Closed 4rzael closed 5 years ago
First, undefine LMIC_USE_INTERRUPTS. That is not a tested configuration in this library. (It came from the original library, but it's not supported, and wasn't supported there, either; I just haven't gotten around to taking it out). Then see if things become more sensible.
However, it's very strange that you would get something that decrypts correctly -- I've never seen that, but it suggests that the RAM buffer for the message in LMIC is getting overwritten. The APPEUI you show looks suspiciously like pointers.... check your link map and make sure everything is sane. (Trying to use SPI interrupts in this code could cause any kind of strange problem, so it still could be LMIC_USE_INTERRUPTS.)
Hello, I also tried without LMIC_USE_INTERRUPTS, and I am having the same results. And thanks for the hint on pointers, I didn't think of this, I'm going to check what is going on.
Ok, I found out the issue:
I copied the function GetOtaaProvisioningInfo
from simple.ion, which seems to be incorrect (it fills a data structure that is never returned and then destroyed at the end of the function.
When changing it to this:
bool cMyLoRaWAN::GetOtaaProvisioningInfo(
OtaaProvisioningInfo *pInfo)
{
static const uint8_t APPKEY[16] = { 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07, 0x08, 0x09, 0x0A, 0x0B, 0x0C, 0x0D, 0x0E, 0x0F };
static const uint8_t DEVEUI[8] = { 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07 };
static const uint8_t APPEUI[8] = { 0x00, 0x01, 0x02, 0x03, 0x04, 0x05, 0x06, 0x07 };
if (pInfo) {
memcpy(pInfo->AppKey, APPKEY, sizeof(APPKEY));
memcpy(pInfo->DevEUI, DEVEUI, sizeof(DEVEUI));
memcpy(pInfo->AppEUI, APPEUI, sizeof(APPEUI));
}
return true;
}
and making sure that DEVEUI and APPEUI are written in LSB first mode while APPKEY is MSB first, I am able to authenticate to TTN and send messages. Thanks for the help !
Could you please share your pin mapping for the SPI? MOSI, MISO and SCLK I am trying to connect sx1276 with teensy 3.6. Thanks in advance
Hello, I am trying to use your library to make a teensy 3.6 using the arduino framework communicate to TTN through an RFM95W chip.
I've setup a platformio project, configured the compile flags (
-DCFG_eu868=1 -DARDUINO_LMIC_PROJECT_CONFIG_H_SUPPRESS=1 -DCFG_sx1276_radio=1 -DLMIC_USE_INTERRUPTS=1
).I also setup a LoraWAN gateway at around 1m of the sensor.
When the sensor starts, it transmits the following events:
Using a software-defined radio, I can see that my sensor seems to succeed in sending the packets at the correct frequency: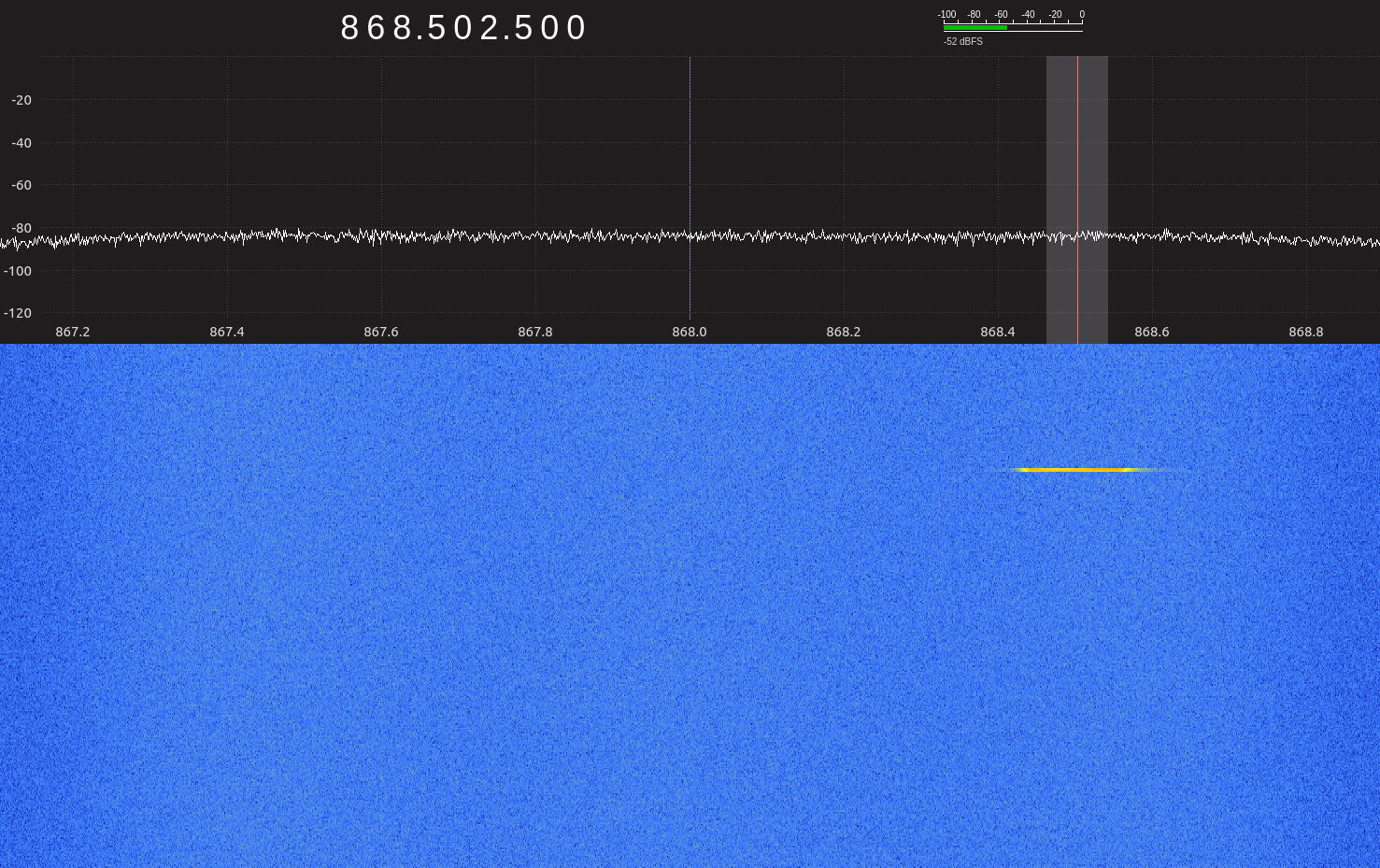
However, my gateway either doesn't receive the packets, or receives something with wrong EUIs (ex: APP_EUI=1FFF19001FFF18EC and DEV_EUI=00000000000542A7).
Do you have any idea why I get this result ? How can I have more insights on what's going wrong ?
This is a simplified version of my code: (taken from simple.ino)