Closed netlcod closed 1 year ago
This example also doesn't work as I expect https://github.com/kfrlib/kfr/issues/59#issuecomment-584579088
Hello! I solved the problem. The problem was not in the filter code, but in the signal generator code. When creating time samples: kfr::univector<kfr::fbase> time = kfr::linspace(m_last, m_last+m_batchSize*m_dt, m_batchSize, true, kfr::ctrue);
4 the argument of linspace (endpoint)should have been set to true
My task is to filter the incoming values generated in a batch. For example, the packet size is 100 samples. Figure "generated1" - visualization of the signal under test (1 Hz sine wave, sampling rate 100 Hz). Figure "generated2" - visualization of 100 packets.
generated1:
generated2:
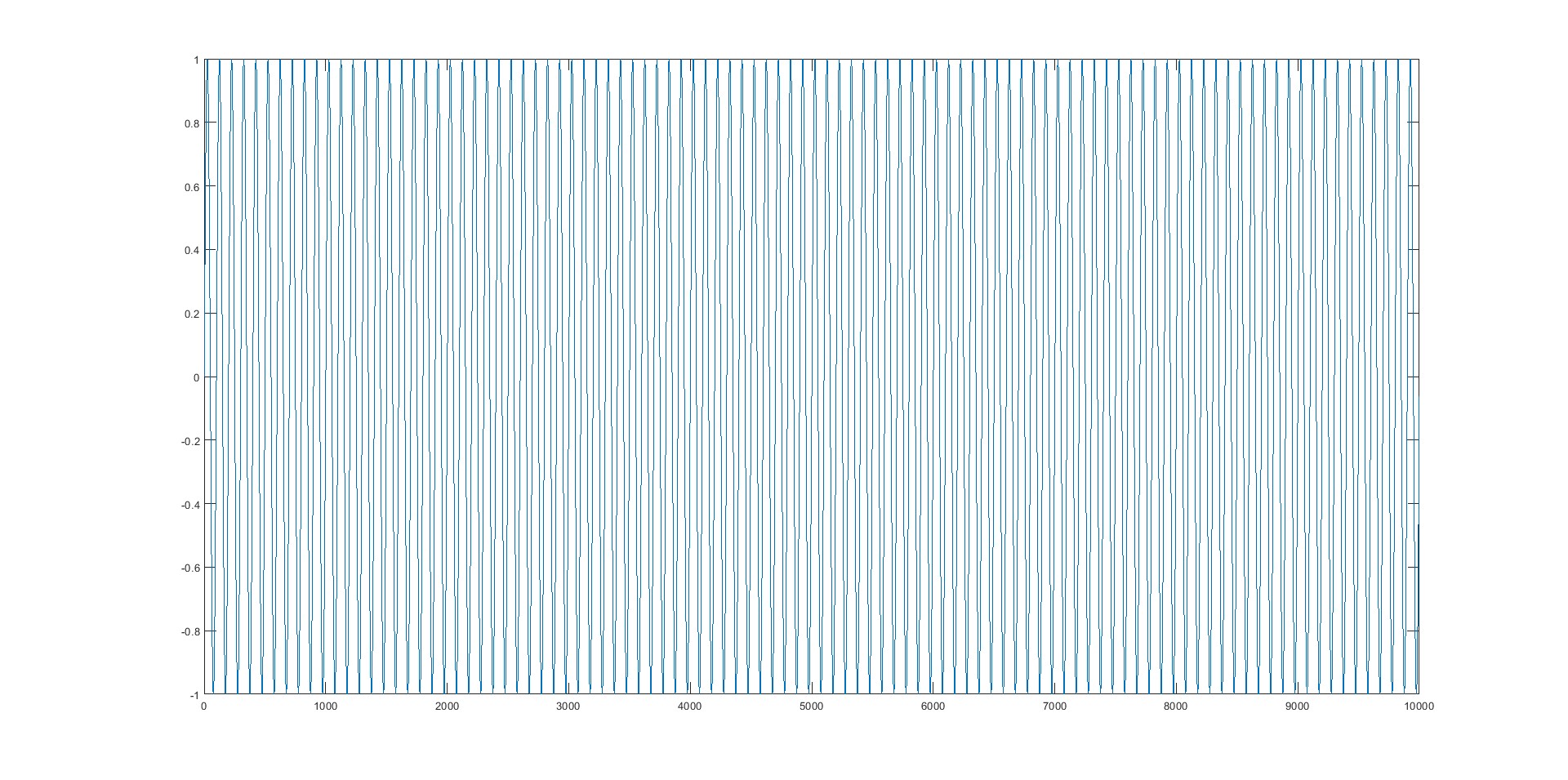
I use an IIR filter of second order (in this case a highpass filter with fc = 20 Hz). Figure "filtered1" is the filter's response to one packet. Figure "filtered2" - processing of 100 packets with the filter. The "total" picture is a cropping for several packets (the filter's response is amplified for clarity). filtered1:
filtered2:
total:
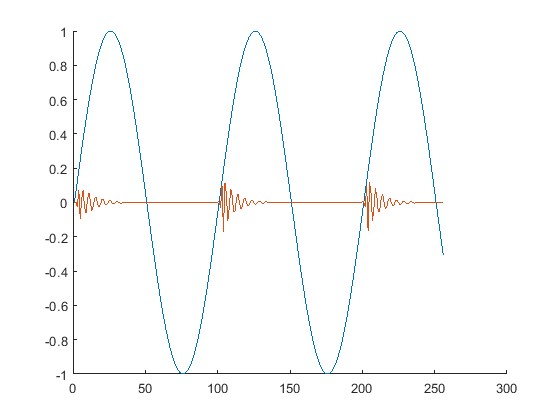
The problem is that the filter does not keep its internal state between calls when processing incoming packets. In the picture the "expected" is what I expect from my filter. So the question is how do I keep the filter state between calls? expected:
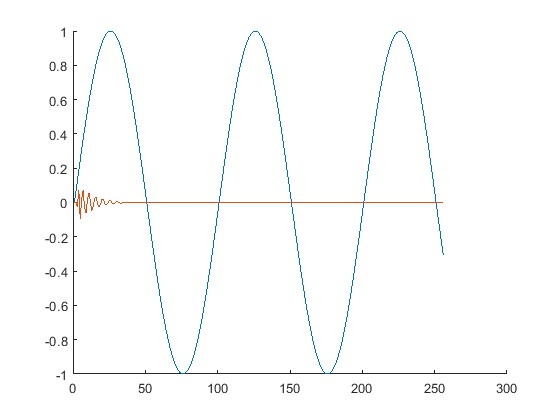
My test code is attached below.