Closed dbartenstein closed 1 year ago
@dbartenstein here is working example:
import logging
import os
from logdna import LogDNAHandler
log = logging.getLogger('logdna')
key = os.environ['LOGDNA_INGESTION_KEY']
options = { 'custom_fields': 'meta' }
custom = LogDNAHandler(key, options)
log.addHandler(custom)
log.setLevel(logging.INFO)
log.warning('7.7%', extra={'meta': {'user': 5698}})
This line is critical in your case:
options = { 'custom_fields': 'meta' }
You are right - the initial error was caused by missing 'extra='. We will fix the doc. Thanks.
@dbartenstein here is working example:
import logging import os from logdna import LogDNAHandler log = logging.getLogger('logdna') key = os.environ['LOGDNA_INGESTION_KEY'] options = { 'custom_fields': 'meta' } custom = LogDNAHandler(key, options) log.addHandler(custom) log.setLevel(logging.INFO) log.warning('7.7%', extra={'meta': {'user': 5698}})
This line is critical in your case:
options = { 'custom_fields': 'meta' }
You are right - the initial error was caused by missing 'extra='. We will fix the doc. Thanks.
@dkhokhlov thanks Dmitri! That works! Please update the doc accordingly and close this alleged issue.
From what I see in the docs, the meta parameter is passed to the log method using
*args
:log.warning("Warning message", {'app': 'bloop'})
https://docs.python.org/3/library/logging.html#logging.debugWith the following code fragment we run into a nasty error as it tries to do a substitution of the message:
Looking at the Python logging documentation, the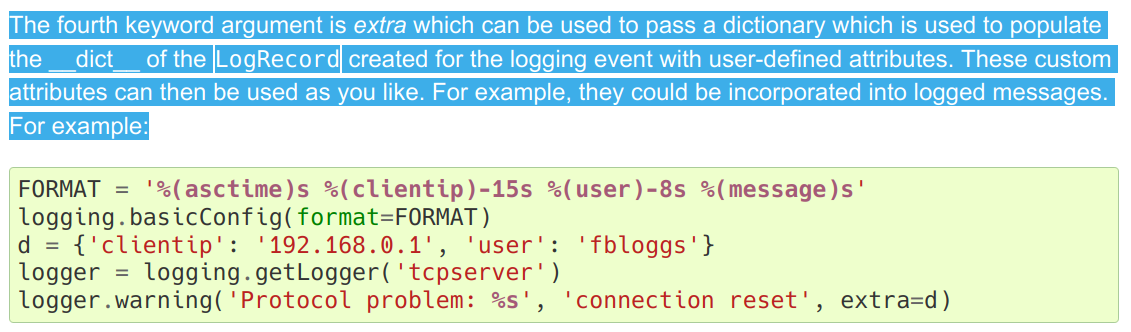
extra
parameter seems to be the proper way of doing it: https://docs.python.org/3/library/logging.html#logging.Logger.debugThe way forward
logging.warning('7.7%', extra={'meta': {'user': 5698}})
currently does not send the meta data to LogDNA.That should be changed of course. Python libraries for other logging services do it exactly like that: https://docs.logtail.com/integrations/python