Closed Kimbsen closed 3 years ago
To control the layout, your CustomButton
will have to implement the CreateLayoutItem
method of the walk.Widget
interface. The code below is very similar to that of walk.Button
and should get you going:
func (cb *CustomButton) idealSize() walk.Size {
canvas, err := cb.CreateCanvas()
if err != nil {
return walk.Size{}
}
defer canvas.Dispose()
bounds, _, _ := canvas.MeasureTextPixels(/* TODO: Insert appropriate args here. */)
// TODO: Adjust size if required.
return bounds.Size()
}
func (cb *CustomButton) CreateLayoutItem(ctx *walk.LayoutContext) walk.LayoutItem {
return &customButtonLayoutItem{
idealSize: cb.idealSize(),
}
}
type customButtonLayoutItem struct {
walk.LayoutItemBase
idealSize walk.Size
}
func (li *customButtonLayoutItem) LayoutFlags() walk.LayoutFlags {
return 0
}
func (li *customButtonLayoutItem) IdealSize() walk.Size {
return li.MinSize()
}
func (li *customButtonLayoutItem) MinSize() walk.Size {
return li.idealSize
}
For the declarative part, you could have a decarative
sub package and do something like this:
package declarative
import (
"github.com/lxn/walk"
. "github.com/lxn/walk/declarative"
)
type CustomButton struct {
// Window
Background Brush
ContextMenuItems []MenuItem
Enabled Property
Font Font
MaxSize Size
MinSize Size
Name string
OnBoundsChanged walk.EventHandler
OnKeyDown walk.KeyEventHandler
OnKeyPress walk.KeyEventHandler
OnKeyUp walk.KeyEventHandler
OnMouseDown walk.MouseEventHandler
OnMouseMove walk.MouseEventHandler
OnMouseUp walk.MouseEventHandler
OnSizeChanged walk.EventHandler
Persistent bool
RightToLeftReading bool
ToolTipText Property
Visible Property
// Widget
AlwaysConsumeSpace bool
Column int
ColumnSpan int
GraphicsEffects []walk.WidgetGraphicsEffect
Row int
RowSpan int
StretchFactor int
// ImageView
Image Property
Margin Property
Mode ImageViewMode
// CustomButton
AssignTo **yourpackage.CustomButton
OnClicked walk.EventHandler
// TODO: Put additional CustomButton fields here.
}
func (cb CustomButton) Create(builder *Builder) error {
w, err := yourpackage.CustomButton(builder.Parent())
if err != nil {
return err
}
if cb.AssignTo != nil {
*cb.AssignTo = w
}
return builder.InitWidget(cb, w, func() error {
// TODO: Do additional initialization here.
if cb.OnClicked != nil {
w.Clicked().Attach(cb.OnClicked)
}
return nil
})
}
Thanks, i got it working using a custom layout item!
I'm having some trouble making a custom pushbutton. ( i need to color it and have nice rounded edges :/ )...
In any case i have a working button but i'm struggeling to keep it from expanding and taking all availiable space in the layout. I've tried using spacers to take the extra space but it doesn't work. I've used spacers like that before with Qt, but at this point i'm sure i'm misunderstanding layouts, spacers etc somewhat.
The code i have is modified from the drawing example:
main.go
button.go
This ends up looking like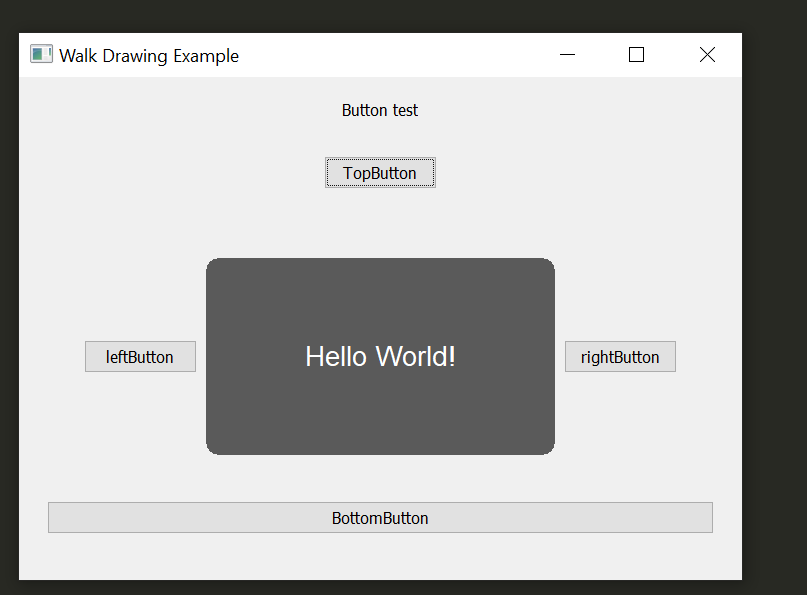
The button will expand in size as i increase the window size. Or shrink to smaller than its "boundry" if i make the window smaller.
What i would like to achieve is to have it stay the minimum size it needs to render the text properly when the window grows. And ideally not shrink so the text becomes cropped. Like TopButton in the example. It has one spacer on each side and they take the space on each side.
Any ideas?