repeat : Int -> String -> String
repeat n chunk =
repeatHelp n chunk ""
repeatHelp : Int -> String -> String -> String
repeatHelp n chunk result =
if n <= 0 then
result
else
repeatHelp (Bitwise.shiftRightBy 1 n) (chunk ++ chunk) <|
if Bitwise.and n 1 == 0 then
result
else
result ++ chunk
Replacement
The replacement is equivalent to the following Elm code:
repeat : Int -> String -> String
repeat n chunk =
repeatHelp n chunk ""
repeatHelp : Int -> String -> String -> String
repeatHelp n chunk result =
if n <= 0 then
result
else
repeatHelp (Bitwise.shiftRightBy 1 n)
(chunk ++ chunk ++ "")
(if Bitwise.and n 1 == 0 then
result
else
result ++ chunk ++ ""
)
The main improvements are:
the function is made tailcall recursive (by removing the <|)
++ "" has been added when concatenating strings, forcing the compiler to use + instead of the slower _Utils_ap. This was the biggest gain in performance.
For this PR, I have slightly altered the replacement to
not have a repeatHelp function (its replacement is empty to remove it) by inlining repeatHelp inside repeat
not have the unnecessary + '' (that could be removed by the compiler IMO)
remove the unnecessary temporary variables
These don't seem to have a noticeable effect as far as I can tell, but it reduces the bundle size ever so slightly maybe. Let me know if you want me to use the "original version".
String replacements seem to be disabled in all configurations for the tool and I'm not sure why. That also needs to change otherwise this replacement will not have any effect.
This is a replacement for
String.repeat
Original
Replacement
The replacement is equivalent to the following Elm code:
The main improvements are:
<|
)++ ""
has been added when concatenating strings, forcing the compiler to use+
instead of the slower_Utils_ap
. This was the biggest gain in performance.For this PR, I have slightly altered the replacement to
repeatHelp
function (its replacement is empty to remove it) by inliningrepeatHelp
insiderepeat
+ ''
(that could be removed by the compiler IMO)These don't seem to have a noticeable effect as far as I can tell, but it reduces the bundle size ever so slightly maybe. Let me know if you want me to use the "original version".
Results
Benchmark is run with
elm-optimize-level-2
.Chrome: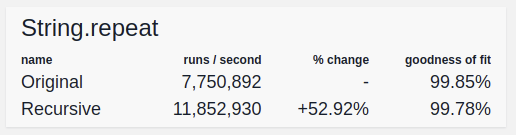
Firefox: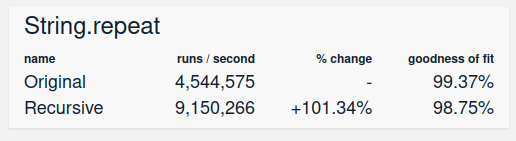
Notes
String replacements seem to be disabled in all configurations for the tool and I'm not sure why. That also needs to change otherwise this replacement will not have any effect.