Closed DeafMan1983 closed 1 year ago
Please provide a full backtrace, such that we can see the values of various symbols. Make sure to build with debug information, and install the debug symbols of at least SDL2. That way, gdb is able to show symbol and function names instead of hexadecimal values.
I found bug cause SDL_Surface shouldn't get private void *value with operator implicit and explicit. It works fine now. It was gone crash dumped. I have checked SDL2-CS by @flibitijibibo = OK! Now I need update my DeafMan1983.Interop.SDL3
Thanks for helping me! I expect @flibitijibibo is great C# developer for SDL2 and I am also C# developer for SDL3 :)
Hello everyone,
I have made test app ( test.cpp ) and it works fine for testing. But in C# crashes as SDL_MapRGB_REAL or SDL_MapRGBA_REAL shows in gdb debbugging crash message.
And I use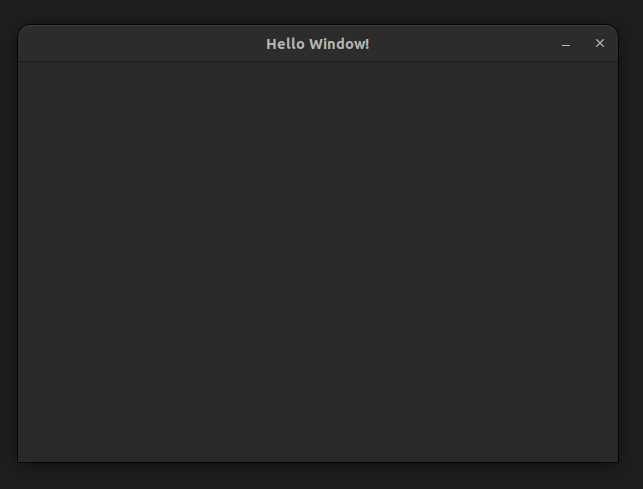
g++ -o test_app test.cpp -lSDL3
and run with./test_app
The result looks great.And I try with C# in DeafMan1983.Interop.SDL3 ( I forget to add defines... I will add soon... ) But I am working hard with SDL3 in C# I have checked
I don't understand why does SDL shared has 2 REAL methods from
SDL_MapRGB()
andSDL_MapRGBA()
with valid p/invoke viaDllImport()
I have verified with ClangSharpPInvokeGenerator. And they are valid. I have checked with objdump and objdump shows valid public methods but why do they need to useSDL_MapRGB_REAL
orSDL_MapRGBA_REAL
SDL_MapRGB()
orSDL_MapRGBA()
I try out in C#
I prompt with dotnet:
dotnet build -c Release
anddotnet run -c Release
And dotnet app runs out cause it hassegment core dump
I have verified gdb with dotnet app and It shows.Or I have tried without gdb:
./01_HelloWindow
then it shows crash message.That is crash because segment fault has thrown.
Please fix both
SDL_MapRGB()
andSDL_MapRGBA()