Closed thintn222 closed 2 years ago
For updating, I'm pretty sure the error is about the hard constraints BC since the PDE does not satisfy the dirichletBC even I put lots of weight in there. How can I set the Dirichlet BC for this case? For example, T(x, y=0) = 1. I read the Q&A. However, these problems are about, i.e., y(0) = 0, not 1. Thank you, Lu Lu, again for the amazing library.
Yes, the hard constraint above is correct for T(x,y=0)=1.
Hi @lululxvi, I'm sorry for bothering you again.
As your suggestion, since the PINN does not satisfy the BC, I use the hard constraint as
net.apply_output_transform(lambda x, T: x[:, 1:2] * T + T_AB #T_AB = 1
However, the results are still completely off, and the RobinBC loss does not converge even the PDE loss converge well to 1e-4
I tried using loss weights to put more weight in the RobinBC, but it still cannot converge. All the data and geometry also have been scaled to 0-1. Do you have any suggestions that I can try out? Thank you so much for an amazing library <3
To verify your code, you can also use the exact solution as the training data, see the examples of inverse problems. If the loss still cannot do down, then it is highly possible that there might be some issue in the code.
Another suggestion is that you may first try a 1D problem.
Dear @lululxvi, Thank you for the great and useful library that can help a beginner like me approaching the PINNs techniques. Recently, I start investigating a heat transfer problem that consists of 2 convective boundary conditions (RobinBC) and 1 Dirichlet BC. Here is the domain
The PDE and RobinBC are shown, respectively, as
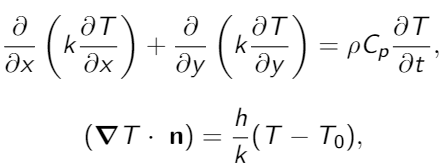
First, to test the algorithm, I use only one convection BC (Robin one) one the upper edge CD. The result shows a good prediction with the temperature increases linearly from the bottom to the upper. This really makes sense. However, when I do the real problem with 2 convective BC (the right and upper edges), the results look extremely off as shown below, which the bottom edge seems not to hold the temperature during the simulation even I use Dirichlet in there.
Here is the code for implementing the algorithm
The training and testing are not converged well even I use the loss_weights option. I read all of the Q&A to get some ideas however the problem still existed. One more notice that I tried to use the model to predict the material point in the bottom edges only and see that they are not always equal to the reference value as the Dirichlet should do. Do you think the hard constraints BC will work in this problem?. Is it the right expression for the DirichletBC hard constraints in my case?
net.apply_output_transform(lambda x, T: x[:, 1:2] * T + T_AB
Once again, thank you so much for the useful library DeepXDE. Do you have any suggestions that I can try out?
Thank you very much.