Closed Pieter-127 closed 6 years ago
@PieterVenter77 this issue will stem from using GLSurfaceView as render surface. For this use-case you will need to use a TextureView instead. You can enable this feature by either in xml attributes:
mapbox_renderTextureMode=true
or
programatically with:
MapboxMapOptions#textureMode(true)
This should fix your use-case, let us know if it didn't, closing as answered.
Making use of the mapbox snapshotter to take a picture of the map I need, on click of the map trying to show a dialog fragment with the entire mapview. The snapshotter works perfectly fine, but the dialog i'm creating more often than not just shows the mapview entirely blacked out. Occasionally it works, although mostly it goes black.
Steps to reproduce
class MapDialogFragment : DialogFragment() {
}
Expected behavior
Map shows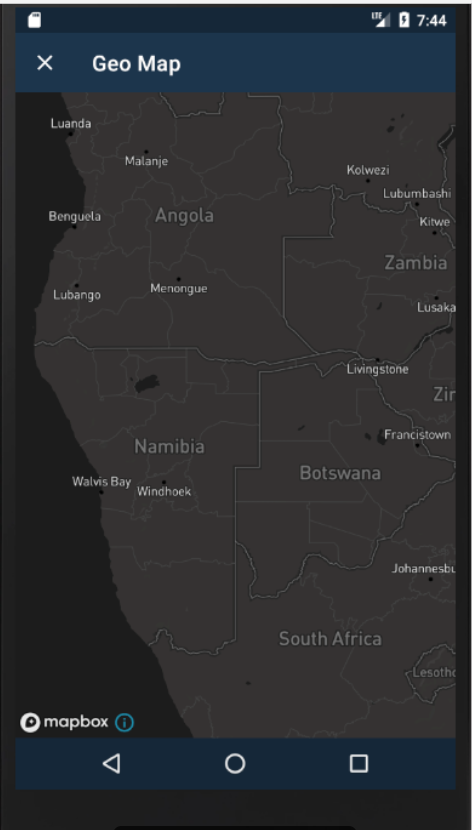
Actual behavior
Map goes entirely dark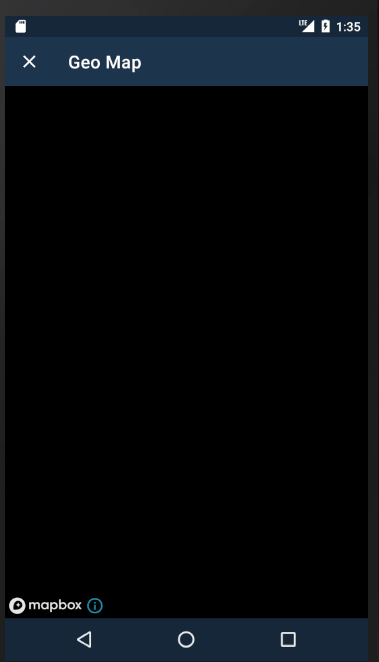
Configuration
Android versions: API 23 Device models: Pixel 2 Mapbox SDK versions: 6.2.0 - 6.5.0