Open SongPapers opened 2 years ago
PS: This problem does not occur if p is set as the output of the network ( inputs: x,t, outputs: A, u, p ).
When defining "eq3 = p - k*((A/Ae)*(1/2)-1) ", the net will work, while net cannot work if "eq3 = p - k(tf.sqrt(A/Ae) -1) ".
You need to make sure A/Ae > 0.
You need to make sure A/Ae > 0.
Oh my God, To be so involved with the result that forget the most important details. Thx~
You need to make sure A/Ae > 0.
Oh my God, To be so involved with the result that forget the most important details. Thx~
Hi, Would you like to tell me how you did in order to make sure A/Ae>0?
Hi, you could use function tf.sign to make sure A/Ae>0
Hi Dr.Lu, Thank you very much for sharing and answering. I am trying to simulate a system of equations and solve it by DeepXDE. However I meet a problem. In order to briefly, I give the picture and the code.
A set of equations are given by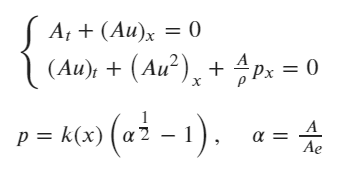
The input variables are x and t as well as the outputs are A and u. Ae = 1, k(x) = 1.
Then I set up a network.
But the loss function has nan cases:
The problem is the second loss function and the corresponding code is " eq2 = Au_t + Au2_x + p_xA/rho ". Further, the problem is " p = k(tf.sqrt(A/Ae)-1) ". I find that nan does not appear if tf.sqrt() (same as **(1/2) ) is removed.
I don't understand why? Looking forward to your answer~