Open yuriescl opened 3 years ago
i have similar problem - the plugin doesn't account for cursor width and contentPadding paraps and so part of a content hidden ( overflow | runs out of the view bounady ) . . . 😥
value: 123
-- the space on the right is 10px cursor ( it will look the the same if padding is provided)
@yuriescl @MisaGu May I ask was the text configured to scale in the Android settings on the physical device? Thank you!
@lzhuor If you mean accessibility scale, it's disabled and the size is at the default (middle):
The system font settings are also in default:
I also get this issue running the code on a stock Huawei P30 lite with a flutter based app...
Hi @antb123 and @yuriescl may I ask did you manage to figure out the root cause? Could you please also try auto_size_text
package and see if it works for displaying static texts?
This code makes the text overflow the widget in physical Android devices:
Screenshot of physical Android screen (Android 10) running the above code: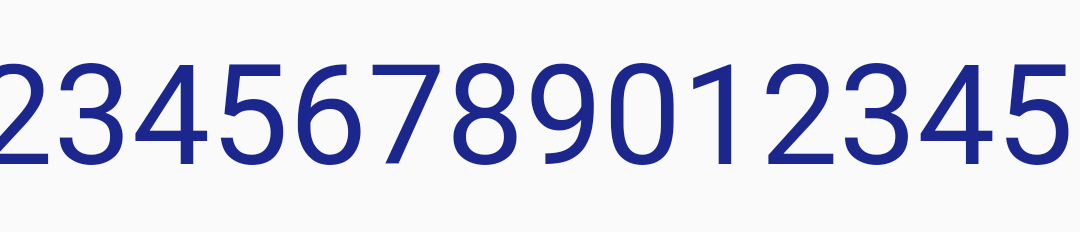
The same code works in Android Emulator (tested on Pixel 4 XL API 28), there's no overflow there:
Version tested:
auto_size_text_field: ^0.1.7