Hi, I'm trying to render Pointclouds from Depth and RGB images which are captured by the azure kinect dk.
While using the compute buffer method of the PointCloudRenderer the positions are rendered correctly but the color encoding isn't working correctly.
I took the color encoding part from the common.cginc of this project.
This is the result when using the PointCloudRendererwith the PointCloudVertexBuffer:
I've confirmed that the ColorRenderTextureis displayed correctly and the render texture format is ARGB32.
I've tried switching the channels around but no luck so far.
Hi, I'm trying to render Pointclouds from Depth and RGB images which are captured by the azure kinect dk. While using the compute buffer method of the
PointCloudRenderer
the positions are rendered correctly but the color encoding isn't working correctly.This is the compute shader I'm using:
Shader dispatch:
I took the color encoding part from the common.cginc of this project.
This is the result when using the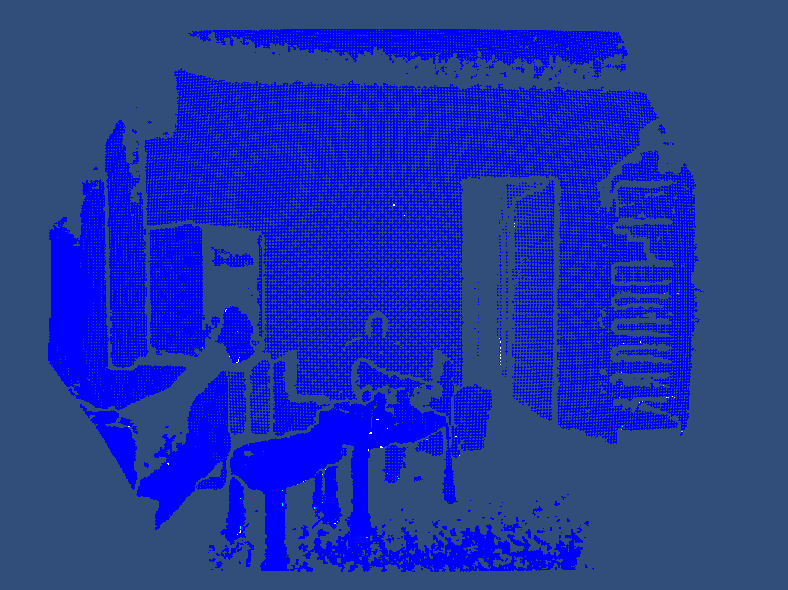
PointCloudRenderer
with thePointCloudVertexBuffer
:I've confirmed that the
ColorRenderTexture
is displayed correctly and the render texture format is ARGB32. I've tried switching the channels around but no luck so far.