I set the top boundary (the parametrized one) as follows:
def boundary_top(X, on_boundary):
# The top boundary is applied at all points on X[2] i.e. np.isclose(param_geo, param_geo)
param_geo = X[2] # X[:, 1:2] is equivalent to X[:,1]
# third dim is the parameter L
return on_boundary and np.isclose(param_geo, param_geo) # when y = 1
The PDE loss reduces the residual as follows:
def pde(X,u):
dy_xx = dde.grad.hessian(u, X, i=0, j=0) # x from -0.5 to 0.5
dy_yy = dde.grad.hessian(u, X, i=1, j=1) # y from 0 to 10.0
return dy_xx + dy_yy
I set num_domain = 25000 and num_boundary = 10000.
This solution looks good.
I do not think this is the correct way. The geometry should be variable like this
training_dataset = np.array([]) # the training dataset
L = np.linspace(1.0,10.0,1000) # the 3rd input
x = np.linspace(-0.5, 0.5, 512) # x coordinates
for length_parameter in L: # for each length parameter
# y is used to satisfy the PDE i.e the collocation points
y = np.linspace(0, length_parameter, 1000) # y coodinates should be less the length_parameter i.e. inside the variable upper boundary
mesh_x, mesh_y = np.meshgrid(x, y, indexing="ij") # create x,y meshgrid
# extracting individual x, y coordinates
x_temp = np.expand_dims(mesh_x.flatten(), axis=-1)
y_temp = np.expand_dims(mesh_y.flatten(), axis=-1)
# L contains the same coordinate for the entire length of x,y coordinates
L_temp = np.ones_like(y_temp)*length_parameter
# stacking the (x,y,L) coordinates to the training dataset.
temp_dataset = np.hstack([x_temp, y_temp, L_temp])
training_dataset = np.vstack([training_dataset, temp_dataset]) if training_dataset.size else temp_dataset
I am solving a 2D steady-state heat conduction problem as follows:
Here, I have parametrized the upper limit of y-boundary condition. For this, I introduced another input in the network i.e.
u(x,y,L)
.I set the top boundary (the parametrized one) as follows:
The PDE loss reduces the residual as follows:
I set
num_domain = 25000
andnum_boundary = 10000
.This solution looks good.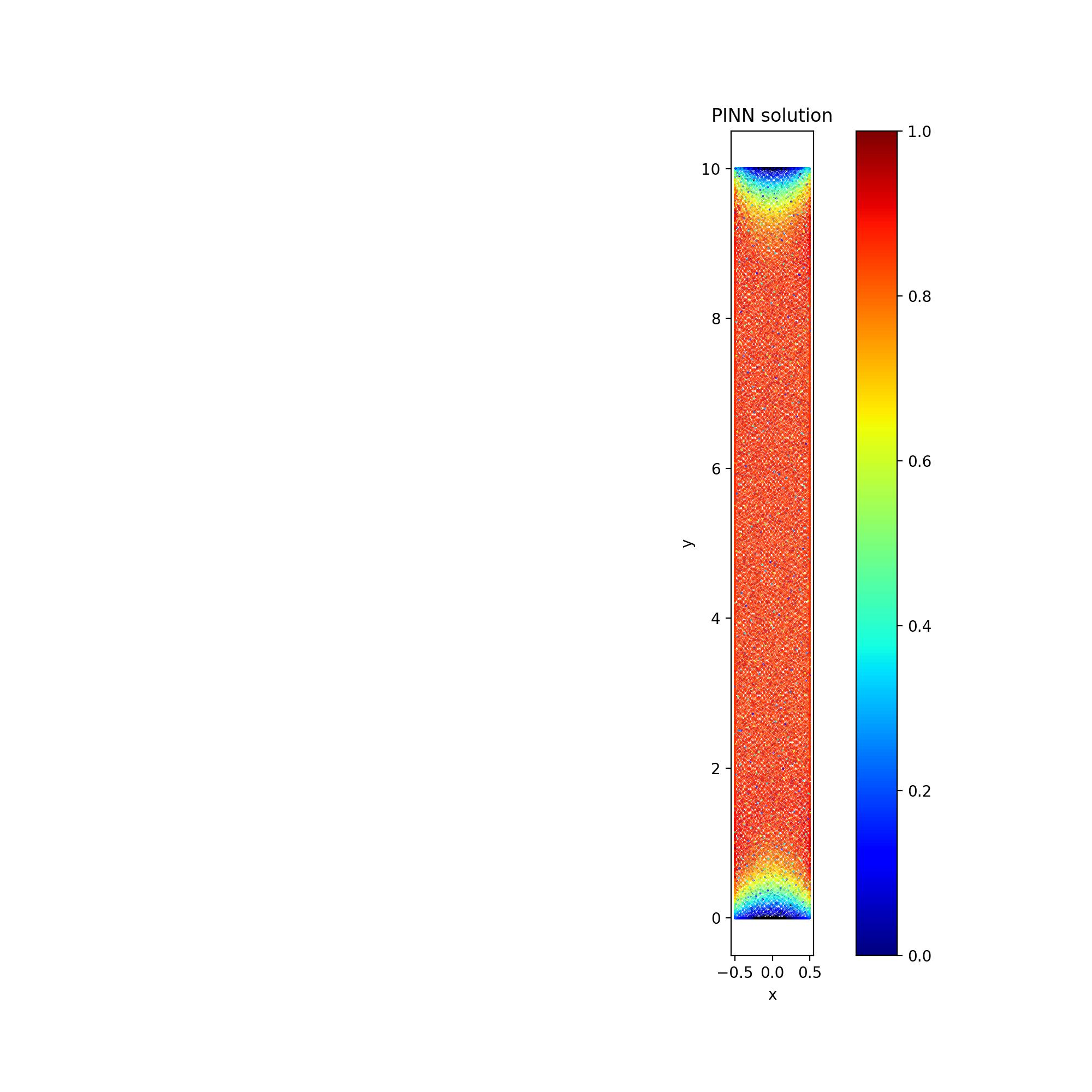
I do not think this is the correct way. The geometry should be variable like this
How to implement a sampling like this in DeepXDE?