Open jonagoldman opened 1 year ago
+1 for the usage of version numbers. :D
Same results when using a regular Laravel command:
class RegisterUser extends Command
{
protected $signature = 'register:user';
protected $description = 'Register a new user.';
public function handle()
{
$attributes = [];
$attributes['name'] = $this->ask('Name?', 'User 1');
$attributes['email'] = $this->ask('Email?', 'user-1@godesk.wip');
$attributes['password'] = $this->secret('Password?');
$attributes['password_confirmation'] = $this->secret('Password Confirmation?');
// small tweak to set the attributes in a request so I can use them in the action
$request = request()->merge($attributes);
$action = app(RegisterUserAction::class);
$user = $action->handle($attributes);
$this->info('User registered!');
}
}
I guess the console exception handler is not prepared for this types of exceptions...
Hi, I'm using v2.5.1 of this package with Laravel v10.1.3.
To ensure data always gets validated no matter how the action is called, I'm using unified attributes to manually validate directly in the
handle()
method. This works fine when the action is running as a controller, so if when the validation fails, the exception is handled correctly, but when the validation fails running as a command it is not handled.Full example:
Console result: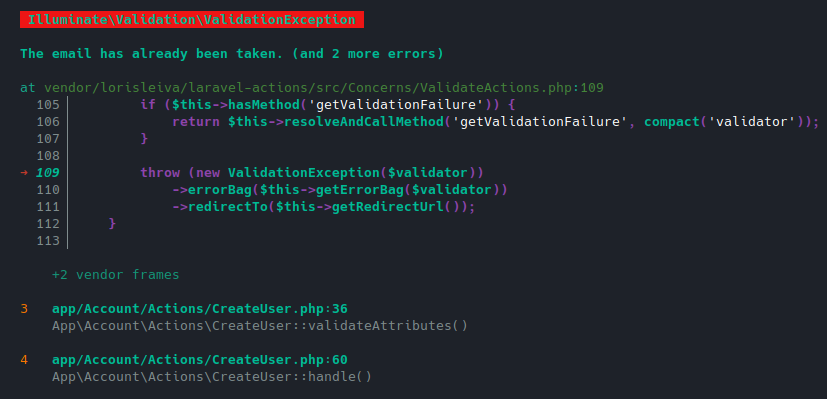
I can catch the exception locally inside the
asCommand()
method:But I wonder if there is a better, cleaner way, and I don't have to do this on every action. I don't know if it needs to be handled inside the package or in the app itself.
Thanks.