Closed johnpierson closed 3 years ago
This was how I solved it on my end for what I was messing with.
public static string TagText(global::Revit.Elements.Element tag)
{
var internalTag = tag.InternalElement;
string internalType = internalTag.GetType().BaseType.Name;
if (internalType.Equals("SpatialElementTag"))
{
Autodesk.Revit.DB.SpatialElementTag spatialTag = internalTag as Autodesk.Revit.DB.SpatialElementTag;
return spatialTag.TagText;
}
IndependentTag independentTag = internalTag as IndependentTag;
return independentTag.TagText;
}
I was going to submit a PR, but the way the node was originally made is a bit different than how I made it.
@johnpierson I converted this into this:
[NodeCategory("Query")]
public static string TagText(Element tag)
{
if (tag == null)
throw new ArgumentNullException(nameof(tag));
switch (tag.InternalElement)
{
case Autodesk.Revit.DB.IndependentTag t:
return t.TagText;
case Autodesk.Revit.DB.SpatialElementTag st:
return st.TagText;
default:
throw new ArgumentException(nameof(tag));
}
}
Thanks for pointing this out, and sorry it took me 2 years to get to it.
Specifically, tags that inherit from SpatialElement.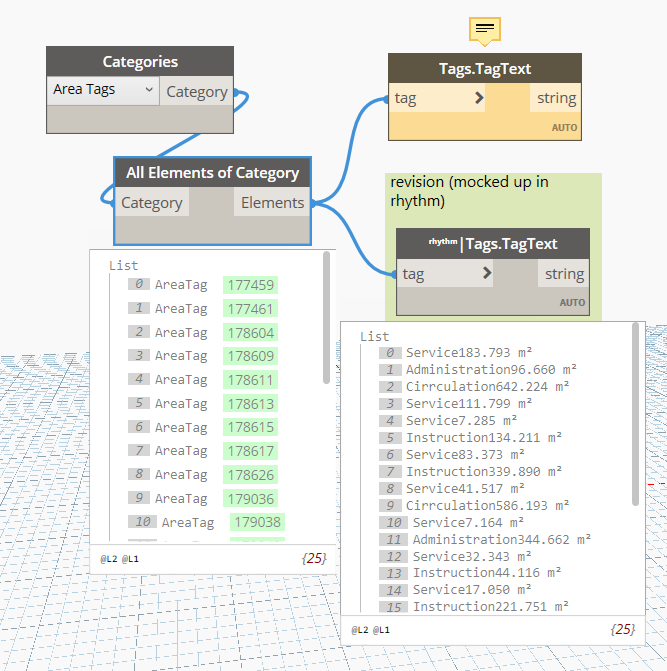