Open Sherlock-Holo opened 2 years ago
by the way I am using the master branch
i would like to know why, but this works:
Flex::column().with_child(button).with_default_spacer().with_flex_child(list, 1.)
I believe this is because when using Flex.with_child
the child widget has infinite height. with_flex_child
restricts the height to the "viewport"(?).
I want to scroll the list, thats my codes
it can scroll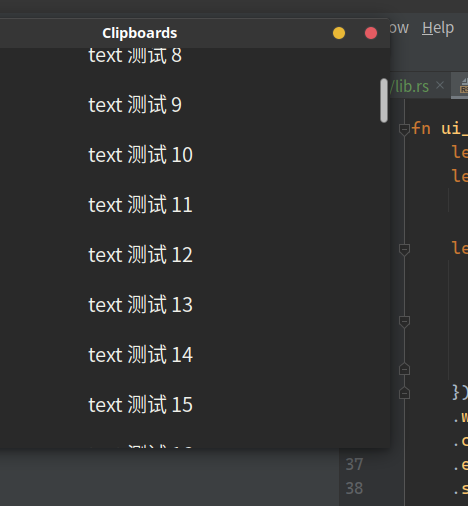
and now I want to add a button on the top
but now the list can't scroll and the scroll bar is miss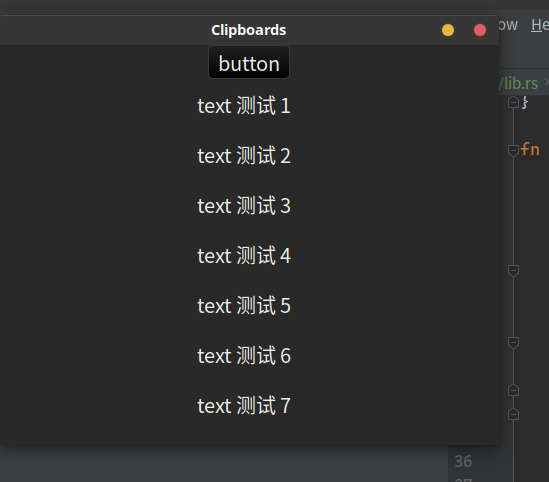
how should I do to add the button on the top and keep the list scrollable?