Closed Monracloche closed 2 years ago
Did you give the sensors the address 0x10
and 0x11
? You have to use the set address script on each sensor individually in order to configure their address.
Yes, with this instruction pzems[i] = PZEM004Tv30(PZEM_SERIAL, 0x10 + i);
they didn't change their addresses, they both kept the same address, I thought that's why it didn't work and I changed them with this other instruction pzems[i].setAddress(addr+i);
now they each have a different address but they still don't work
No, I haven't explained that well. You DON'T configure the address in your program! You have to use the PZEMChangeAddress
example sketch to configure each of the PZEMs individually! The PZEMChangeAddress
sketch will write the custom address into the EEPROM of the connected PZEM. This way even after a power off, it will remember the new address. The PZEMChangeAddress
example sketch should be available form the example folder in Arduino IDE under the PZEM-004T-v30 library.
Open this example program https://github.com/mandulaj/PZEM-004T-v30/tree/master/examples/PZEMChangeAddress
Change this #define SET_ADDRESS
to 0x10:
#if !defined(SET_ADDRESS)
#define SET_ADDRESS 0x10
#endif
Make sure #define INCREMENT
is false!
Flash the modified example program (PZEMChangeAddress
) to your microcontroller
Connect ONE of the PZEMs to the micro controller and run the sketch. This configures the address for that ONE PZEM to 0x10
Disconnect the PZEM!
Change the SET_ADDRESS
to 0x11
#if !defined(SET_ADDRESS)
#define SET_ADDRESS 0x11
#endif
Reflash it to the microcontroller
Connect the second PZEM to the microcontroller and run the sketch
The second PZEM will now have the address of 0x11
Disconnect it from the microcontroller
Flash your program (Do not include the pzems[i].setAddress(addr+i);
in your program) Just use the pzems[i] = PZEM004Tv30(PZEM_SERIAL, 0x10 + i);
when initizalizing the PZEMs
Now connect both of the PZEMs to the microcontroller and yoru sketch should work.
Basically, when you have only one PZEM connected you can use the default 0xF8
address which every PZEM will always listen to regardless of what its actually custom address is. But if there are multiple PZEMs connected to the bus, the address 0xF8
makes no sense since all PZEMs would be responding to the messages.
This is why you have to configure them individually. Once you write a separate 0x10
and 0x11
address to the two PZEMs, you can use those to differentiate which one to talk to.
The PZEMChangeAddress
example sketch does exactly this. It uses the default address 0xF8
to talk to the connected PZEM and uses pzems.setAddress(SET_ADDRESS);
to set its address to the value of SET_ADDRESS
.
You only have to do this once. Then the PZEMs will remember their custom addresses and you can talk to them using those.
I just tested what you wrote and it worked at the first time. Thank you very much for the help ... I had been trying things without success for days
Hello, first of all thank you very much for the help. I am testing the @mandulaj code for multiple sensors (2) with arduino mega and serial2 hardware but I can't operate both sensors at the same time. This is de code:
This is the wiring:
And this is the results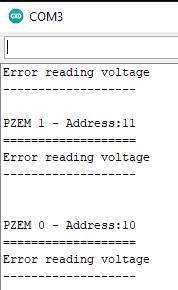
The Tx and Rx leds from the sensors are blinking and when I disconnect one of them, the one that stays connected works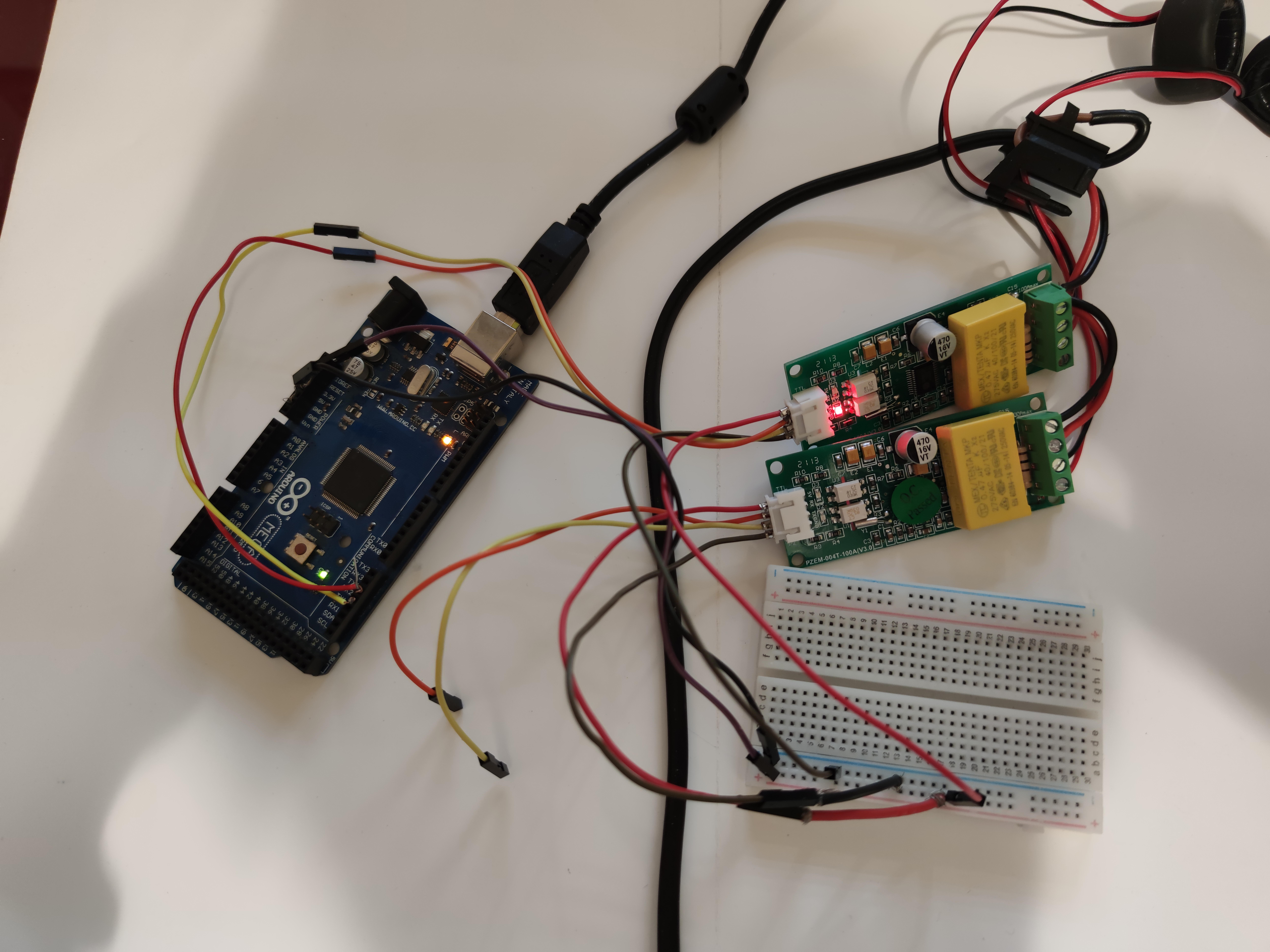
I have also tried serial software code with the same result, does anyone know what the problem is? Thank you very much again