Open ajoykarmakar opened 3 years ago
Same issue in:
"react": "17.0.2",
"react-native": "0.66.1"
"react-native-big-list": "^1.4.3",
Try use inside a View with flex: 1
+1 same issue
renderHeader and Footer worked but item is not
I had a case of blank list in an app that I am working on, using react-navigation, I had two bottom tab routes, one with the list of collections in Collections Screen (but not only) and the other being the Settings screen, If I entered Settings and got back to collections, the list would be there, but if I entered in another route through Settings, in this case, Accounts Screen route, and got back to Collections after, the list would be blank (only the BigList), every time.
Only fix was to set the Collections screen route to unmount on blur through react-navigation.
I follow the example from your project and documentation and got a blank screen.
Code -
Config -
Result -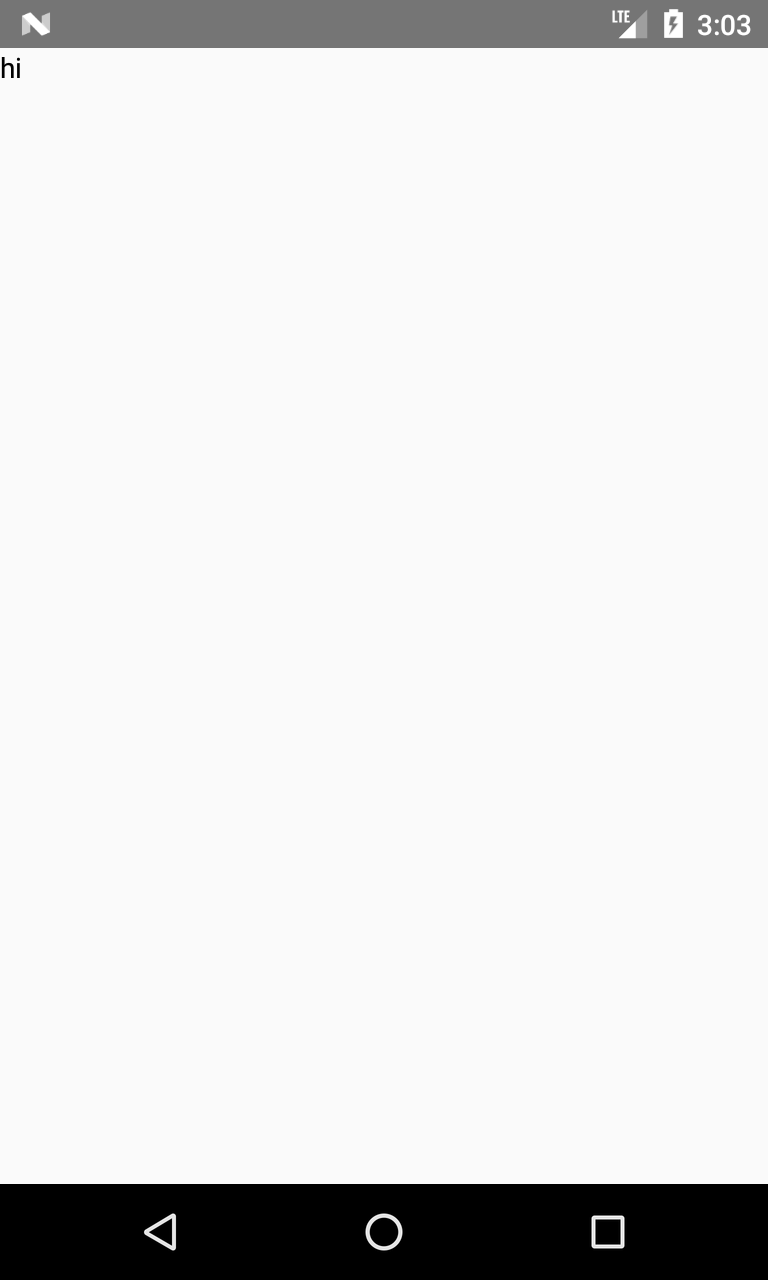