I've been having issues with displaying PDFs on iOS.
When entering the PDF display screen, the first few pages are displayed correctly. When advancing the pages, they begin to show file rendering failures, displaying glitch's and the screen is blank, as shown in the gif below.
I tried to reduce the size of the PDF to 1280x720 pixels (good quality - as small as possible), and this increased the amount of pages to be rendered without the error, but around page 13, the rendering error starts again.
The PDF was created by Keynote software (Apple - Mac OS), similar to PowerPoint.
Other tests performed:
1 - I tried to change it to a PDF I found on the internet, with 200 pages, and there was no problem when opening.
3 - I found that it only occurs on iOS on physical cell phones. In debug/emulation, the bug does not occur. On the physical phone, the app doesn't close due to the bug, so I don't have any error logs.
I use Firebase to store the PDFs files in my Flutter app.
I've been having issues with displaying PDFs on iOS. When entering the PDF display screen, the first few pages are displayed correctly. When advancing the pages, they begin to show file rendering failures, displaying glitch's and the screen is blank, as shown in the gif below. I tried to reduce the size of the PDF to 1280x720 pixels (good quality - as small as possible), and this increased the amount of pages to be rendered without the error, but around page 13, the rendering error starts again.
The PDF was created by Keynote software (Apple - Mac OS), similar to PowerPoint.
Other tests performed:
1 - I tried to change it to a PDF I found on the internet, with 200 pages, and there was no problem when opening.
2 - I used the website https://www.ilovepdf.com/compress_pdf to compress the PDF, but it didn't solve the problem either.
3 - I found that it only occurs on iOS on physical cell phones. In debug/emulation, the bug does not occur. On the physical phone, the app doesn't close due to the bug, so I don't have any error logs.
I use Firebase to store the PDFs files in my Flutter app.
Gif showing the bug - from page 13.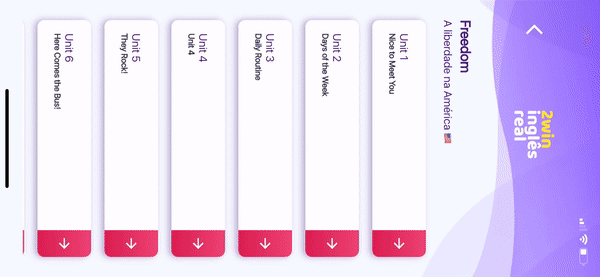
Here's the code: