
],
),
),
);
}
}
class ScannerScreen extends StatelessWidget {
const ScannerScreen({
super.key,
required this.controller,
});
final MobileScannerController controller;
@override
Widget build(BuildContext context) {
return MobileScanner(
onDetect: (data) {
controller.stop();
Navigator.pop(context);
},
controller: controller,
);
}
}
as you can see, I create a new controller each time the ScannerScreen is navigated to, and pass it through as a parameter of ScannerScreen.
None of the workarounds seemed to work. What ended up working was adding a delay between stopping and starting the controller:
@override
void initState() {
controller.stop();
Future.delayed(const Duration(milliseconds: 1000), () => controller.start());
super.initState();
}
Not a great solution, but atleast it works. Im running this on a OnePlus Nord N100 (cheap, old phone) in debug mode. Im guessing that the timeout can be shorter on a newer phone running a production build.
None of the workarounds seemed to work. What ended up working was adding a delay between stopping and starting the controller:
@override void initState() { controller.stop(); Future.delayed(const Duration(milliseconds: 1000), () => controller.start()); super.initState(); }
Not a great solution, but atleast it works. Im running this on a OnePlus Nord N100 (cheap, old phone) in debug mode. Im guessing that the timeout can be shorter on a newer phone running a production build.
I think you could just await the controller to stop
await controller.stop(); await controller.start();
OR
await controller.stop().whenComplete(() => controller.start());
In my case, when scanning the QR I go to a new screen where I perform an action and make a pop to return to the QR scan. The following code worked for me, for some reason Future.delayed is a must; otherwise it doesn't work. Remember to set autoStart: false
final MobileScannerController mobileScannerController = MobileScannerController(autoStart: false);
@override
void initState() {
super.initState();
_startCamera();
}
@override
void dispose() {
super.dispose();
mobileScannerController.stop();
mobileScannerController.dispose();
}
Future<void> _startCamera() async {
Future.delayed(const Duration(seconds: 1), () async => await mobileScannerController.start());
}
Every time when i am trying to navigate from one screen to another (both screens use MobileScanner), on the second screen i receive white screen if i do not use autostart and starting/stopping controller by myself. If i use autostart, i receive black screen
Package: mobile_scanner: 3.2.0 iOS Device: iPad Pro 12.9 inch (5th generation) iPadOS: 16.4 Android Device: Samsung Galaxy Tab S7 Android version: Android 11
Code:
Console: output:
Actual result when autostart false: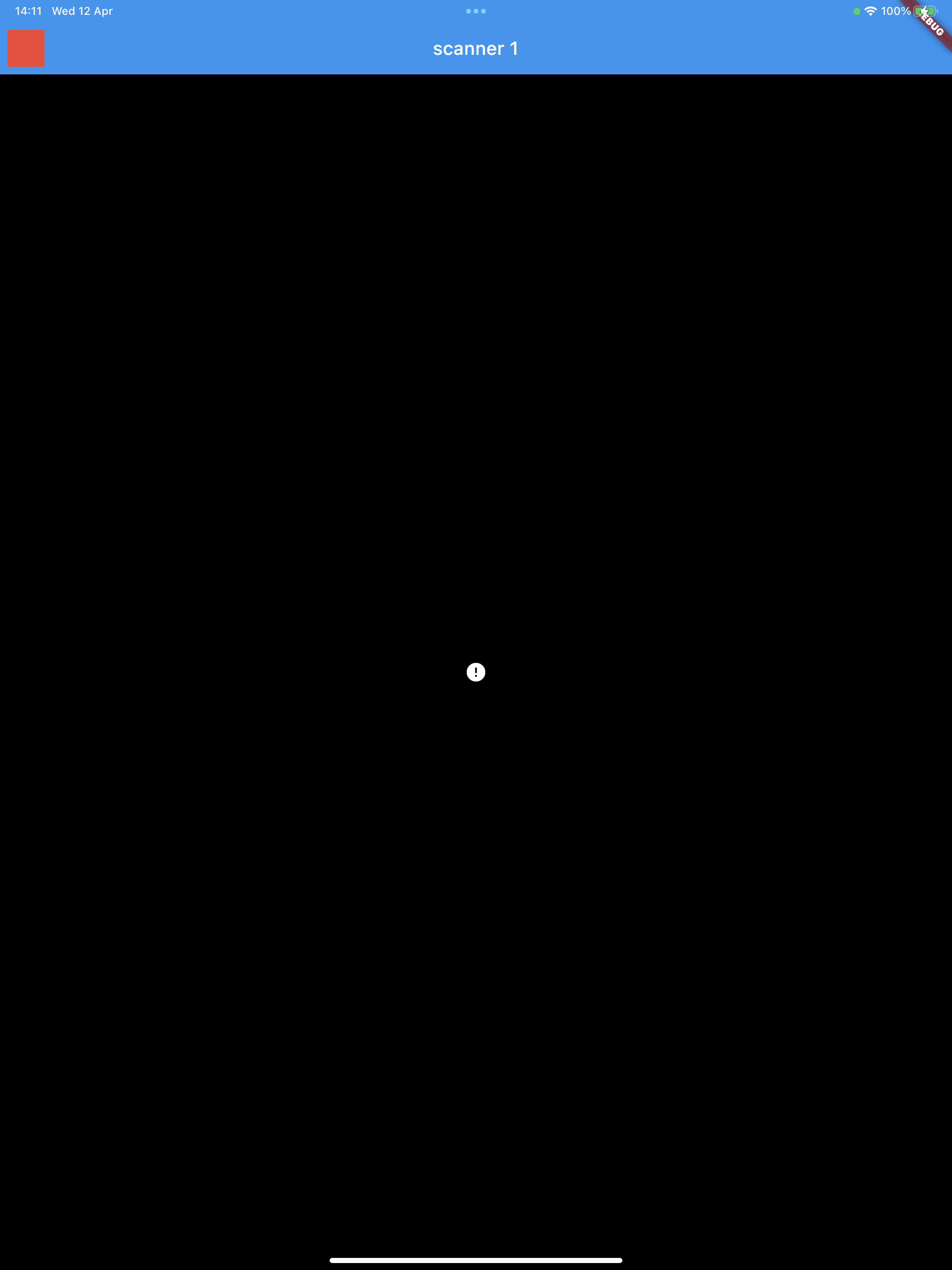
Actual result when autoStart true: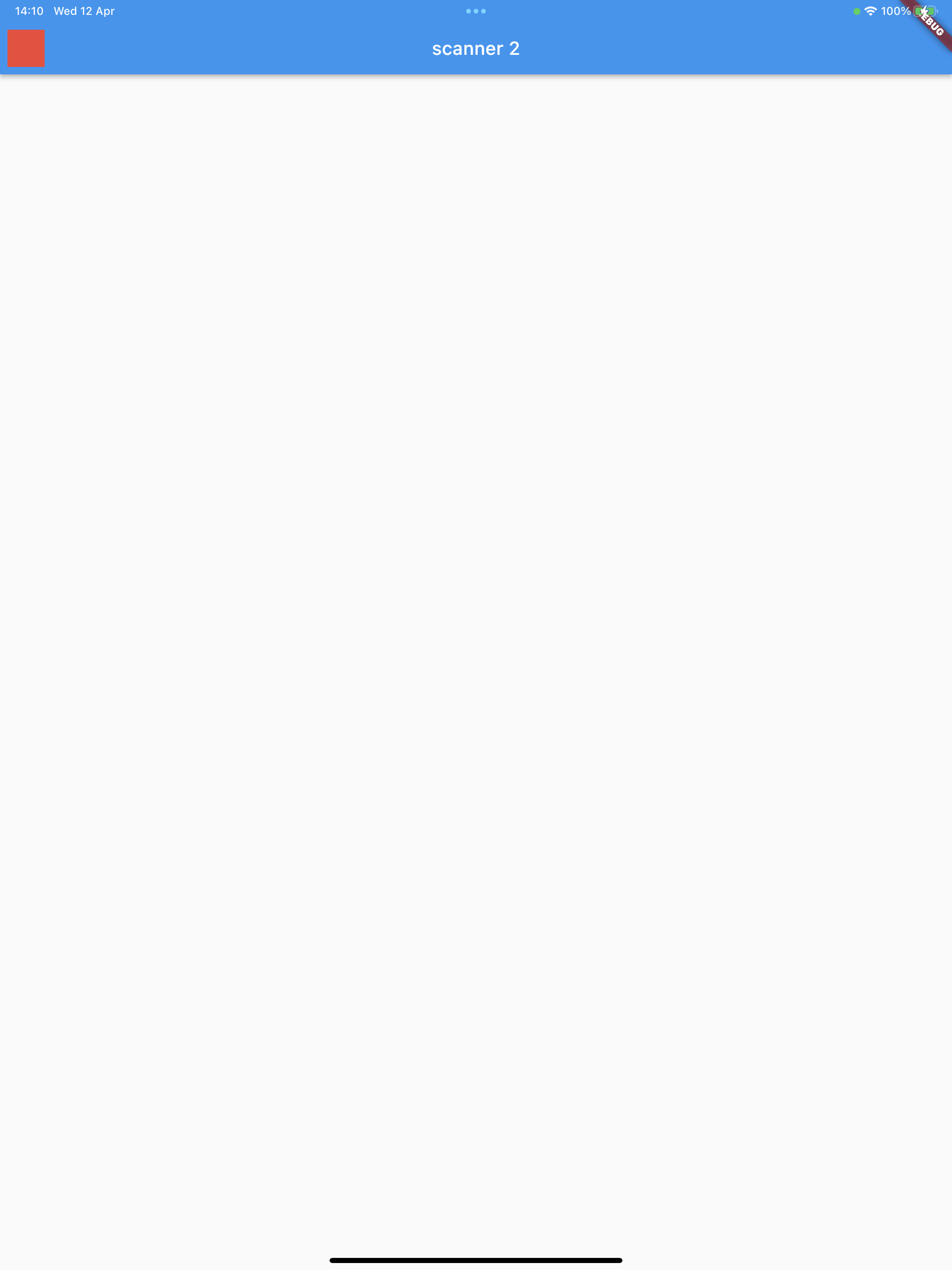
Flutter doctor:
https://user-images.githubusercontent.com/101318767/231444263-4b8b314c-9320-478c-a85c-0a1848d6ac3c.mov