Describe the bug
I'm trying to build on the example app provided, so far I've implemented everything like the example app and I've been successful with connecting to a room on example website, I recieve audio from website, but I don't read the video stream, and I also can't send audio or video at all.
To Reproduce
Steps to reproduce the behavior:
add the following to index.js
import { registerRootComponent } from "expo";
import { registerGlobals } from "livekit-react-native";
import App from "./App";
registerRootComponent(App);
registerGlobals();
Describe the bug I'm trying to build on the example app provided, so far I've implemented everything like the example app and I've been successful with connecting to a room on example website, I recieve audio from website, but I don't read the video stream, and I also can't send audio or video at all.
To Reproduce Steps to reproduce the behavior:
index.js
App.tsx
the components used here are mostly the same as what's in the example, I've removed the screensharing logic and the messages
await room.localParticipant.enableCameraAndMicrophone();
in theuseEffect
, I get the following error:Expected behavior This should both receive & send video and audio streams between the two clients
Screenshots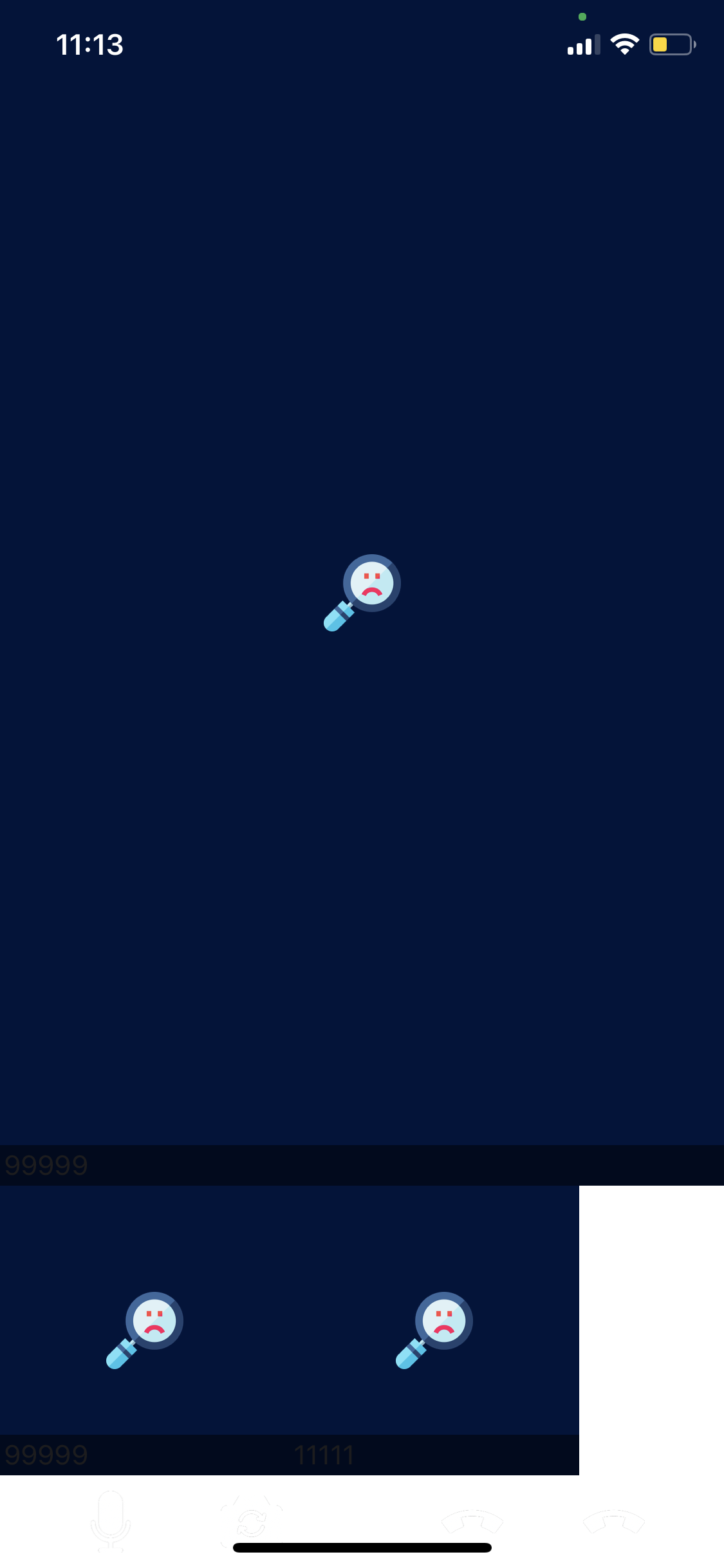
Device Info (please complete the following information):
Additional context Normal peer to peer video calling using react-native-webrtc is working fine, so the issue isn't in any native setup of webrtc