Open maicFir opened 2 years ago
在上一篇文章中我们用webpack与webpack-cli搭建了最简单的前端应用,通常在项目中我们会用vue或者react,我们看下如何利用我们自己搭的工程来适配react
webpack
webpack-cli
vue
react
正文开始...
首先我们要确定,react并不是在webpack中像插件一样安装就可以直接使用,我们需要支持jsx以及一些es6的一些比较新的语法,在creat-react-app这个脚手架中已经帮我们高度封装了react项目的一些配置,甚至你是看不到很多的配置,比如@babel/preset-react转换jsx等。所以我们需要知道一个react项目需要哪些插件的前提条件,本文主要参考从头开始打造工具链
jsx
es6
creat-react-app
@babel/preset-react
安装babel相关插件
babel
npm i @babel/core @babel/cli @babel/preset-env @babel/preset-react --save
其中babel/core就是能将代码进行转换,@babel/cli允许命令行编译文件,babel/preset-env与@babel/preset-react都是预设环境,把一些高级语法转换成es5
babel/core
@babel/cli
babel/preset-env
es5
安装好相关插件后,我们需要在根目录中创建一个.babelrc来让babel通知那两个预设的两个插件生效
.babelrc
// .babelrc { "presets": ["@babel/env", "@babel/preset-react] }
接下来我们需要安装在react中的支持的jsx,主要依赖babel-loader来编译jsx
babel-loader
npm i babel-loader --save-dev
并且我们需要改下webpack.config.js的loader
webpack.config.js
loader
{ module: { rules: [ { test: /\.css$/, use: ['style-loader', 'css-loader'] }, { test: /\.(png|svg|jpg|gif|jpeg)$/, use: [ { loader: 'file-loader', options: { outputPath: 'assets', name: '[name].[ext]?[hash]' } } ] }, { test: /\.(js|jsx)$/, loader: 'babel-loader', exclude: /node_modules/, options: { presets: ['@babel/env'] } } ] }, }
在react中我们设置HMR,我们需要结合new webpack.HotModuleReplacementPlugin(),并且在devServer中设置hot为true
HMR
new webpack.HotModuleReplacementPlugin()
devServer
hot
true
module.exports = { ... plugins: [ new HtmlWebpackPlugin({ template: './public/index.html' }), new miniCssExtractPlugin({ filename: 'css/[name].css' }), new webpack.HotModuleReplacementPlugin() ], devServer: { hot: true } }
完整的配置webpack.config.js就已经 ok 了
// webpack.config.js const path = require('path'); const webpack = require('webpack'); const HtmlWebpackPlugin = require('html-webpack-plugin'); const miniCssExtractPlugin = require('mini-css-extract-plugin'); module.exports = { mode: 'development', entry: { app: './src/app.js' }, output: { path: path.resolve(__dirname, 'dist'), filename: '[name].bundle.js' }, module: { rules: [ { test: /\.css$/, use: ['style-loader', 'css-loader'] }, { test: /\.(png|svg|jpg|gif|jpeg)$/, use: [ { loader: 'file-loader', options: { outputPath: 'assets', name: '[name].[ext]?[hash]' } } ] }, { test: /\.(js|jsx)$/, loader: 'babel-loader', options: { presets: ['@babel/env'] } } ] }, plugins: [ new HtmlWebpackPlugin({ template: './public/index.html' }), new miniCssExtractPlugin({ filename: 'css/[name].css' }), new webpack.HotModuleReplacementPlugin() ], devServer: { hot: true } };
安装react、react-dom这两个核心库
react-dom
npm i react react-dom --save-dev
在src目录下新建一个App.jsx
src
App.jsx
// App.jsx import React, { Component } from 'react'; import deepMerge from './utils/index.js'; import '../src/assets/css/app.css'; import image1 from '../src/assets/images/1.png'; import image2 from '../src/assets/images/2.jpg'; class App extends Component { constructor(props) { super(props); this.state = { text: 'hello webpack for react', name: 'Maic', age: 18, publicName: 'Web技术学苑', imgSource: [image1, image2] }; } render() { const { text, name, age, publicName, imgSource } = this.state; return ( <> <div className='app'> <h1>{text}</h1> <div> <p>{name}</p>,<span>{age}</span>岁<p>{publicName}</p> </div> <div> {imgSource.map((src) => ( <img src={src} key={src} /> ))} </div> </div> </> ); } } export default App;
我们在app.js中引入App.jsx
app.js
// app.js import React from 'react'; import { createRoot } from 'react-dom/client'; import App from './App.jsx'; const appDom = document.getElementById('app'); const root = createRoot(appDom); root.render(<App />);
我们运行npm run server,浏览器打开localhost:8080 ok,用webpack搭建的一个自己的react应用就已经 ok 了
npm run server
localhost:8080
1、react 需要的一些插件,@babel/core、@babel/cli、@babel/preset-env、@babel/preset-react、babel-loader
@babel/core
@babel/preset-env
2、设置.babelrc
3、引入react、react-dom,modules中设置babel-loader编译jsx文件
modules
4、本文code-example
正文开始...
前置
首先我们要确定,
react
并不是在webpack
中像插件一样安装就可以直接使用,我们需要支持jsx
以及一些es6
的一些比较新的语法,在creat-react-app
这个脚手架中已经帮我们高度封装了react
项目的一些配置,甚至你是看不到很多的配置,比如@babel/preset-react
转换jsx
等。所以我们需要知道一个react
项目需要哪些插件的前提条件,本文主要参考从头开始打造工具链安装
babel
相关插件其中
babel/core
就是能将代码进行转换,@babel/cli
允许命令行编译文件,babel/preset-env
与@babel/preset-react都是预设环境,把一些高级语法转换成es5
安装好相关插件后,我们需要在根目录中创建一个
.babelrc
来让babel
通知那两个预设的两个插件生效接下来我们需要安装在
react
中的支持的jsx
,主要依赖babel-loader
来编译jsx
并且我们需要改下
webpack.config.js
的loader
在
react
中我们设置HMR
,我们需要结合new webpack.HotModuleReplacementPlugin()
,并且在devServer
中设置hot
为true
完整的配置
webpack.config.js
就已经 ok 了安装
react
、react-dom
这两个核心库在
src
目录下新建一个App.jsx
我们在
app.js
中引入App.jsx
我们运行
ok,用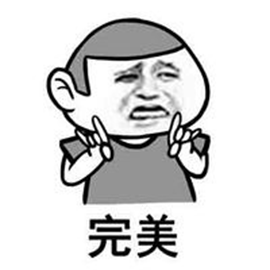
npm run server
,浏览器打开localhost:8080
webpack
搭建的一个自己的react
应用就已经 ok 了总结
1、react 需要的一些插件,
@babel/core
、@babel/cli
、@babel/preset-env
、@babel/preset-react
、babel-loader
2、设置
.babelrc
3、引入
react
、react-dom
,modules
中设置babel-loader
编译jsx
文件4、本文code-example