Open j0sh opened 5 years ago
I have been able to verify this by ear - there is a noticeable "blip" sound every 2 seconds.
Listen to this test stream with good speakers / headphones.
ffplay http://52.29.226.43:8935/stream/hello_world/P144p30fps16x9.m3u8
Does anyone else hear what I hear?
When transcoding audio with consecutive segments, harmonic content such as sine waves produces audible clicking when playing back transcoded results. This clicking shows up as a transient in spectrograms. Presumably this is because most audio encoders operate with a sliding window, which leads to discontinuities at the edges of each group of samples.
Mitigation
One way to mitigate this effect is to pad the source segment with audio samples from adjacent segments. After transcoding, the padded samples can be dropped. However, it is possible that the efficiency of this depends on a few factors:
This may have other implications in a live transcoding context, such as the need to wait for trailing padding to become available before submitting the source segment for transcoding.
Example of padding the end of a segment:
Example of trimming the padding:
Samples
Source:
ffmpeg -f lavfi -i sine -c:a aac -f hls -hls_time 2 -t 10 test.m3u8
Source audio, concatenated:
Sample program to transcode audio:
Transcoded audio, concatenated: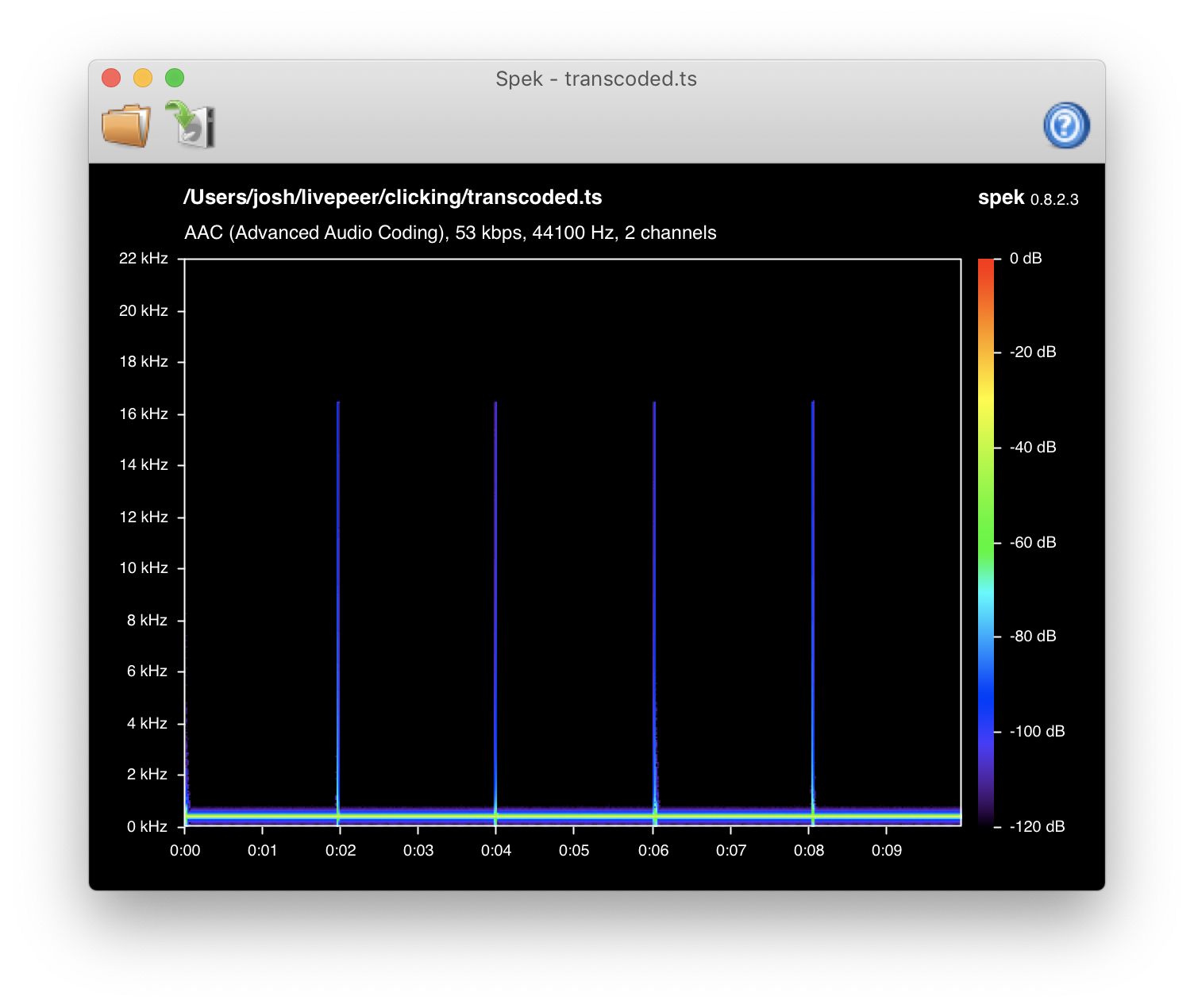