import { bundleMDX } from 'mdx-bundler';
const mdxSource = `
---
title: Example Post
published: 2021-02-13
description: This is some description
---
# Wahoo
import Demo from './demo'
Here's a **neat** demo:
Adding a <= 10 to see if it breaks.
<Demo />
`.trim();
const run = async () => {
const result = await bundleMDX(mdxSource, {
files: {
'./demo.tsx': `
import * as React from 'react'
function Demo() {
return <div>Neat demo!</div>
}
export default Demo
`
}
});
const { code, frontmatter } = result;
console.log(code);
};
run();
What you did:
The line Adding a <= 10 to see if it breaks. was added to the mdx file, rest of the content is similar to the demo provided in this repo readme. I have some mdx files which have statements like these (<=, >= etc). For all the files which have these characters, the mdx-bundler fails.
I am using contentlayer which internally uses mdx-bundler and esbuild so this error was seen during that for which there is an open ticket seen here however this seems more like an bug in mdx-bundler and not contentlayer.
What happened:
The build failed for such files which have these characters mentioned above. The screenshot is what seen in contentlayer
Error text seen while running the above mentioned code with mdx-bundler's bundleMDX function directly
> _mdx_bundler_entry_point-856386d9-f2ca-417c-b32b-7adff34d061b.mdx:13:10: error: [plugin: esbuild-xdm] Unexpected character `=` (U+003D) before name, expected a character that can start a name, such as a letter, `$`, or
`_`
13 │ Adding a <= 10 to see if it breaks
╵ ^
C:\Users\AkashRajpurohit\Documents\akash\akashrajpurohit.com\node_modules\esbuild\lib\main.js:1493
let error = new Error(`${text}${summary}`);
^
Error: Build failed with 1 error:
_mdx_bundler_entry_point-856386d9-f2ca-417c-b32b-7adff34d061b.mdx:13:10: error: [plugin: esbuild-xdm] Unexpected character `=` (U+003D) before name, expected a character that can start a name, such as a letter, `$`, or `_` at failureErrorWithLog (C:\Users\AkashRajpurohit\Documents\akash\akashrajpurohit.com\node_modules\esbuild\lib\main.js:1493:15)
at C:\Users\AkashRajpurohit\Documents\akash\akashrajpurohit.com\node_modules\esbuild\lib\main.js:1151:28
at runOnEndCallbacks (C:\Users\AkashRajpurohit\Documents\akash\akashrajpurohit.com\node_modules\esbuild\lib\main.js:941:63)
at buildResponseToResult (C:\Users\AkashRajpurohit\Documents\akash\akashrajpurohit.com\node_modules\esbuild\lib\main.js:1149:7)
at C:\Users\AkashRajpurohit\Documents\akash\akashrajpurohit.com\node_modules\esbuild\lib\main.js:1258:14
at C:\Users\AkashRajpurohit\Documents\akash\akashrajpurohit.com\node_modules\esbuild\lib\main.js:629:9
at handleIncomingPacket (C:\Users\AkashRajpurohit\Documents\akash\akashrajpurohit.com\node_modules\esbuild\lib\main.js:726:9)
at Socket.readFromStdout (C:\Users\AkashRajpurohit\Documents\akash\akashrajpurohit.com\node_modules\esbuild\lib\main.js:596:7)
at Socket.emit (node:events:390:28)
at addChunk (node:internal/streams/readable:315:12) {
errors: [
{
detail: [13:11: Unexpected character `=` (U+003D) before name, expected a character that can start a name, such as a letter, `$`, or `_`] {
reason: 'Unexpected character `=` (U+003D) before name, expected a character that can start a name, such as a letter, `$`, or `_`',
line: 13,
column: 11,
source: 'micromark-extension-mdx-jsx',
ruleId: 'unexpected-character',
position: {
start: {
line: 13,
column: 11,
offset: 160,
_index: 0,
_bufferIndex: 10
},
end: { line: null, column: null }
},
fatal: true
},
location: {
column: 10,
file: '_mdx_bundler_entry_point-856386d9-f2ca-417c-b32b-7adff34d061b.mdx',
length: 1,
line: 13,
lineText: 'Adding a <= 10 to see if it breaks',
namespace: 'file',
suggestion: ''
},
notes: [],
pluginName: 'esbuild-xdm',
text: 'Unexpected character `=` (U+003D) before name, expected a character that can start a name, such as a letter, `$`, or `_`'
}
],
warnings: []
}
mdx-bundler
version: ^6.0.2node
version: 16.13.0npm
version: 8.1.0Relevant code or config
What you did: The line
Adding a <= 10 to see if it breaks.
was added to the mdx file, rest of the content is similar to the demo provided in this repo readme. I have some mdx files which have statements like these (<=, >= etc). For all the files which have these characters, the mdx-bundler fails. I am using contentlayer which internally uses mdx-bundler and esbuild so this error was seen during that for which there is an open ticket seen here however this seems more like an bug in mdx-bundler and not contentlayer.What happened: The build failed for such files which have these characters mentioned above. The screenshot is what seen in contentlayer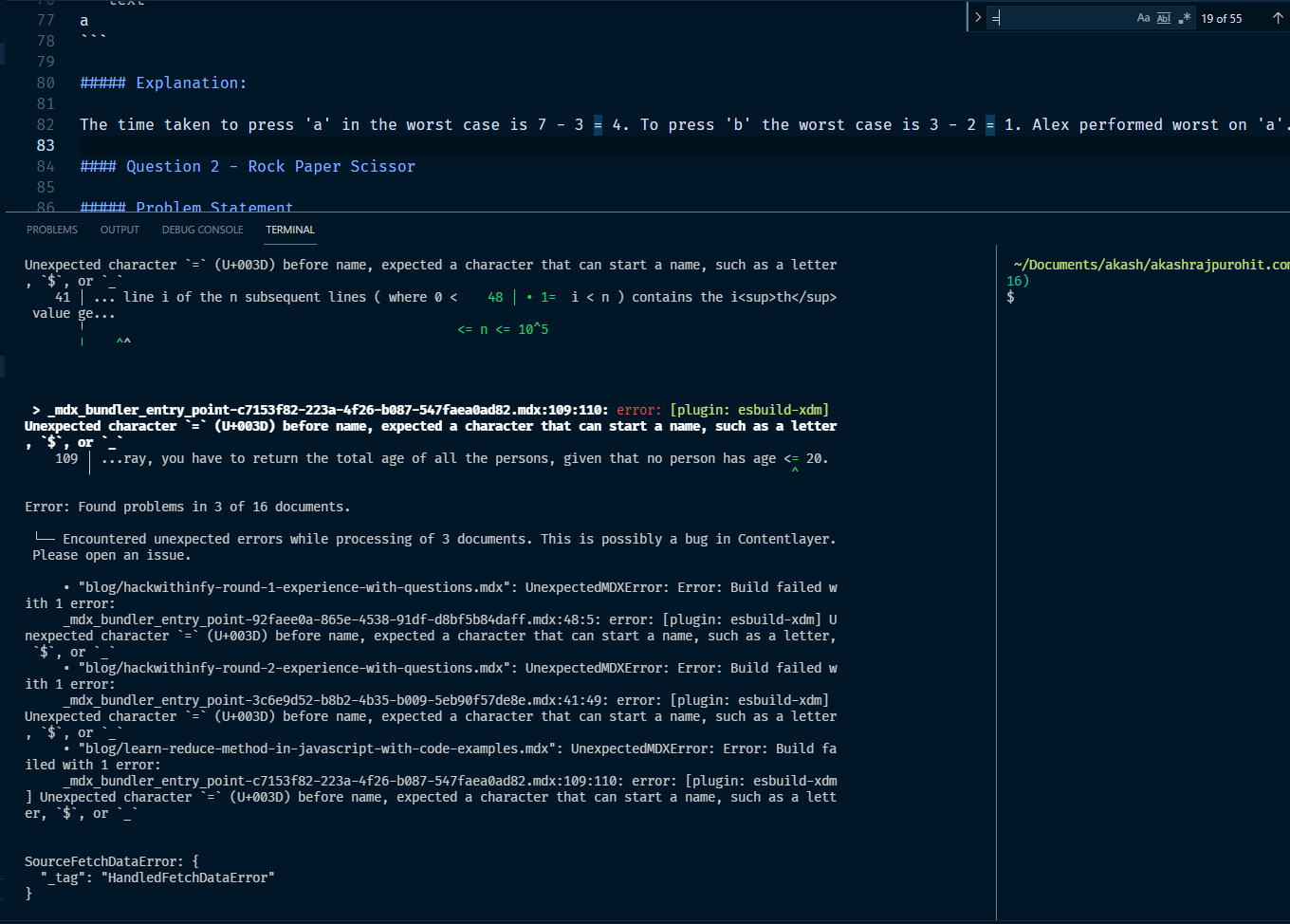
Error text seen while running the above mentioned code with mdx-bundler's
bundleMDX
function directly