Closed jonnywilliamson closed 4 years ago
@tuktukvlad @rvzug The fix I've made is disabled for Laravel Vapor, as it would dispatch some extra jobs for existing customers. This could represent an extra amount of jobs that customers would not be expecting.
@nunomaduro Ok, I guess pending in our case is mainly "something went wrong". How can I see what went wrong, as we have no telescope-updates and also no Horizon or worker-cli to see what went wrong? The only way is the Vapor logs in the vapor backend?
We have the same issue with Vapor and Laravel 9.
I also have this problem, but because of the wrong time zone in config.
@c0ntr-all Could you expand on that? What do you mean by a wrong timezone in the config. Surely all timezones are valid, no matter which one is used?
Still happening, Laravel 11.9, telescope 5.2
@themsaid
So I have seen the previous issues related to this https://github.com/laravel/telescope/issues/507 https://github.com/laravel/telescope/issues/503 https://github.com/laravel/telescope/issues/411
And I'm suffering the same problem. Some of my jobs are reporting as PENDING even though I know they have completed successfully, and also HORIZON shows the same job as being done correctly.
I've spent a lot of time trying to recreate this using as minimal amount of code as possible, and I hope I've succeeded in providing you with an example that will replicate on your system.
Very quickly as an overall picture.
Here's some code.
I have started horizon, and ensured that it is processing jobs from "default" and "broadcast" queues.
Now when I start the whole process job (hitting "/test" in the browser) I'll get the following:
But in horizon it's perfect: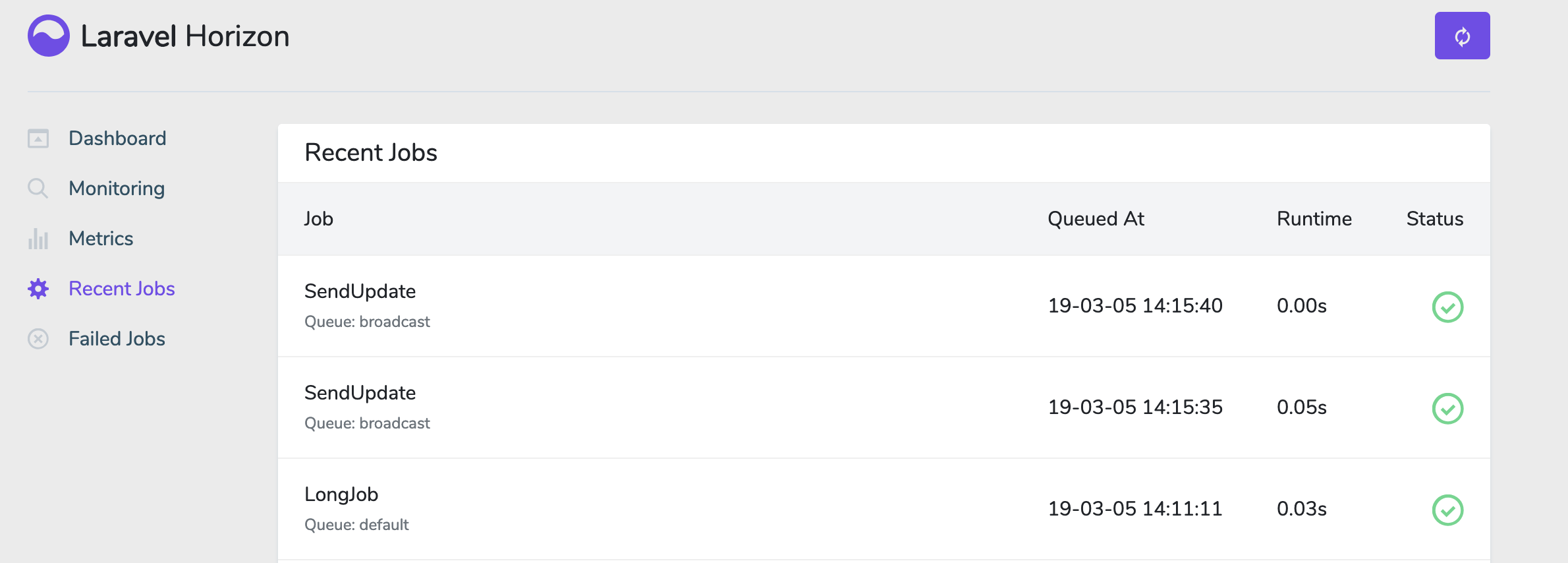
The next post is some info on my debugging attempts.