Open arpansengupta-appsbee opened 4 years ago
I guess this is related to this GPS_MIN_DISTANCE. I mean gps receiver provides coordinates not frequently and sum of noise from accelerometer becomes bigger (we accumulate this error during predict step).
Hello, I have implemented your library in a foreground service. I am using only the Kalman filter to get user's position every 10m. While setting Utils.GPS_MIN_DISTANCE to 0 the deviations in the points returned is acceptable but setting it to 10m it becomes very noisy.
Below is my locationService. I have used default values in KalmanLocationService.Settings except Utils.GPS_MIN_DISTANCE
` public class LocationMonitoringService extends Service implements LocationServiceInterface, ILogger {
} ` Below are the poly lines drawn using these position. The actual polylines should be along the road.
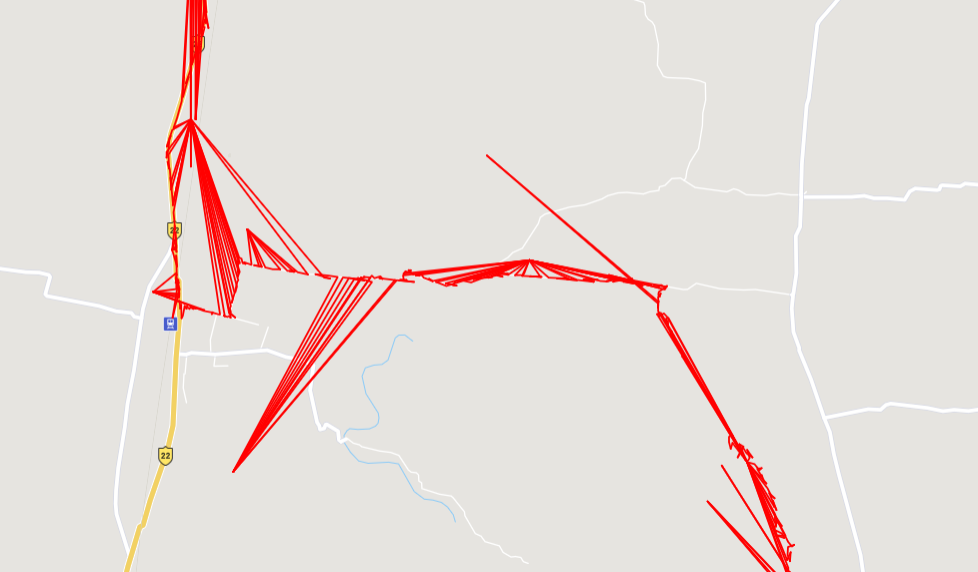