Closed rjx-ray closed 7 years ago
Can you try:
static bool p_open = true;
Thanks for the quick response, but that makes no difference
I've only used the Menubar once - here's how I did it. It's a bit different than yours so maybe it helps
ofxImGui gui;
bool showControlsWindow;
void drawMenuBar()
{
if (ImGui::BeginMainMenuBar())
{
if (ImGui::BeginMenu("File"))
{
if (ImGui::MenuItem("Quit"))
{
ofExit();
}
ImGui::EndMenu();
}
if (ImGui::BeginMenu("Windows"))
{
ImGui::MenuItem("Show Controls", NULL, &showControlsWindow);
bool doCloseAll = false;
if(ImGui::MenuItem("Close All", NULL, &doCloseAll))
{
showControlsWindow = false;
}
ImGui::EndMenu();
}
ImGui::EndMainMenuBar();
}
}
void draw()
{
gui.begin();
drawMenuBar();
if(showControlsWindow)
{
if(!ImGui::Begin("Controls Window", &showControlsWindow))
{
ImGui::End();
}else
{
ImGui::End();
}
}
gui.end();
}
The behaviour is still the same, click anywhere within the window but outside the menu and you lose focus. It must be something to do with how the event is passed
I discovered my error. I need to set ImGuiWindowFlags_MenuBar and then use BeginMenuBar instead of BeginMainMenuBar. Everything then works as expected
I'm trying to build a separate ImGui settings window for my app. I've followed the multiWindowOneAppExample which does this using ofxGui not ofxImGui
I can create a separate window with a menubar and a drop down menu like this: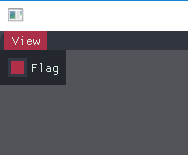
The problem is that whenever I click in the window off the menubar or the dropdown item I lose the mouse focus, the menu drop down is greyed out and I can never get it back.
I made a simple test program to illustrate the issue in app.h I have
in main.cpp I have
and in app.cpp I have
I must be missing something somewhere but I can't figure it out. Thanks for any help