Open mate-h opened 2 years ago
When you click back, you see the video playing backwards.
function AnimatedPages({
totalPages = 4,
timecodes = [437, 580, 793, 1177],
filename = 'customization-demo-2.mp4'
}) {
const [currentPage, setCurrentPage] = useState(0);
const [prevPage, setPrevPage] = useState(0);
const [playing, setPlaying] = useState(false);
function wrap(i) {
return i % totalPages;
}
/** Returns the timecode in frames */
const lookupTimecode = (i) => timecodes[i];
function playVideo(){
// TODO: implement
// only play the video to the next keyframe
const prevKeyframe = lookupTimecode(prevPage);
const nextKeyframe = lookupTimecode(currentPage);
}
function playVideoBackwards(){
// TODO: implement
}
function pauseVideo(){
// TODO: implement
}
function skipTo(page){
// TODO: implement
}
useEffect(() => {
if (playing) {
const diff = currentPage - prevPage;
if (Math.abs(diff) > 1) {
skipTo(currentPage);
}
else if (diff > 0) {
playVideo();
} else {
playVideoBackwards();
}
} else {
pauseVideo();
}
}, [playing]);
// dir can be +1 or -1
function onClick(dir: number) {
//Is the video paused?
// 1.1 if paused, then set to playing. Change animation state (increment or decrement with wrap around)
// 1.2 if not paused, the skip to current page's keyframe. It's set to paused.
if (playing) {
setPlaying(false);
// we don't change the current page, because we want to keep the same page
// skip to the current page's keyframe
skipTo(currentPage);
} else {
setPlaying(true);
setPrevPage(currentPage);
setCurrentPage(wrap(currentPage + dir));
}
}
}
Take this video file: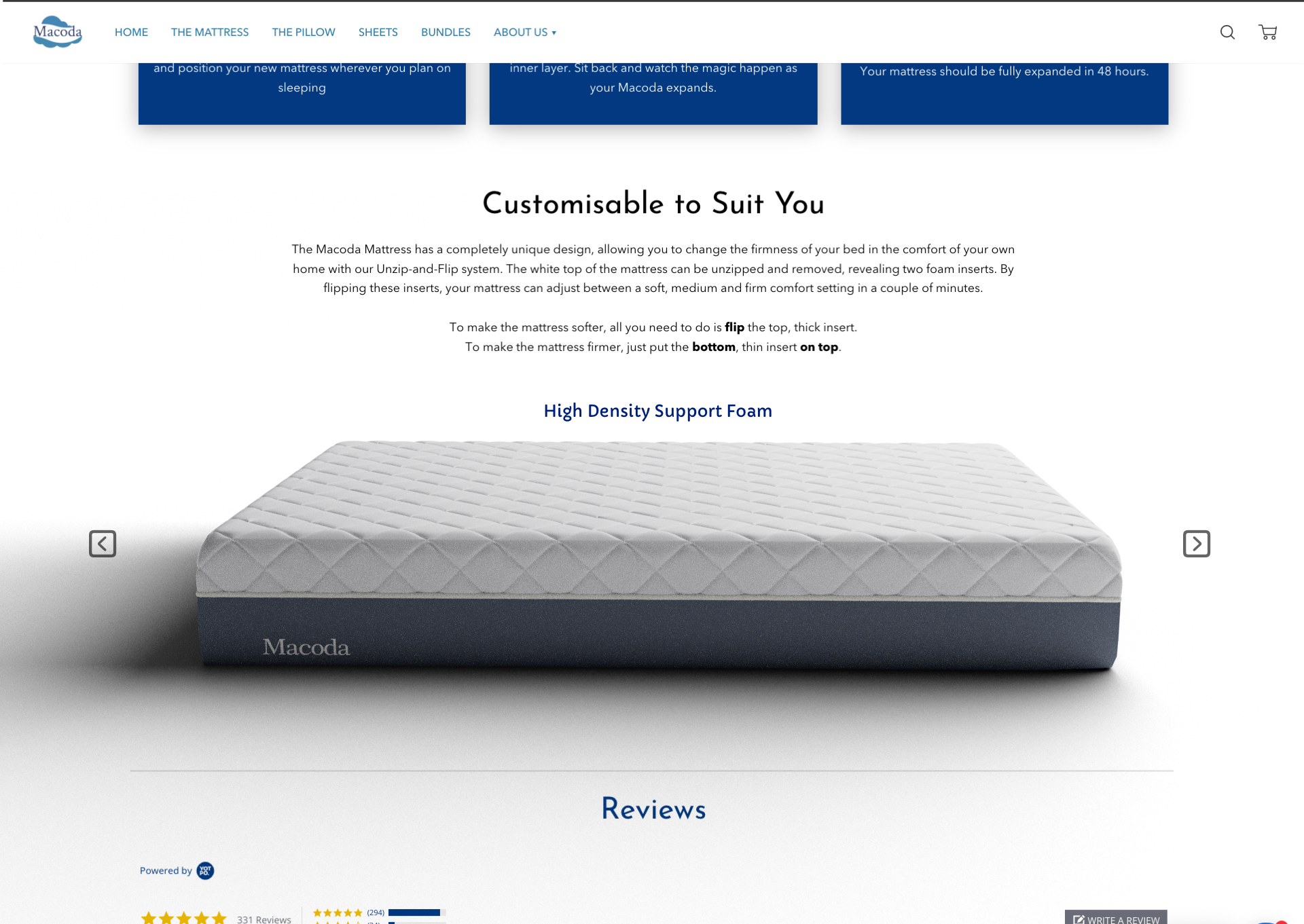
customization-demo-2.mp4
and skip to the appropriate times as the user skips the buttons.customization-demo-2.mp4
has the following keyframes:By default, the video is paused. As user clicks the right arrow, the page is incremented, and the video is seeked to the next keyframe. If the current page is the last, wrap around (start from beginning). Same with the first page, skip to last.
Video state: playing/paused. Animation state: current page (1-4), previous page
If click happens:
(Animation time could be constant between pages, let's say
1s
, may not look good)