Open mutthunaveen opened 6 years ago
Hello, If I want to use this code with an old roomba, I must replace:
bool socket_1_on() {
Serial.println(socket_1_name);
Serial.println("on");
is_socket_1_on = true;
return is_socket_1_on;
}
bool socket_1_off() { Serial.println(socket_1_name); Serial.println("off"); is_socket_1_on = false; return is_socket_1_on;
FOR
bool socket_1_on() {
Serial.println(socket_1_name);
Serial.println("on");
is_socket_1_on = true;
return is_socket_1_on;
Serial.write(128); // start command
delay(50);
Serial.write(131); // safe mod
delay(50);
Serial.write(135); // clean
return true;
}
bool socket_1_off() { Serial.println(socket_1_name); Serial.println("off"); is_socket_1_on = false; return is_socket_1_on; Serial.write(128); // start command delay(50); Serial.write(131); // safe mod delay(50); Serial.write(143); // dock return false;
Is this correct? thxs
Dear bro...
i ve used your source code of multiple wemo and added android ap code for configuring wifi name pass code and also to configure switches dynamically..
here is the brief description of code i ve developed
Waits for wifi connection during attempt GPIO blinks every 1 sec untill 60sec (60sec because if power failure then my wifi router takes around 50sec to restore with good wifi (i beleive it is same in most cases))
if connection is unsuccessful after 60seconds then GPIO permanently goes off and ap mode auto starts, as shown in below figure
now take your mobile and trun on wifi and connect to the name "BUBBLES".. refer screenshot below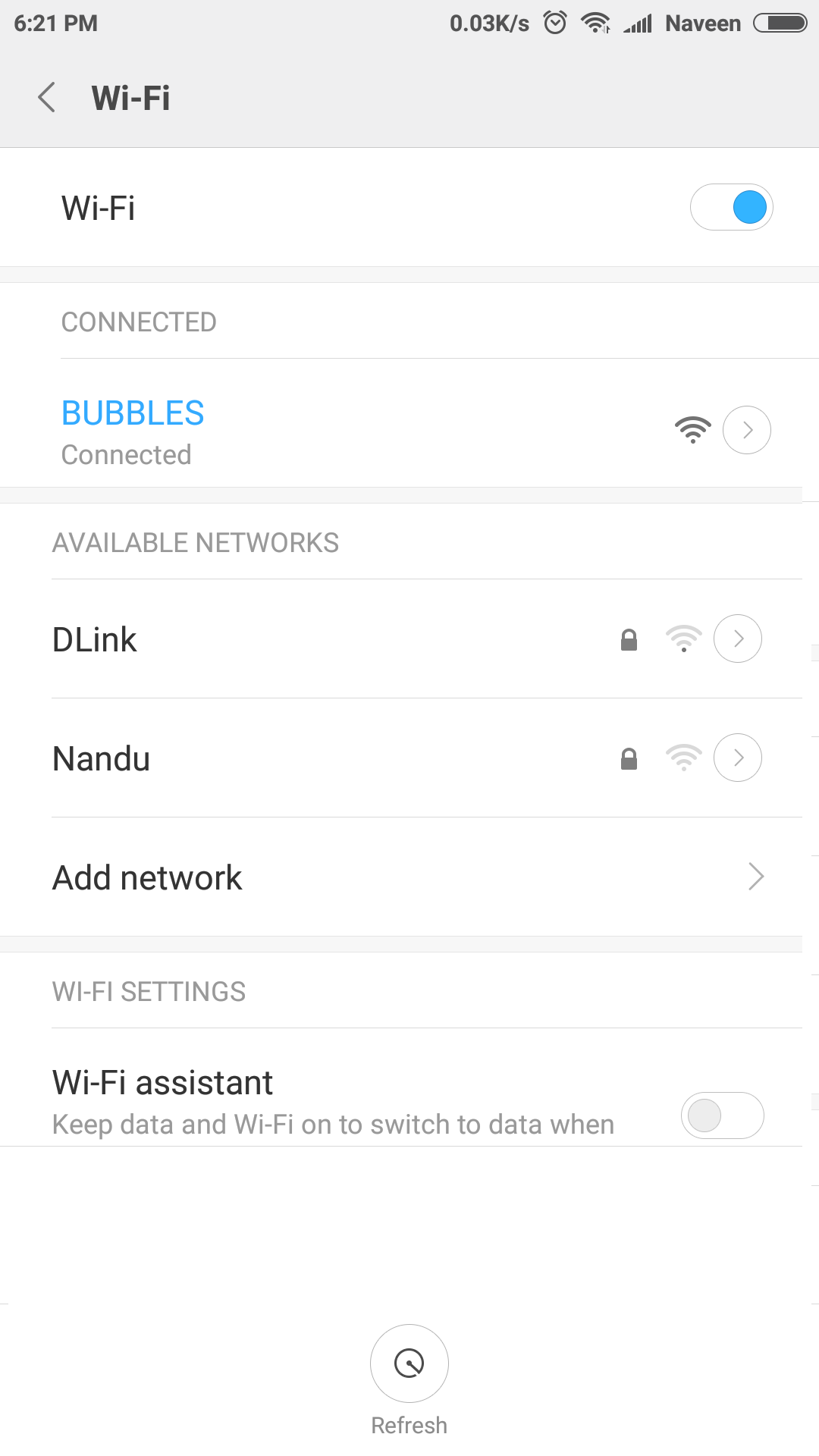
connect to ip as mentioned in 2nd step uart log. the mobile screen will look like below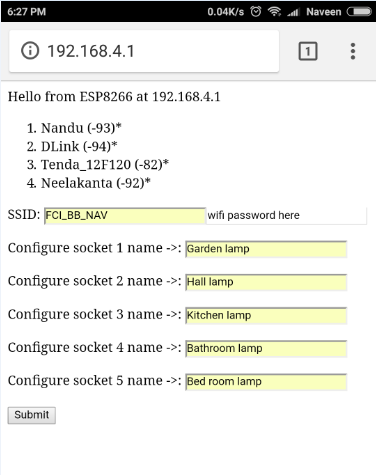
and "Submit" thats it.. wifi id and password will be saved in EEPROM and everything will work fine..
when power reset wifi will get auto connected with ID and password read from EEPROM of ESP8266. and GPIO will be on continuously when wifi is connected "symbol of successful wifi connection"
if you dont want 5 smart switches then leave blank "configure socket name text box". if you leave blanck then switch will not be configured anymore.
`
include
`
i hope the code is useful.... cheers kakopappa.... please include my code in your repositories if you are interested......