Closed juhaku closed 1 year ago
Thanks for the detailed report but this is not a client issue but a problem with the language server, or in this case probably even with eclipse.jdt.core. You'll have to report this upstream
Yeah, this seems to have been an issue for a long time in eclipse jdt language server based on these threads: https://github.com/redhat-developer/vscode-java/issues/2324 https://github.com/eclipse/eclipse.jdt.ls/issues/2237 :facepalm: Just when I thought I was able to rely on neovim to write some Java, but it seems that I have to fall back to IntelliJ.
LSP client configuration
Eclipse.jdt.ls version
1.19.0 / 1.20.0
Steps to Reproduce
Open a code base and try to apply completion within a lambda body as seen in the picture. The completion does not show up in cases where the lambda body is an method argument which expects a lambda body. This seems to be an issue with lambdas that are returning something. Consumers does seem to work since they will match the method argument signature immediately. But the Suppliers break for some reason. Perhaps because they do not match to the method signature.
If the lambda was to be extracted from the method argument to a separate method or a local variable, the auto completion starts working again as normally.
Expected Result
The completion should open as in the picture:
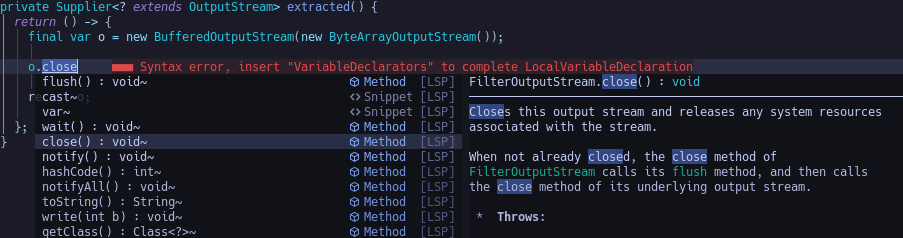
Actual Result
Completion does not open: