Closed nvaytet closed 2 years ago
Since there has been no answers here, I'm going to close this, as it doesn't seem to be about an issue in pythreejs itself, but rather a question on how to resolve certain problems in webgl/threejs. If there is any questions/problems directly related to pythreejs, please leave a comment and I can reopen the issue.
Hi,
I am trying to create a point cloud with a custom shader that allows me to change the opacity of certain points. I am using this to render an opaque slice inside a 3d volume, surrounded by lower opacity points. While I have managed to create the custom shader, I am facing an issue with the transparency and what appears to be the order in which the points are drawn.
My code example is the following:
With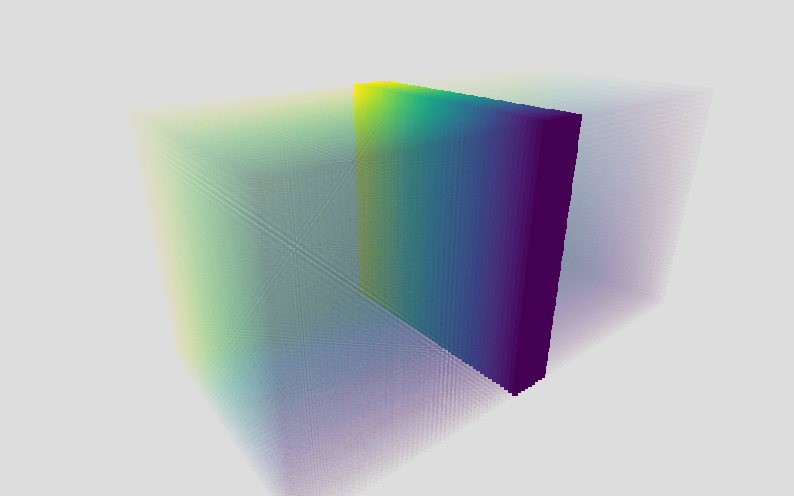
depthTest=True
in theShaderMaterial
, the scene looks good from one viewing angleBut when I move the camera to view the scene from below, the low opacity points start to block some of the high opacity points which are then no longer visible behind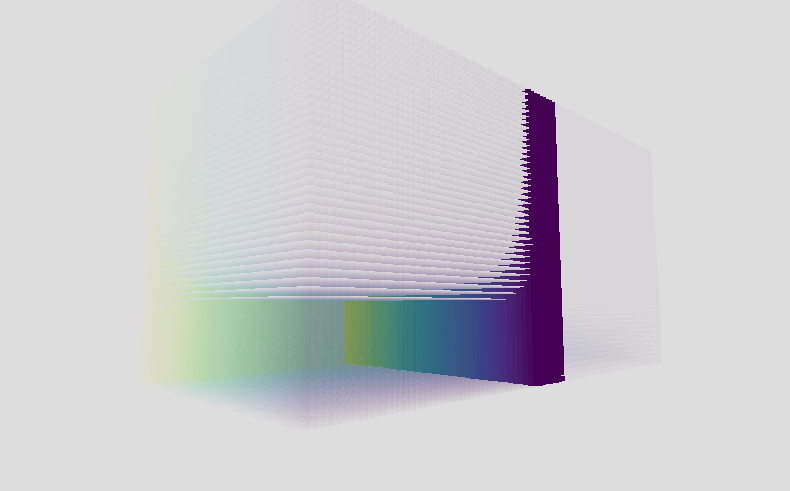
I believe this has to do with the way threejs and Webgl work, where it performs a
depthTest
and does not render points that would be hidden behind other points along the line of sight (to save on computational cost), and does not take into account transparency.So I tried to set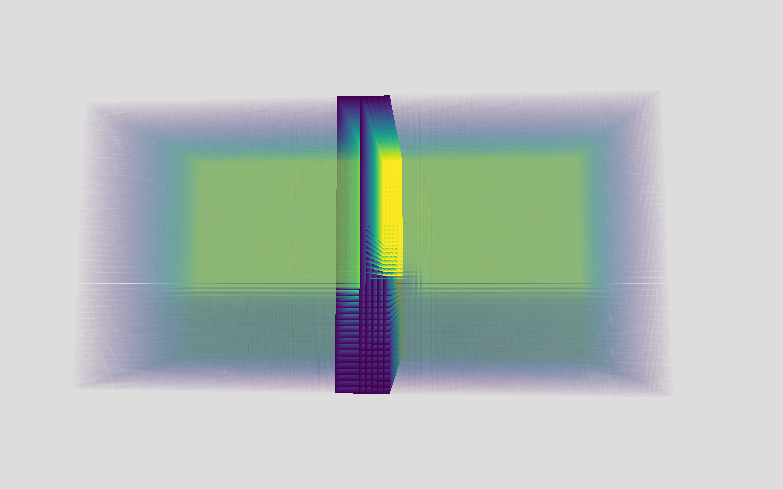
depthTest=False
, and while this generally works better with transparent points, it leads to strange artifacts that are related to the order in which the points are drawn. So in my opaque slab, depending on the viewing angle, I may be seeing some points from the back of the slab in front of other points that should be at the front. This leads to the strange 'rift' that can be seen in the centre of the slab, where I have purposefully drawn the left half of the points after the right half of the points to highlight the issue:I have tried to read up on threejs, transparency and
depthTest
but so far I have not found a fix for my problem. Many thanks for any help!