// show.js
export function show(content) {
window.document.getElementById('app').innerHTML = content
};
function getAge(age) {
console.log(age)
}
var fileName1 = 'show1'
export {
fileName1 as f1,
getAge as age
}
然后打包,发现基本没变,就只改了两行:
继续,我们试试 export default
// show.js
function getAge(age) {
console.log(age)
}
export default getAge
继续上文webpack打包后代码浅析1,现在我们对
show.js
作如下修改:现在我们是用
export
直接暴露出两个方法和一个变量,我们来看看打包后的文件发生了啥变化:可以看到,变的是数组第二个元素,这里把原来传进来的第二个参数
exports
转为了__webpack_exports__
这里我们注意到,对于暴露一个变量来说,他是直接调用
__webpack_require__.d
方法,并在__webpack_exports__
上定义属性,那么为什么不直接像定义方法一样定义 变量呢? 来到__webpack_require__.d
函数,他是先做了一个 if 判断,!__webpack_require__.o(exports, name)
,判断该属性是否已经存在,,如果不存在就进行定义,这就有个问题,难道不能重复定义覆盖吗?我们做下测试:果然,打包的时候出现了一个错误:
同理,重复暴露方法也会报错:
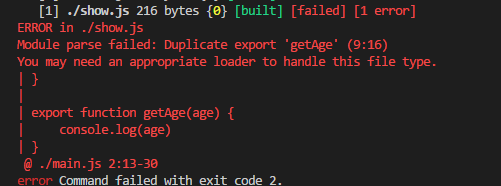
这里我还有一个疑问,就是为什么变量不能像方法一样,直接用 点的方式挂到
exports
上,而是使用defineProperty
呢?后面我又对
as
的作用做了测试:然后打包,发现基本没变,就只改了两行:
继续,我们试试
export default
前面我们都是将
export
、module.exports
、export default
分开来用,现在我们把他们结合起来用试试这样子用没啥区别,如果既
export
有export default
呢最后我们加上
module.exports
,看看有啥刺激的导出的结果: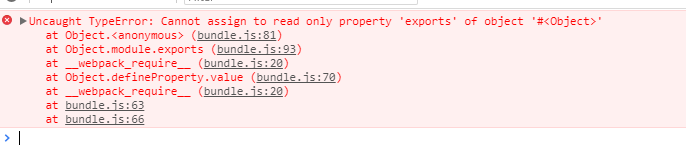
呦呵,确实我们不一样,不一样,还有,我的
getAge
呢,来分析一波:上面,在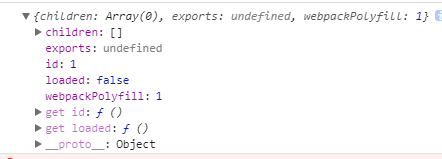
.call(__webpack_exports__, __webpack_require__(2)(module))
时,先执行__webpack_require__(2)
,执行后返回的module.exports
是一个函数,接受一个参数,在这里就是__webpack_require__(1)
模块,并返回一个新模块对象:那为什么会报错呢?因为
结果就出来了,但是依旧没有 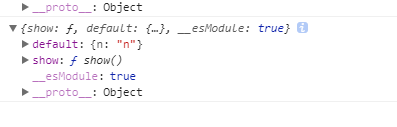
writable 当且仅当该属性的writable为true时,value才能被赋值运算符改变。默认为 false。
所以报错了,我们只要作如下改动:getAge
方法所以尽量不要这么用
突然看了下上面的,还有一种单独使用
module.exports
的情形,我们看看:好嘛,,简洁明了。
今天就到这里,我们还没有解决
浅析1
中的一些疑问,我们明天继续 ^_^